1、添加依赖
implementation 'net.gotev:uploadservice:4.8.0'
implementation 'net.gotev:uploadservice-okhttp:4.8.0'
2、在application中初始化服务:
//初始化上传服务private fun initUploadService() {// 文件上传createNotificationChannel()//notificationChannelID为自定义channelIdUploadServiceConfig.initialize(context = this,defaultNotificationChannel = notificationChannelID,debug = GlobalLocalStore.IS_DEBUG)UploadServiceConfig.httpStack = OkHttpStack(OkHelper.fileUploadHttpClient())UploadServiceConfig.threadPool = ThreadPoolExecutor(1, // Initial pool size5, // Max pool size5.toLong(), // Keep Alive TimeTimeUnit.SECONDS,LinkedBlockingQueue<Runnable>())UploadServiceConfig.notificationConfigFactory = { _, _ ->val title = "视频上传"UploadNotificationConfig(notificationChannelId = UploadServiceConfig.defaultNotificationChannel!!,isRingToneEnabled = true,progress = UploadNotificationStatusConfig(title = title,message = "上传中 ${Placeholder.UploadRate} (${Placeholder.Progress})",),success = UploadNotificationStatusConfig(title = title,message = "视频上传成功,耗时:${Placeholder.ElapsedTime}"),error = UploadNotificationStatusConfig(title = title,message = "视频上传失败"),cancelled = UploadNotificationStatusConfig(title = title,message = "已取消上传视频"))}GlobalRequestObserver(this, GlobalUploadObserver())}private fun createNotificationChannel() {if (Build.VERSION.SDK_INT >= 26) {val channel = NotificationChannel(notificationChannelID,"视频上传",NotificationManager.IMPORTANCE_LOW)val manager = getSystemService(Context.NOTIFICATION_SERVICE) as NotificationManagermanager.createNotificationChannel(channel)}}
3、视频上传
MultipartUploadRequest(this, serverUrl = "${BuildConfig.HTTP_BASE_URL}$UPLOAD_FILE").setMethod("POST").addHeader("Authorization", GlobalLocalStore.token).addFileToUpload(filePath = filePath,parameterName = "file",contentType = "application/octet-stream").startUpload()
4、视频上传结果处理
class GlobalUploadObserver : RequestObserverDelegate {override fun onProgress(context: Context, uploadInfo: UploadInfo){}override fun onSuccess(context: Context,uploadInfo: UploadInfo,serverResponse: ServerResponse) {if (serverResponse.code == 200 && !TextUtils.isEmpty(serverResponse.bodyString)) {// 视频上传成功val fileResponse = GsonUtils.fromJson(serverResponse.bodyString, UploadFileResponse::class.java)}Logger.e("video Success: $serverResponse")}override fun onError(context: Context, uploadInfo: UploadInfo, exception: Throwable) {when (exception) {is UserCancelledUploadException -> {Logger.e("Error, user cancelled upload: $uploadInfo")}is UploadError -> {Logger.e("Error, upload error: ${exception.serverResponse}")}else -> {Logger.e("Error: $uploadInfo", exception)}}}override fun onCompleted(context: Context, uploadInfo: UploadInfo) {Logger.e("Completed: $uploadInfo")}override fun onCompletedWhileNotObserving() {Logger.e("Completed while not observing")}
}
5、视频上传效果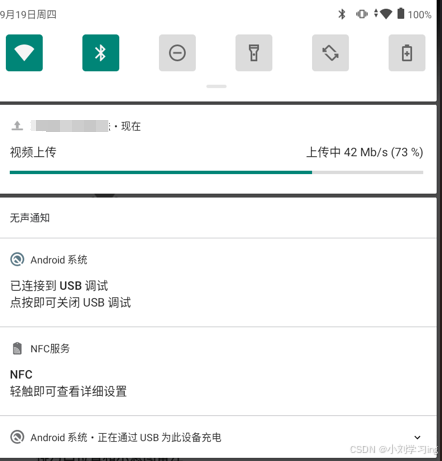
6、通知视频上成功
使用Eventbus进行实现
implementation 'org.greenrobot:eventbus:3.1.1'
①发送数据: LiveEventBus.get(context.getString(R.string.bus_key_video_upload_success)).post(newInfo)
其中newinfo为需要通知的数据
②接收数据
LiveEventBus.get(
getString(R.string.bus_key_video_upload_success),
OnUploadVideoFileInfo::class.java
).observe(this) {}