DROP database IF EXISTS `scott`;
CREATE database IF NOT EXISTS `scott` DEFAULT CHARACTER SET utf8 COLLATE utf8_general_ci;USE `scott`;DROP TABLE IF EXISTS `dept`;
CREATE TABLE `dept` (`deptno` int(2) unsigned zerofill NOT NULL COMMENT '部门编号',`dname` varchar(14) DEFAULT NULL COMMENT '部门名称',`loc` varchar(13) DEFAULT NULL COMMENT '部门所在地点'
);DROP TABLE IF EXISTS `emp`;
CREATE TABLE `emp` (`empno` int(6) unsigned zerofill NOT NULL COMMENT '雇员编号',`ename` varchar(10) DEFAULT NULL COMMENT '雇员姓名',`job` varchar(9) DEFAULT NULL COMMENT '雇员职位',`mgr` int(4) unsigned zerofill DEFAULT NULL COMMENT '雇员领导编号',`hiredate` datetime DEFAULT NULL COMMENT '雇佣时间',`sal` decimal(7,2) DEFAULT NULL COMMENT '工资月薪',`comm` decimal(7,2) DEFAULT NULL COMMENT '奖金',`deptno` int(2) unsigned zerofill DEFAULT NULL COMMENT '部门编号'
);DROP TABLE IF EXISTS `salgrade`;
CREATE TABLE `salgrade` (`grade` int(11) DEFAULT NULL COMMENT '等级',`losal` int(11) DEFAULT NULL COMMENT '此等级最低工资',`hisal` int(11) DEFAULT NULL COMMENT '此等级最高工资'
);insert into dept (deptno, dname, loc)
values (10, 'ACCOUNTING', 'NEW YORK');
insert into dept (deptno, dname, loc)
values (20, 'RESEARCH', 'DALLAS');
insert into dept (deptno, dname, loc)
values (30, 'SALES', 'CHICAGO');
insert into dept (deptno, dname, loc)
values (40, 'OPERATIONS', 'BOSTON');insert into emp (empno, ename, job, mgr, hiredate, sal, comm, deptno)
values (7369, 'SMITH', 'CLERK', 7902, '1980-12-17', 800, null, 20);insert into emp (empno, ename, job, mgr, hiredate, sal, comm, deptno)
values (7499, 'ALLEN', 'SALESMAN', 7698, '1981-02-20', 1600, 300, 30);insert into emp (empno, ename, job, mgr, hiredate, sal, comm, deptno)
values (7521, 'WARD', 'SALESMAN', 7698, '1981-02-22', 1250, 500, 30);insert into emp (empno, ename, job, mgr, hiredate, sal, comm, deptno)
values (7566, 'JONES', 'MANAGER', 7839, '1981-04-02', 2975, null, 20);insert into emp (empno, ename, job, mgr, hiredate, sal, comm, deptno)
values (7654, 'MARTIN', 'SALESMAN', 7698, '1981-09-28', 1250, 1400, 30);insert into emp (empno, ename, job, mgr, hiredate, sal, comm, deptno)
values (7698, 'BLAKE', 'MANAGER', 7839, '1981-05-01', 2850, null, 30);insert into emp (empno, ename, job, mgr, hiredate, sal, comm, deptno)
values (7782, 'CLARK', 'MANAGER', 7839, '1981-06-09', 2450, null, 10);insert into emp (empno, ename, job, mgr, hiredate, sal, comm, deptno)
values (7788, 'SCOTT', 'ANALYST', 7566, '1987-04-19', 3000, null, 20);insert into emp (empno, ename, job, mgr, hiredate, sal, comm, deptno)
values (7839, 'KING', 'PRESIDENT', null, '1981-11-17', 5000, null, 10);insert into emp (empno, ename, job, mgr, hiredate, sal, comm, deptno)
values (7844, 'TURNER', 'SALESMAN', 7698,'1981-09-08', 1500, 0, 30);insert into emp (empno, ename, job, mgr, hiredate, sal, comm, deptno)
values (7876, 'ADAMS', 'CLERK', 7788, '1987-05-23', 1100, null, 20);insert into emp (empno, ename, job, mgr, hiredate, sal, comm, deptno)
values (7900, 'JAMES', 'CLERK', 7698, '1981-12-03', 950, null, 30);insert into emp (empno, ename, job, mgr, hiredate, sal, comm, deptno)
values (7902, 'FORD', 'ANALYST', 7566, '1981-12-03', 3000, null, 20);insert into emp (empno, ename, job, mgr, hiredate, sal, comm, deptno)
values (7934, 'MILLER', 'CLERK', 7782, '1982-01-23', 1300, null, 10);insert into salgrade (grade, losal, hisal) values (1, 700, 1200);
insert into salgrade (grade, losal, hisal) values (2, 1201, 1400);
insert into salgrade (grade, losal, hisal) values (3, 1401, 2000);
insert into salgrade (grade, losal, hisal) values (4, 2001, 3000);
insert into salgrade (grade, losal, hisal) values (5, 3001, 9999);
可以看到有三张表部门表dept,员工表emp,工资等级表salgrade。
多表查询
在MySQL中,多表查询是指在一个查询中涉及到两个或更多的表。这种查询通常用于从多个相关的数据表中检索数据。多表查询的关键在于使用连接条件来关联不同表中的数据。
内连接
内连接(Inner Join)是一种SQL操作,用于从两个或多个表中检索相关数据。内连接通过在连接条件满足的情况下返回两个表中匹配的行。内连接仅返回那些在连接条件下有匹配的行,其他不匹配的行将被排除。
select 列名1,列名2 from 表名1 inner join 表名2 on 条件;
以上为内连接的标准写法,还有以下写法和内连接得到的效果一致,更简单。
select 列名1,列名2... from 表名1,表名2... where 条件;
select emp.ename,emp.sal,dept.dname from emp inner join dept on emp.deptno=dept.deptno;
写法二
select emp.ename,emp.sal,dept.dname from emp,dept where emp.deptno=dept.deptno;
左外连接
select 列名1,列名2... from 表名1 left join 表名2 on 条件;
左外连接(Left Join)是一种SQL操作,用于从两个或多个表中检索相关数据。左外连接返回左表中的所有记录,以及右表中与左表匹配的记录,如果右表中没有匹配项,左表的记录仍然会被返回,但右表的字段会显示为 NULL。
现在创建两张表学生表stu和成绩表exam,并插入数据,其代码如下:
create table stu (id int, name varchar(30)); -- 学生表
insert into stu values(1,'jack'),(2,'tom'),(3,'kity'),(4,'nono');
create table exam (id int, grade int); -- 成绩表
insert into exam values(1, 56),(2,76),(11, 8);
select * from stu left join exam on stu.id=exam.id;
右外连接
右外连接(Right Join)是一种SQL操作,用于从两个或多个表中检索相关数据。右外连接返回右表中的所有记录,以及左表中与右表匹配的记录。如果左表中没有匹配项,左表的字段会显示为 NULL。
select 列名1,列名2... from 表名1 right join 表名2 on 条件;
select * from stu right join exam on stu.id=exam.id;
自连接
自连接是一种特殊的多表查询,可以理解为自己与自己之间进行多表查询。
select 列名1,列名2... from 表名 as 别名1, 表名 as 别名2;
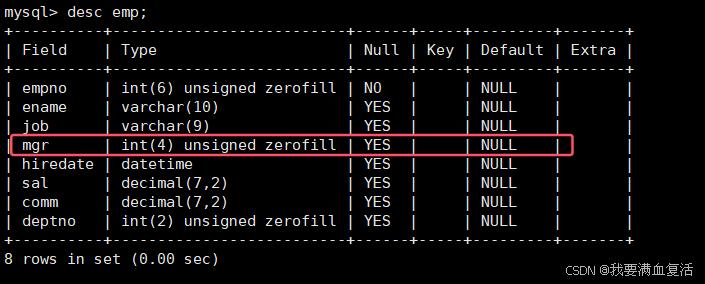
select distinct leader.empno,leader.ename from emp leader,emp worker where worker.mgr=leader.empno;
子查询
子查询(Sub Query)是指在一个查询语句中嵌套另一个查询语句,其中内层查询的结果作为外层查询的条件或数据来源。子查询也被称为嵌套查询或内层查询,而包含子查询的查询语句被称为外层查询或父查询。
单行子查询
select ... from ... where 列名 = (select ... from ...);
select * from dept=(select deptno from emp where ename='smith');
多行子查询
select ... from ... where 列名 in (select ... from ...);
select ... from ... where 列名 比较操作符 all(select ... from ...);
select ... from ... where 列名 比较操作符 any(select ... from ...);
在单行子查询中,子查询的结果是单行数据,所以能进行=
。如果是多行查询,那么此时就不能进行判等,而是使用in
,all
,any
这三个关键字,来进行范围判断。
in:判断是否是多行数据中的一个
select ename,job,sal,deptno from emp where job in (select job from emp where deptno=10) and deptno!=10;
select ename,job,sal,deptno from emp where sal > all (select sal from emp where deptno=30);
select ename,job,sal,deptno from emp where sal > any (select sal from emp where deptno=30);
多列子查询
select ... from ...
where (列1, 列2...) 逻辑运算符 (select 列1, 列2... from ...);
查询和SMITH的部门和岗位完全相同的所有雇员,不含SMITH本人
select ename from emp where (deptno,job)=(select deptno,job from emp where ename='smith') and ename!='smith';
在from子句中使用子查询
select ... from (select ... from ...) as 别名 where ...;
select ename,deptno,sal,format(asal,2) from emp,(select deptno de,avg(sal) asal from emp group by deptno) tmp where sal>asal and deptno=de;
select ename,sal,emp.deptno from emp,(select deptno,max(sal) msal from emp group by deptno) tmp where sal=msal and emp.deptno=tmp.deptno;
合并查询
在实际应用中,为了合并多个select的执行结果,可以使用集合操作符 union,union all。
union
该操作符用于取得两个结果集的并集。当使用该操作符时,会自动去掉结果集中的重复行。
select ... from ... union select ... from ...;
select ename,sal,job from emp where sal>2500 union select ename,sal,job from emp where job='manager';
union all
select ... from ... union all select ... from ...;
将工资大于2500或职位是MANAGER的人找出来
select ename,sal,job from emp where sal>2500 union all select ename,sal,job from emp where job='manager';