目录
IO流
一 字节流
1 FileOutStream
1 书写:
2 换行书写与续写:
2 FileInputStream
1 读取数据
2 循环读取:
二 字符流
1 FileReader
1 空参的read()方法读取数据:
2 有参的read()方法读取数据:
3 指定字符编码读取
2 FileWriter
3 补充:字符输入流底层-缓冲区:
4 补充:字符输出流底层-缓冲区
三.字节缓冲流-对基本流进行了包装
四 字符缓冲流-对基本流进行了包装
五 应用
1 文件夹的拷贝:
2 文件加密:
3 读取文件修改内容:
4 拷贝文件夹(四种方式)
5 修改文本顺序
6 软件运行次数
7 简单应用
六 转换流
应用1:指定字符编码读取数据
应用2:使用指定字符编码进行写入
应用3 :将文本的编码方式更改写入另一个文本
阶段性总结
七 序列化流
八 反序列化流
编辑1
2
3
九 打印流
1 字节打印流
2 字符打印流
十 解压缩流 /压缩流
使用idea解压文件:
使用idea压缩文件:
十一 Commons-io/Hutool
IO流
File:
- 表示系统中文件或者文件的路径,只能对文件本身进行操作,不能读写文件里面的内容。
IO流
- 存储和读取数据的解决方案,用于读写文件中的数据(可以读写文件中的数据或者网络中的数据)
流的分类:
1. 什么是IO流?
- IO流是指计算机系统中用来存储和读取数据的一种解决方案。
- I代表Input(输入),O代表Output(输出)。
- 流(Stream)就像水流一样传输数据,它是一系列连续的数据单元。
2. IO流的作用?
- IO流主要用于读写数据,无论是本地文件还是网络上的数据。
3. IO流按照流向可以分为哪两种流?
- 输出流(OutputStream):从程序到文件的方向流动,即将数据从程序发送到外部设备(如磁盘文件)。
- 输入流(InputStream):从文件到程序的方向流动,即将数据从外部设备(如磁盘文件)读取到程序中。
4. IO流按照操作文件的类型可以分为哪两种流?
- 字节流(Byte Stream):可以操作所有类型的文件,因为它们是以二进制形式处理数据的。
- 字符流(Character Stream):只能操作纯文本文件,因为它们是以字符编码的形式处理数据的。
5. 什么是纯文本文件?
- 纯文本文件是可以用Windows系统自带的记事本打开并且能够读懂的文件。这类文件只包含文本信息,没有特殊的格式或排版指令。
IO的体系结构:
一 字节流
1 FileOutStream
操作本地文件的字节输出流,可以把程序中的数据写到本地文件中。
1 书写:
代码实现:
import java.io.FileOutputStream;
import java.io.IOException;public class shu15_9 {public static void main(String[] args) throws IOException {// 创建对象->创建对象时参数是路径或者FIle都是可以的,// 如果文件不存在那么将会创建一个新的文件,但是要保证父级路径要存在FileOutputStream fos = new FileOutputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\shu15\\a.txt");// 写入数据->文件存在会覆盖原有的内容(以读取为算)// write方法写入的是整数,但是实际上写到本地文件中的是整数在ASCLL码上对应的字符//一次写一个byte num =99;fos.write(num);//一次写多个byte[] buf = {97, 98, 99,100,101,102,103,104,105};fos.write(buf);//多个数据的部分截取,第一个参数是首索引位置,第二个位置是元素个数fos.write(buf,2,4);// 释放资源->可以解除资源的占用如果不写文件会被程序占用fos.close();}
}
2 换行书写与续写:
import java.io.FileOutputStream;
import java.io.IOException;public class shu15_10 {public static void main(String[] args) throws IOException {FileOutputStream fos = new FileOutputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\shu15\\a.txt");String str = "anxainhaoshuai";//getBytes的返回值是字节数组fos.write(str.getBytes());//换行书写String str2 = "anxiannihao";String str3 = "\r\n";fos.write(str3.getBytes());fos.write(str2.getBytes());//释放资源fos.close();//续写需要讲构造方法的第二个参数改为trueFileOutputStream fos2 = new FileOutputStream("shu15\\a.txt",true);}
}
2 FileInputStream
1 读取数据
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;public class shu15_11 {public static void main(String[] args) throws IOException {//创建对象FileInputStream fis = new FileInputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\shu15\\a.txt");//读取数据int read = fis.read();System.out.println(read);//释放资源fis.close();//文件不存在直接报错,一次只能读取一个字节,读出来的是ASCLL码值(可以使用char转换)读到文件末尾方法返回-1//输出流不存在会创建(父级路径存在)//输入流不存在会报错}
}
2 循环读取:
import java.io.FileInputStream;
import java.io.IOException;public class shu15_12 {public static void main(String[] args) throws IOException {FileInputStream fis = new FileInputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\shu15\\a.txt");int b;while (((b = fis.read()) != -1)) {System.out.print((char)b);}fis.close();}
}
import java.io.FileInputStream;
import java.io.IOException;public class shu15_13 {public static void main(String[] args) throws IOException {FileInputStream fis = new FileInputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\shu15\\shu15_7.java");int len;byte[] buf = new byte[1024];while((len = fis.read(buf))!=-1){System.out.write(buf,0,len);}fis.close();}
}
读取多个数据:如果放在字节数组当中,其是遵循覆盖原则
代码实现:
import java.io.FileInputStream;
import java.io.IOException;public class shu15_12 {public static void main(String[] args) throws IOException {FileInputStream fis = new FileInputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\shu15\\a.txt");byte[] arr = new byte[2];int read = fis.read(arr);System.out.println(read);System.out.println(new String(arr, 0, read));int read2 = fis.read(arr);System.out.println(read2);System.out.println(new String(arr, 0, read2));int read3 = fis.read(arr);System.out.println(read3);System.out.println(new String(arr, 0, read3));fis.close();}
}
执行结果:
异常使用try-catch
import java.io.*;public class shu15_14 {public static void main(String[] args) {try (FileInputStream f1 = new FileInputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\shu15\\a.txt"); FileOutputStream f2 = new FileOutputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\shu15\\b.txt")) {int len;byte[] arr = new byte[1024];while ((len = f1.read(arr)) != -1) {f2.write(arr, 0, len);}} catch (IOException e) {String message = e.getMessage();System.out.println(message);}}
}
补充
二 字符流
1 特点:
- 输入流:一次读取一个字节遇到中文时,一次读取多个字节。
- 输出流:底层会把数据按照指定的编码方式进行编码,变成字节再写到文件中。
1 FileReader
1 空参的read()方法读取数据:
import java.io.FileReader;
import java.io.IOException;public class shu15_16 {public static void main(String[] args) throws IOException {//创建对象并关联本地文件FileReader fr = new FileReader("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\shu15\\shu15_15.java");int len;//空参的read方法//默认也是一个字节一个字节的读取,但是遇到中文会一次读取多个//读取之后还会转为十进制,最终返回while ((len = fr.read()) != -1) {System.out.print((char) len);}fr.close();}
}
2 有参的read()方法读取数据:
import java.io.FileReader;
import java.io.IOException;public class shu15_17 {public static void main(String[] args) throws IOException {//创建对象FileReader fr = new FileReader("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\shu15\\shu15_16.java");char[] arr = new char[3];int len;//有参的read//如果读取到汉字会读取多个字节,并进行解码并转成十进制,最终还会转为对应的字符while ((len = fr.read(arr)) != -1) {System.out.print(new String(arr, 0, len));}fr.close();}
}
3 指定字符编码读取
import java.io.*;
import java.nio.charset.Charset;public class shu15_32 {public static void main(String[] args) throws IOException {FileReader fileReader = new FileReader("C:\\\\Users\\\\ABC\\\\IdeaProjects\\\\My-projects\\\\myfirstmodule\\\\src\\\\itheima\\\\b.txt", Charset.forName("GBK"));int len;while ((len = fileReader.read()) != -1) {System.out.print((char) len);}fileReader.close();}
}
2 FileWriter
写入数据:
import java.io.FileWriter;
import java.io.IOException;public class shu15_18 {public static void main(String[] args) throws IOException {//单独的写数据FileWriter fr = new FileWriter("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\shu15\\a.txt",true);//传入一个整数fr.write(25105);//传入一个字符串fr.write("Hello World ");fr.write("Hello World ",1,5);//传入一个字符数组char[] arr = {'安','贤','好','帅','6','6','6','6'};fr.write(arr);fr.write(arr,0,3);//释放资源fr.close();}
}
3 补充:字符输入流底层-缓冲区:
会有一个8192字节的缓冲区,将需要读取的数据尽可能多的放到缓冲区中,每次就直接冲缓冲区中读取数据,缓冲区读完了从文件中读取,文件中读完了返回-1,退出读取。
【Java】`字符输入流` 的底层原理超详解_java 字节输入流原理-CSDN博客
4 补充:字符输出流底层-缓冲区
会有一个8192的缓冲区,每次写入先写到缓冲区当中,如果满了将这8192字节的数据填入本地文件中,flush方法可以将已经放到缓冲区的内容放到本地文件中,close直接结束输出流的写入,将缓冲区的内容放到本地文件中
【Java】`字符输出流` 的底层原理超详解_java的输出流是从缓冲区写到磁盘中了吗-CSDN博客
三.字节缓冲流-对基本流进行了包装
实现文件拷贝
代码实现:
import java.io.*;public class shu15_25 {public static void main(String[] args) throws IOException {BufferedInputStream bis = new BufferedInputStream(new FileInputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\shu15\\b.txt"));BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\shu15\\f.txt"));int len;while ((len = bis.read()) != -1) {bos.write(len);}bis.close();bos.close();}
}
运行结果:
四 字符缓冲流-对基本流进行了包装
特有方法:
代码实现:
import java.io.*;public class shu15_26 {public static void main(String[] args) throws IOException {//创建对象BufferedReader br = new BufferedReader(new FileReader("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\shu15\\b.txt"));BufferedWriter bw = new BufferedWriter(new FileWriter("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\shu15\\g.txt"));String s;while ((s = br.readLine()) != null) {bw.write(s);bw.newLine();}br.close();bw.close();}}
缓冲流总结
五 应用
1 文件夹的拷贝:
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;public class shu15_20 {public static void main(String[] args) throws IOException {File f1 = new File("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\ax");File f2 = new File("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\AX_1");f2.mkdir();copy_File(f1, f2);}private static void copy_File(File f1, File f2) throws IOException {for (File f : f1.listFiles()) {if (f.isFile()) {File newFile = new File(f2.getPath() + "\\" + f.getName());newFile.createNewFile();FileInputStream fis = new FileInputStream(f);FileOutputStream fos = new FileOutputStream(newFile);int len;byte[] arr = new byte[1024];while ((len = fis.read(arr)) != -1) {fos.write(arr, 0, len);}fos.close();fis.close();} else {File newFile = new File(f2.getPath() + "\\" + f.getName());newFile.mkdir();copy_File(f, newFile);}}}
}
2 文件加密:
加密:
使用对100取异或,将原文件加密成一个新文件,然后将这个新文件,再次对10取异或即可解密
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;public class shu15_21 {public static void main(String[] args) throws IOException {FileInputStream fis = new FileInputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\新建文件夹\\0.jpg");FileOutputStream fos = new FileOutputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\shu15\\ax.jpg");int len;while((len=fis.read())!=-1){fos.write(len^10);}fis.close();fos.close();}
}
解密:
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;public class shu15_21 {public static void main(String[] args) throws IOException {FileInputStream fis = new FileInputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\shu15\\ax.jpg");FileOutputStream fos = new FileOutputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\shu15\\axx.jpg");int len;while ((len = fis.read()) != -1) {fos.write(len ^ 10);}fis.close();fos.close();}
}
3 读取文件修改内容:
记事本内容:
代码实现
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collections;public class shu15_24 {public static void main(String[] args) throws IOException {//创建对象用于读取数据FileReader fr = new FileReader("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\shu15\\a.txt");//用于存储数据StringBuilder str = new StringBuilder();int len;while((len=fr.read())!=-1){str.append((char)len);}fr.close();String[] arrStr = str.toString().split("-");ArrayList<Integer> arr = new ArrayList<>();for (String s : arrStr) {int i = Integer.parseInt(s);arr.add(i);}Collections.sort(arr);//写入数据FileWriter fw = new FileWriter("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\shu15\\a.txt");for (int i = 0; i < arr.size()-1; i++) {fw.write(arr.get(i) + "-");}fw.write(arr.get(arr.size()-1)+"");fw.close();}
}
运行结果:
4 拷贝文件夹(四种方式)
- 字节流的基本流:一次读写一个字节
- 字节流的基本流:一次读写一个字节数组
- 字节缓冲流:一次读写一个字节
- 字节缓冲流:一次读写一个字节数组
1 字节流的基本流:一次读写一个字节
import java.io.*;public class shu15_27 {public static void main(String[] args) throws IOException {File f1 = new File("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\ax");File f2 = new File("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\Ax_2");f2.mkdir();coop(f1, f2);}private static void coop(File f1, File f2) throws IOException {for (File f : f1.listFiles()) {if (f.isFile()) {File newfile = new File(f2.getPath() + "\\" + f.getName());newfile.createNewFile();FileInputStream F1 = new FileInputStream(f);FileOutputStream F2 = new FileOutputStream(newfile);int len;while ((len = F1.read()) != -1) {F2.write(len);}F2.close();F1.close();} else {File newFile = new File(f2.getPath() + "\\" + f.getName());newFile.mkdir();coop(f, newFile);}}}
}
2 字节流的基本流:一次读写一个字节数组
import java.io.*;public class shu15_27 {public static void main(String[] args) throws IOException {File f1 = new File("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\ax");File f2 = new File("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\Ax_3");f2.mkdir();coop(f1, f2);}private static void coop(File f1,File f2) throws IOException {for (File f:f1.listFiles()){if(f.isFile()){File newfile = new File(f2.getPath()+"\\"+f.getName());newfile.createNewFile();FileInputStream F1 = new FileInputStream(f);FileOutputStream F2 = new FileOutputStream(newfile);int len;byte[]arr = new byte[1024];while((len=F1.read(arr))!=-1){F2.write(arr,0,len);}F2.close();F1.close();}else {File newFile = new File(f2.getPath()+"\\"+f.getName());newFile.mkdir();coop(f,newFile);}}}
}
3 字节缓冲流:一次读写一个字节
import java.io.*;public class shu15_27 {public static void main(String[] args) throws IOException {File f1 = new File("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\ax");File f2 = new File("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\Ax_4");f2.mkdir();cooop(f1, f2);}private static void cooop(File f1, File f2) throws IOException {for (File f : f1.listFiles()) {if (f.isFile()) {File newfile = new File(f2.getPath() + "\\" + f.getName());newfile.createNewFile();BufferedInputStream F1 = new BufferedInputStream(new FileInputStream(f));BufferedOutputStream F2 = new BufferedOutputStream(new FileOutputStream(newfile));int len;while ((len = F1.read()) != -1) {F2.write(len);}F2.close();F1.close();} else {File new_mkdir = new File(f2.getPath() + "\\" + f.getName());new_mkdir.mkdir();cooop(f, new_mkdir);}}}
}
4 字节缓冲流:一次读写一个字节数组
import java.io.*;public class shu15_27 {public static void main(String[] args) throws IOException {File f1 = new File("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\ax");File f2 = new File("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\Ax_4");f2.mkdir();cooop(f1, f2);}private static void cooop(File f1, File f2) throws IOException {for (File f : f1.listFiles()) {if (f.isFile()) {File newfile = new File(f2.getPath() + "\\" + f.getName());newfile.createNewFile();BufferedInputStream F1 = new BufferedInputStream(new FileInputStream(f));BufferedOutputStream F2 = new BufferedOutputStream(new FileOutputStream(newfile));int len;byte[] arr = new byte[1024];while ((len = F1.read(arr)) != -1) {F2.write(arr, 0, len);}F2.close();F1.close();} else {File new_mkdir = new File(f2.getPath() + "\\" + f.getName());new_mkdir.mkdir();cooop(f, new_mkdir);}}}
}
5 修改文本顺序
需求:将一个带顺序的文本排序
第一种代码实现:(使用Collections对集合进行排序)
import java.io.*;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;public class shu15_28 {public static void main(String[] args) throws IOException {//创建输入缓冲流对象BufferedReader F1 = new BufferedReader(new FileReader("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\shu15\\c.txt"));//将读取的数据放到集合中ArrayList<String> list = new ArrayList<>();String strLine;//readLine为读取一行(缓冲流特有)while ((strLine = F1.readLine()) != null) {list.add(strLine);}F1.close();//使用集合工具类对list进行排序(匿名内部类)Collections.sort(list, new Comparator<String>() {@Overridepublic int compare(String o1, String o2) {//以.分隔开(需要转义)return Integer.parseInt(o1.split("\\.")[0]) - Integer.parseInt(o2.split("\\.")[0]);}});//写入数据,并且不覆盖--newLine为换行(缓冲流特有)BufferedWriter F2 = new BufferedWriter(new FileWriter("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\shu15\\c.txt", true));F2.newLine();for (String s : list) {F2.write(s);//换行操作F2.newLine();}F2.close();}
}
第二种代码实现(使用TreeMap)
import java.io.*;
import java.util.*;public class shu15_29 {public static void main(String[] args) throws IOException {//创建输入缓冲流对象BufferedReader F1 = new BufferedReader(new FileReader("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\shu15\\c.txt"));//将读取的数据放到集合中TreeMap<Integer, String> map = new TreeMap<>();String strLine;//readLine为读取一行(缓冲流特有)while ((strLine = F1.readLine()) != null) {String[] split = strLine.split("\\.");map.put(Integer.parseInt(split[0]), strLine);}F1.close();System.out.println(map);//写入数据,并且不覆盖--newLine为换行(缓冲流特有)BufferedWriter F2 = new BufferedWriter(new FileWriter("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\shu15\\c.txt"));Set<Map.Entry<Integer, String>> entries = map.entrySet();F2.newLine();for (Map.Entry<Integer, String> entry : entries) {F2.write(entry.getValue());F2.newLine();}F2.close();}
}
6 软件运行次数
代码实现:-数据存储在本地文档
import java.io.*;public class shu15_30 {public static void main(String[] args) throws IOException {BufferedReader br = new BufferedReader(new FileReader("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\c.txt"));int count = Integer.parseInt(br.readLine());count++;if(count<=3){System.out.println("欢迎第"+count+"次使用");br.close();}else{System.out.println("次数使用结束");System.exit(0);}BufferedWriter bw = new BufferedWriter(new FileWriter("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\c.txt"));bw.write(count+"");bw.close();}
}
7 简单应用
import java.io.*;public class shu15_31 {public static void main(String[] args) throws IOException {//1FileInputStream fis = new FileInputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\b.txt");FileOutputStream fos = new FileOutputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\a.txt");int len;byte[] buffer = new byte[1024];while((len=fis.read(buffer))!=-1){fos.write(buffer,0,len);}fos.close();fis.close();//2FileReader f2 = new FileReader("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\b.txt");FileWriter f3 = new FileWriter("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\a.txt");int lens;while ((lens = f2.read()) != -1) {f3.write(lens);System.out.print((char) lens);}f3.write("helloworld");f3.write("helloworld", 0, 4);f3.write(new char[]{'a', 'c', 'b', 's', 'i'}, 0, 3);f2.close();f3.close();//3BufferedReader f4 = new BufferedReader(new FileReader("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\b.txt"));BufferedWriter f5 = new BufferedWriter(new FileWriter("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\a.txt"));String len;while((len= f4.readLine())!=null){f5.write(len);f5.newLine();}f4.close();f5.close();}}
六 转换流
转换流是字符流和字节流之间的桥梁。
- 可以将字节流转换为字符流然后调用相关方法eg:字节流使用转换流再使用字符缓冲流即可调用相关方法
FileReader 参数中可以指定编码方式Charset.format(" ")
FileWriter 参数中可以指定编码方式Charset.format(" ") 还有是否续写
InputStreamReader 可以指定字符编码方式“ ”
OutStreamWriter 可以指定字符编码方式“ ”
应用1:指定字符编码读取数据
import java.io.*;
import java.nio.charset.Charset;public class shu15_32 {public static void main(String[] args) throws IOException {//使用转换流进行读取InputStreamReader isr = new InputStreamReader(new FileInputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\b.txt"), "GBK");int len;while ((len = isr.read()) != -1) {System.out.print((char) len);}isr.close();//使用字符流读取FileReader fileReader = new FileReader("C:\\\\Users\\\\ABC\\\\IdeaProjects\\\\My-projects\\\\myfirstmodule\\\\src\\\\itheima\\\\b.txt", Charset.forName("GBK"));int len;while ((len = fileReader.read()) != -1) {System.out.print((char) len);}fileReader.close();}
}
应用2:使用指定字符编码进行写入
import java.io.FileOutputStream;
import java.io.FileWriter;
import java.io.IOException;
import java.io.OutputStreamWriter;
import java.nio.charset.Charset;public class shu15_33 {public static void main(String[] args) throws IOException {//1创建转换流对象OutputStreamWriter osw = new OutputStreamWriter(new FileOutputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\a.txt",true),"GBK");osw.write("你好");osw.close();//使用字符流FileWriter fw = new FileWriter("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\a.txt", Charset.forName("GBK"),true);fw.write("skladjk");fw.close();}
}
应用3 :将文本的编码方式更改写入另一个文本
import java.io.*;public class shu15_34 {public static void main(String[] args) throws IOException {InputStreamReader fis = new InputStreamReader(new FileInputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\b.txt"),"GBK");OutputStreamWriter fos = new OutputStreamWriter(new FileOutputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\a.txt"),"UTF-8");int len;while ((len = fis.read())!=-1){fos.write(len);}fis.close();fos.close();}
}
2
import java.io.*;
import java.nio.charset.Charset;public class shu15_34 {public static void main(String[] args) throws IOException {FileReader fr = new FileReader("C:\\\\Users\\\\ABC\\\\IdeaProjects\\\\My-projects\\\\myfirstmodule\\\\src\\\\itheima\\\\b.txt", Charset.forName("GBK"));FileWriter fw = new FileWriter("C:\\\\Users\\\\ABC\\\\IdeaProjects\\\\My-projects\\\\myfirstmodule\\\\src\\\\itheima\\\\a.txt", Charset.forName("UTF-8"))int len;while ((len = fr.read()) != -1) {fw.write(len);}fr.close();fw.close();}
}
阶段性总结
1
FileOutStream字节输出流->write
FileInputStream字节输入流->read
字节输出输入->大多数用于拷贝(读取数据汉字会出现编码问题)注意事项:read可以读取一个字节,字节数组,字节数组的一部分
write可以写入一个字节,字节数组,字节数组的一部分2
FileWriter字符输出流->write
FileReader字符输入流->read
字符输入输出->大多数用于文件读取写入
注意事项:write可以写入一个字符,一个字符数组,字符数组的一部分
一个字符串,字符串的一部分
read可以读取一个字符,读取一个字符数组,字符数组的一部分3
BufferedOutputStream字节缓冲输出流
BufferedInputStream字节缓冲输入流4
BufferedReader字符缓冲输入流 readLine
BufferedWriter字符缓冲输出流 newLine
注意事项:readLine一次读取一行newLine换行read的返回值为编码值
5
FileReader 转换流参数中可以指定编码方式Charset.format(" ")
FileWriter 转换流参数中可以指定编码方式Charset.format(" ")InputStreamReader 可以指定字符编码方式“ ”
OutStreamWriter 可以指定字符编码方式“ ”6
InputStreamReader,OutputStreamWriter,BufferedOutputStream,BufferedInputStream的首参数需要为字节流
BufferedReader,BufferedWriter的首参数要为字符流
七 序列化流
序列化流:可以将java中的对象写到本地文件中。(对象操作输出流)(是字节流的高级流)
代码实现->将一个对象写到本地文档中-类需要实现Serlizable接口
import java.io.Serializable;public class Student implements Serializable {private String name;private int age;public Student(String name, int age) {this.name = name;this.age = age;}public Student() {}public Student(String s){this.name = s.split("-")[0];this.age = Integer.parseInt(s.split("-")[1]);}public String getName() {return name;}public void setName(String name) {this.name = name;}public int getAge() {return age;}public void setAge(int age) {this.age = age;}@Overridepublic String toString() {return "Student [name=" + name + ", age=" + age + "]";}
}
方法调用:
import itheima.shu14.Student;import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;public class shu15_35 {public static void main(String[] args) throws IOException {//创建对象Student ax = new Student("ax", 100);//创建序列化对象ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\c.txt"));//写入对象oos.writeObject(ax);//释放资源oos.close();}
}
八 反序列化流
反序列化流:可以将序列化到本地文件中的对象读取到程序中来。(对象操作输入流)(高级流)
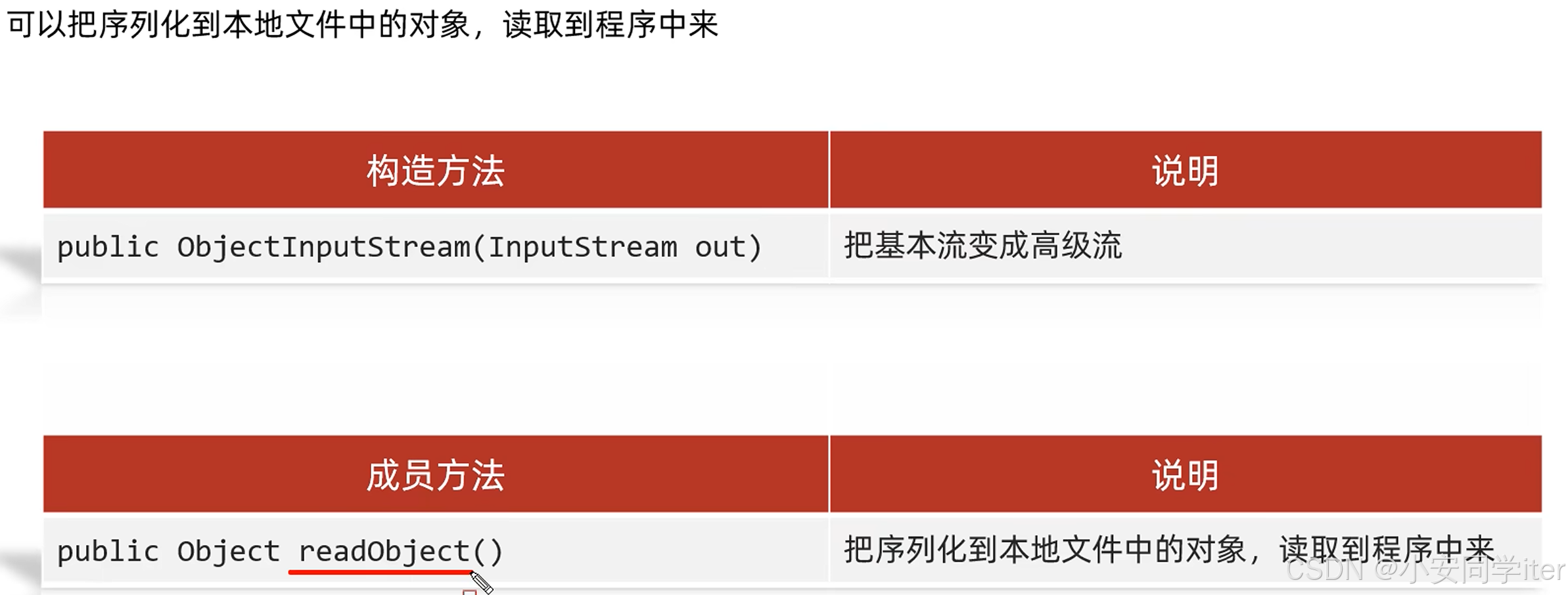
1
import java.io.*;public class shu15_36 {public static void main(String[] args) throws IOException, ClassNotFoundException {//创建反序列化输入流的对象ObjectInputStream ois = new ObjectInputStream(new FileInputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\c.txt"));//读取数据Object o = ois.readObject();System.out.println(o);//释放资源ois.close();}
}
2
javabean
3
反序列化流可以利用瞬态对对象内容进行加密(不会将当前属性序列化到当前本地文件中)
import java.io.Serial;
import java.io.Serializable;public class Student implements Serializable {@Serialprivate static final long serialVersionUID = 1L;private String name;private int age;//瞬态private transient String password;public Student(String name, int age, String password) {this.name = name;this.age = age;this.password = password;}public Student() {}public String getName() {return name;}public void setName(String name) {this.name = name;}public int getAge() {return age;}public void setAge(int age) {this.age = age;}public String getPassword() {return password;}public void setPassword(String password) {this.password = password;}@Overridepublic String toString() {return "Student [name=" + name + ", age=" + age + ", password=" + password + "]";}
}
序列化:
import itheima.shu14.Student;import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;public class shu15_35 {public static void main(String[] args) throws IOException {//创建对象Student ax = new Student("ax", 100,"886789");//创建序列化对象ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\c.txt"));//写入对象oos.writeObject(ax);//释放资源oos.close();}
}
反序列化:
import java.io.*;public class shu15_36 {public static void main(String[] args) throws IOException, ClassNotFoundException {//创建反序列化输入流的对象ObjectInputStream ois = new ObjectInputStream(new FileInputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\c.txt"));//读取数据Object o = ois.readObject();System.out.println(o);//释放资源ois.close();}
}
总结
练习:读取多个对象。
javabean
import java.io.Serial;
import java.io.Serializable;public class Student implements Serializable {@Serialprivate static final long serialVersionUID = 1L;private String name;private int age;//瞬态private transient String password;public Student(String name, int age, String password) {this.name = name;this.age = age;this.password = password;}public Student() {}public String getName() {return name;}public void setName(String name) {this.name = name;}public int getAge() {return age;}public void setAge(int age) {this.age = age;}public String getPassword() {return password;}public void setPassword(String password) {this.password = password;}@Overridepublic String toString() {return "Student [name=" + name + ", age=" + age + ", password=" + password + "]";}
}
序列化对象:
import itheima.shu14.Student;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;
import java.util.ArrayList;public class shu15_100 {public static void main(String[] args) throws IOException {//创建对象ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\c.txt"));//写入数据(放入集合中)ArrayList<Student> students = new ArrayList<>();students.add(new Student("ax5", 100, "as"));students.add(new Student("ax4", 1000, "aas"));students.add(new Student("ax3", 10000, "aaas"));students.add(new Student("ax2", 100000, "aaaas"));students.add(new Student("ax1", 1000000, "aaaas"));oos.writeObject(students);//释放资源oos.close();}
}
反序列化:
import itheima.shu14.Student;import java.io.*;
import java.util.ArrayList;public class shu15_101 {public static void main(String[] args) throws IOException, ClassNotFoundException {//创建反序列化对象ObjectInputStream ois = new ObjectInputStream(new FileInputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\c.txt"));//读取数据ArrayList<Student> o = (ArrayList<Student>) ois.readObject();for (Student s : o) {System.out.println(s);}ois.close();}
}
九 打印流
- 不能读只能写
1 字节打印流
构造方法:
成员方法
代码实现:
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.PrintStream;
import java.nio.charset.Charset;public class shu15_102 {public static void main(String[] args) throws FileNotFoundException {PrintStream ps = new PrintStream(new FileOutputStream("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\a.txt"), true,Charset.forName("UTF-8"));ps.println("Hello World");ps.print("你好");ps.println();ps.printf("%d",12);ps.close();}
}
运行结果:
2 字符打印流
构造方法(字符流底层有缓冲区,想要自动刷新需要开启)
成员方法:
代码实现:
import java.io.FileWriter;
import java.io.IOException;
import java.io.PrintWriter;public class shu15_104 {public static void main(String[] args) throws IOException {PrintWriter out = new PrintWriter(new FileWriter("C:\\Users\\ABC\\IdeaProjects\\My-projects\\myfirstmodule\\src\\itheima\\a.txt"), true);out.println("hskjhdkha");out.print("skdhajkhdh");out.close();}
}
补充:
十 解压缩流 /压缩流
使用idea解压文件:
import java.io.*;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;public class shu15_105 {public static void main(String[] args) throws IOException {File f1 = new File("C:\\Users\\ABC\\Downloads\\yolov5-5.0 (1).zip");File f2 = new File("D:\\ABC");shu15(f1, f2);}public static void shu15(File f1, File f2) throws IOException {//创建压缩流对象读取压缩包中的内容ZipInputStream zip = new ZipInputStream(new FileInputStream(f1));//获取压缩包中的对象文件ZipEntry len;while ((len = zip.getNextEntry()) != null) {System.out.println(len);if (len.isDirectory()) {//需要创建一个同样的文件夹File file = new File(f2, len.toString());file.mkdirs();} else {FileOutputStream fos = new FileOutputStream(new File(f2, len.toString()));int b;while ((b = zip.read()) != -1) {fos.write(b);}fos.close();zip.closeEntry();}}zip.close();}
}
使用idea压缩文件:
import java.io.*;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;public class shu15_106 {public static void main(String[] args) throws IOException {//创建File对象表示要压缩的文件File f1 = new File("D:\\ABC\\yolov5-7.0");//创建File对象博爱平时压缩包放在哪里File f2 = f1.getParentFile();//创建对象表示压缩包的路径File f3 = new File(f2,f1.getName()+".zip");//创建压缩流关联压缩包ZipOutputStream zos = new ZipOutputStream(new FileOutputStream(f3));//获取f1中的每一个文件,变成ZipEntry对象,放入压缩包中shu15(f1, zos, f1.getName());//释放资源zos.close();}private static void shu15(File f1, ZipOutputStream zos,String name) throws IOException {File[] files = f1.listFiles();for (File file : files) {System.out.println(file.getName());if(file.isFile()){//判断文件->变成zip_entry对象,放到压缩包中ZipEntry entry = new ZipEntry(name+"."+file.getName());zos.putNextEntry(entry);//读取文件中的数据,写到压缩包FileInputStream fis = new FileInputStream(file);int len;byte[] buffer = new byte[1024];while ((len = fis.read(buffer)) > 0) {zos.write(buffer, 0, len);}fis.close();zos.closeEntry();}else {shu15(file, zos, name+"\\"+file.getName());}}}
}
十一 Commons-io/Hutool
Commons-io是apache开源基金组织提供的一组有关IO操作的开源工具包。
2
实用网址:
Hutool🍬一个功能丰富且易用的Java工具库,涵盖了字符串、数字、集合、编码、日期、文件、IO、加密、数据库JDBC、JSON、HTTP客户端等功能。
入门和安装 (hutool.cn)