在Unity 3D游戏引擎中,可以使用不同的方式对物体进行旋转。以下是几种常见的旋转方式:
-
欧拉角(Euler Angles):欧拉角是一种常用的旋转表示方法,通过绕物体的 X、Y 和 Z 轴的旋转角度来描述物体的旋转。在Unity中,可以通过修改物体的 transform.eulerAngles 属性来进行欧拉角旋转。
-
四元数(Quaternion):四元数是另一种常见的旋转表示方法,在Unity中经常用于插值和平滑旋转。使用四元数可以避免万向锁等问题,并提供更高效和准确的旋转计算,可以通过修改物体的 transform.rotation 属性来进行四元数旋转。
-
轴角(Axis-Angle):轴角表示法使用一个单位向量来定义旋转轴,并使用旋转角度来描述旋转。在Unity中,可以使用 Quaternion.AngleAxis() 函数来创建一个轴角旋转,并通过修改物体的 transform.rotation 属性进行应用。
-
矩阵(Matrix):矩阵表示法通过使用旋转矩阵来描述旋转变换。在Unity中,可以通过修改物体的 transform.localRotation 属性或 transform.rotation 属性中的矩阵元素来进行矩阵旋转。
我们接下来实现下面2种方法来实现人物的旋转和移动
一:使用WADS进行移动旋转
代码实现:
using System; using System.Collections; using System.Collections.Generic; using UnityEditor; using UnityEngine;public class PlayerMove : MonoBehaviour {// Start is called before the first frame update//设置速度public float speed = 6f;Rigidbody rig;//动画Animator anim;//偏移量Vector3 moveMent;//跳起来的力public float Jump_AddForce = 300f;void Awake(){//获取刚体rig = GetComponent<Rigidbody>();//获取动画anim = GetComponent<Animator>();}// Update is called once per framevoid FixedUpdate(){// -1 1float h = Input.GetAxisRaw("Horizontal");float v = Input.GetAxisRaw("Vertical");//移动 横向和纵向Move(h, v);}void Move(float h, float v){//设置方向moveMent.Set(h, 0f, v);moveMent = moveMent.normalized * speed * Time.deltaTime;//通过刚体主键移动 对象rig.MovePosition(transform.position + moveMent);//移动动画bool isWalking = (h != 0 || v != 0);anim.SetBool("Run", isWalking);//旋转Vector3 dir = new Vector3(h, 0, v);if (dir != Vector3.zero){transform.rotation = Quaternion.LookRotation(dir);transform.Translate(Vector3.forward * speed * Time.deltaTime);}}
二:使用鼠标来控制人物的旋转,WASD移动
代码实现:
using System; using System.Collections; using System.Collections.Generic; using UnityEditor; using UnityEngine;public class PlayerMove : MonoBehaviour {// Start is called before the first frame update//设置速度public float Speed = 6f;Rigidbody RigidbodyPlayer;Animator animatorPlayer;//偏移量Vector3 moveMent;//地板LayerMask floorMask;//Vector3 playerToMouse;void Awake(){//获取刚体RigidbodyPlayer = GetComponent<Rigidbody>();//获取动画animatorPlayer = GetComponent<Animator>();//获取地板floorMask = LayerMask.GetMask("floor");}// Update is called once per framevoid FixedUpdate(){// -1 1float h = Input.GetAxisRaw("Horizontal");float v = Input.GetAxisRaw("Vertical");//移动 横向和纵向Move(h, v);//检测动画Animating(h, v);//角色旋转Turning();}void Move(float h, float v){//设置方向moveMent.Set(h, 0f, v);moveMent = moveMent.normalized * Speed * Time.deltaTime;//通过刚体主键移动 对象RigidbodyPlayer.MovePosition(transform.position + moveMent);}//移动动画void Animating(float h, float v){if (h != 0 || v != 0){animatorPlayer.SetBool("IsWaking", true);}else{animatorPlayer.SetBool("IsWaking", false);}}void Turning(){Ray cameraRay = Camera.main.ScreenPointToRay(Input.mousePosition);RaycastHit cameraHit;if (Physics.Raycast(cameraRay, out cameraHit, 100f, floorMask)){Vector3 playerToMouse = cameraHit.point - transform.position;playerToMouse.y = 0f;//旋转 四元素Quaternion newQraternion = Quaternion.LookRotation(playerToMouse);//角色刚体旋转RigidbodyPlayer.MoveRotation(newQraternion);}} }
最后
以上步骤就是实现角色的移动旋转。希望能对你们提供帮助!!!
看到的小伙伴一键三连一下吧,你们的支持让我更有动力去创作和分享,希望能一直为你们带来惊喜和收获。
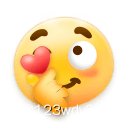