要求如下
[[{"oone": "评估是否聘请第三方机构","otwo": null,"othree": "test",},{"oone": "评估是否聘请第三方机构","otwo": null,"othree": "test",}],[{"oone": "评估是否聘请第三方机构","otwo": null,"othree": "test",},{"oone": "评估是否聘请第三方机构","otwo": null,"othree": "test",}]
]<!-- 转换成 -->
[{"oone": "评估是否聘请第三方机构","otwo": null,"othree_1": "test","othree_2": "test"},{"oone": "评估是否聘请第三方机构","otwo": null,"othree_1": "test","othree_2": "test"}
]
代码实战
function transformData(data) {const numObjectsInResult = data[0].length;const result = Array.from({ length: numObjectsInResult }, (_, objIndex) => {const firstObjectOfSubArray = data[0][objIndex];return { ...firstObjectOfSubArray };
});data.forEach((subArray, subArrayIndex) => {subArray.forEach((obj, objIndex) => {result[objIndex][`othree_${subArrayIndex + 1}`] = obj.othree;});});return result;
}const originalData = [[{"oone": "评估是否聘请第三方机构", "otwo": null, "othree": "test"},{"oone": "评估是否聘请第三方机构", "otwo": null, "othree": "test"},{"oone": "评估是否聘请第三方机构", "otwo": null, "othree": "test"}],[{"oone": "评估是否聘请第三方机构", "otwo": null, "othree": "test"},{"oone": "评估是否聘请第三方机构", "otwo": null, "othree": "test"},{"oone": "评估是否聘请第三方机构", "otwo": null, "othree": "test"}],[{"oone": "评估是否聘请第三方机构", "otwo": null, "othree": "test"},{"oone": "评估是否聘请第三方机构", "otwo": null, "othree": "test"},{"oone": "评估是否聘请第三方机构", "otwo": null, "othree": "test"}],[{"oone": "评估是否聘请第三方机构", "otwo": null, "othree": "test"},{"oone": "评估是否聘请第三方机构", "otwo": null, "othree": "test"},{"oone": "评估是否聘请第三方机构", "otwo": null, "othree": "test"}],[{"oone": "评估是否聘请第三方机构", "otwo": null, "othree": "test"},{"oone": "评估是否聘请第三方机构", "otwo": null, "othree": "test"},{"oone": "评估是否聘请第三方机构", "otwo": null, "othree": "test"}]
];const result = transformData(originalData);
console.log(result);
算法优化
- 减少数组遍历次数
- 避免不必要的对象复制
- 使用Map或对象作为临时存储
function transformData(data) {const numObjectsInResult = data[0].length;const result = new Map();data[0].forEach((obj, index) => {result.set(index, { ...obj });for (let i = 1; i <= data.length; i++) {result.get(index)[`othree_${i}`] = null;}});data.forEach((subArray, subArrayIndex) => {subArray.forEach((obj, objIndex) => {result.get(objIndex)[`othree_${subArrayIndex + 1}`] = obj.othree;});});return Array.from(result.values());
}
应用场景
- 在vue中前面几个列可能是固定的, 后边几个列是动态展示
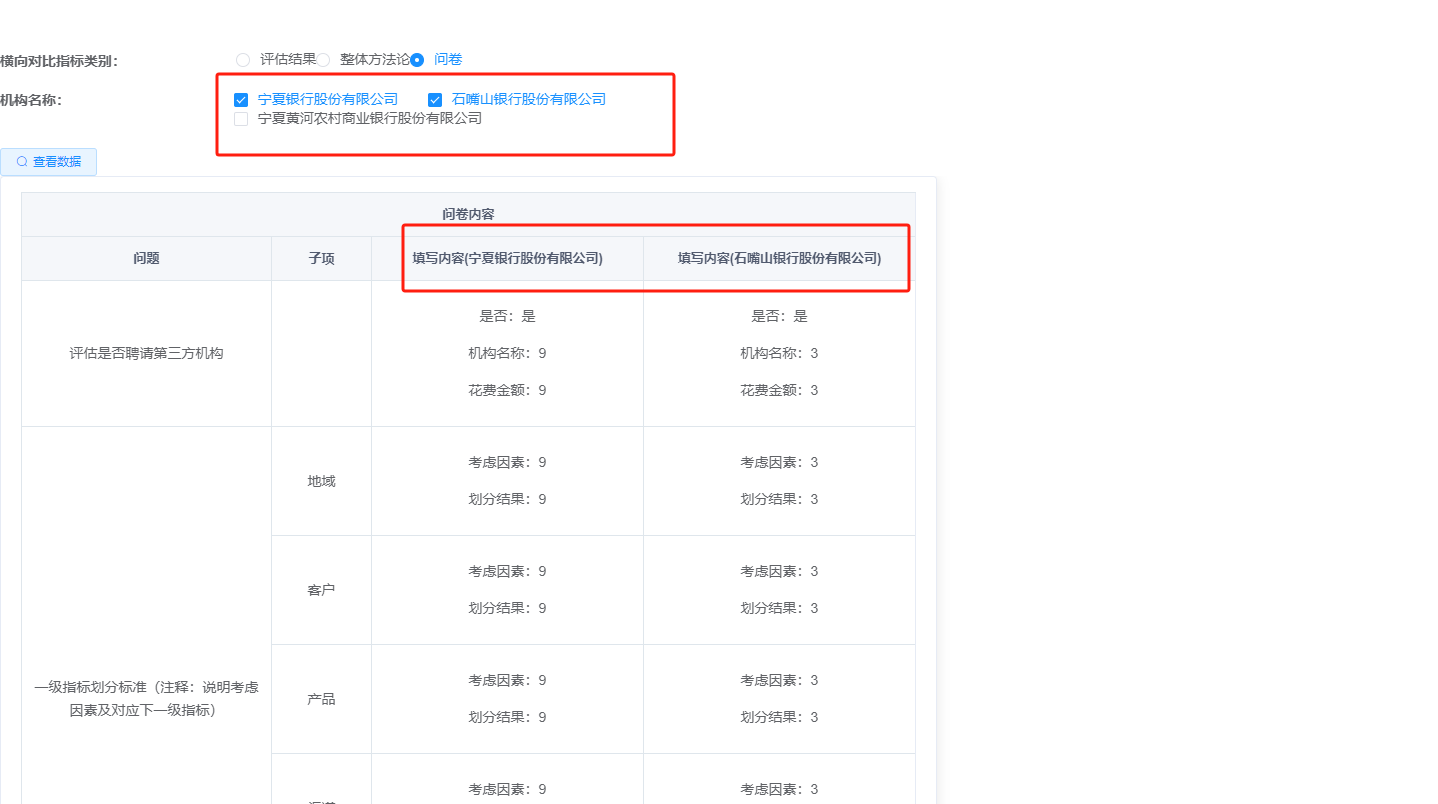
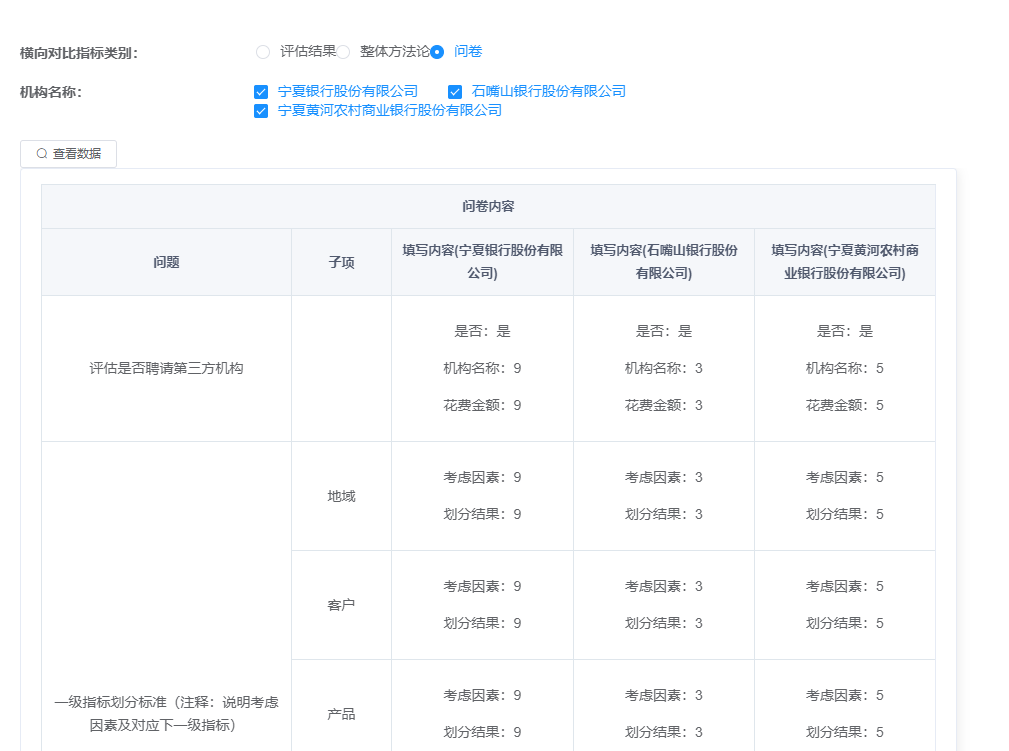
<template v-if="questionList.length > 0"><el-col class="third-el-col" :style="{width: questionlenght}"><el-card><el-table:data="questionListFix":span-method="objectSpanMethodThirdFix"><el-table-column label="问卷内容" rowspan="2" align="center"><el-table-columnprop="oone"label="问题"align="center"width="250px"></el-table-column><el-table-columnprop="otwo"label="子项"align="center"width="100px"></el-table-column><el-table-columnv-for="(column, index) in dynamicColumns":key="index":prop="column.prop":label="column.label"align="center"><template v-slot="scope"><MathInput:disabled="true":isTable="true"v-model="scope.row[column.prop]"></MathInput></template></el-table-column></el-table-column></el-table></el-card></el-col></template>this.dynamicColumns.splice(0);let counter = 1;for (let i = 0; i < this.seachInfoIdList.length; i++) {this.dynamicColumns.push({prop: `othree_${counter++}`,label: `填写内容(${this.taskInfoIdMap.get(this.seachInfoIdList[i])})`});}
解释
- dynamicColumns 是动态拼接的列