餐厅点餐平台导航
【餐厅点餐平台|一】项目描述+需求分析 https://blog.csdn.net/weixin_46291251/article/details/126414430
【餐厅点餐平台|二】总体设计 https://blog.csdn.net/weixin_46291251/article/details/126422811
【餐厅点餐平台|三】模块设计 https://blog.csdn.net/weixin_46291251/article/details/126422826
【餐厅点餐平台|四】UI设计+效果展示 https://blog.csdn.net/weixin_46291251/article/details/126422844
【源码下载】 https://download.csdn.net/download/weixin_46291251/86404328
文章目录
- 一:项目描述
- 二:需求分析
- 2.1业务流分析
- 2.2编程环境以及相关工具
一:项目描述
地大后勤集团餐饮部需要研发一套系统,用于对地大体系内所有餐饮部门进行运维管理。
背景:在校园里面通常会设立食堂,有可能自己经营某种类型的餐食,也可能承包给各个个体户,丰富师生饮食。其收费与经营的基本思路如下所示:
请结合上图进行餐饮系统的设计与实现,要求必须考虑内容如下:
- 就餐时价格如何确定,套餐、点餐、称重?
- 如何付费,一卡通、二维码、收付款?
- 考虑扩展性,将来可能增加的餐饮类型?(顿顿有你)
- 校外人员、校内人员收费标准可否改变?
- 过节时的加餐如何管理?
- 学校后勤集团如何结算各服务窗口的租赁费用?
为平抑物价,学校后勤集团控制菜品价格。
- 在就餐时,窗口服务人员选择就餐者所订购饮食种类(如红烧牛肉面、两荤一素、餐食重量),发送至后台后,计算出价格,反应到打卡机上,就餐者刷卡购餐。
- 如窗口增加餐品类型,则由管理员制定菜品价格,然后将菜品名称、价格、窗口ID等输入到服务器中。窗口服务人员的打卡机上则显示出菜品名称,以便就餐者选择。
- 每周日晚上10点,饮食中心结算每个窗口的经营额,抽取租赁费用后,返还剩余金额。
二:需求分析
2.1业务流分析
基本的注册和登陆业务:
我们根据题目要求,主要分析出两种用户,一种是管理员用户(即题目中所提到的位裁片指定价格的管理员),另一种是普通商户用户(即题目中所提到的窗口服务人员)。我们分析两种用户的关联,结合食堂实际的情况,管理员属于食堂管理员(后勤集团的管理者,比如某某食堂的经理),服务人员属于(窗口所有者),两者的联系在于两者属于同一个组织(比如学一食堂),因此我们抽象出一个组织的实体。在登陆时主要就是对比数据库数据,注册时管理员可以选择注册时开设一个组织,而普通用户则不能选择开设组织。
管理员对用户和窗口的管理:
结合题目要求和我校实际情况,学校的后勤集团管理员能够控制菜品价格,控制窗口是否能够开设,控制某个店的老板能否参与某食堂的运营中来。我们认为一个可行的菜品价格控制方法不是管理员直接设置菜品价格(我们认为这种单向的操作并不是一个良好的决定),我们设计的想法是,窗口的服务人员(比如窗口的老板)向管理员提出一个申请菜品价格的申请,由管理员批准是否能够设置为该价格。
(在该部分考虑餐饮拓展即服务人员提出一个增加菜品的申请以及过节时加餐的处理即开设一个临时窗口,对应要求3和要求5)
用餐收费流程:
分析题目,窗口的服务人员选择好用餐者的订购的饮食的品种后,由后台直接计算价格并反映到打卡机上,我们的想法是由窗口服务人员设置好饮食品种(用餐者可见)后,传送到后台进行计算,在订单结算时,商户可以选择指定对某餐品进行打折(打折以吸引顾客)。分析后我们认为得设计一个收费器,用于响应用户付款,主要是向收费器接口发送收费命令,等待相应和超时处理。
(在该部分考虑了用餐价格的确定即后台计算,以及如何付费即用收费器判断,还考虑了不同人员的收费标准即打折,对应要求1,要求2和要求4)
饮食中心结算:
每周日晚上10点,饮食中心结算窗口经营额,抽取租赁费用,返还剩余金额。我们的想法是在服务器上部署一个定时脚本,到每周日晚上10点时自动触发。平时的时候,用户付费直接进入饮食中心账户(即组织的账户),结算时,饮食中心根据窗口订单情况,给商家进行结算。租赁费我们初步设想是分级的,类似交税,结算后,将钱转入商户账户(即窗口人员账户)。
(在该部分考虑了租赁费用的结算即定时脚本实现,对应要求6)
基于以上分析,分析并绘制出以下总体架构
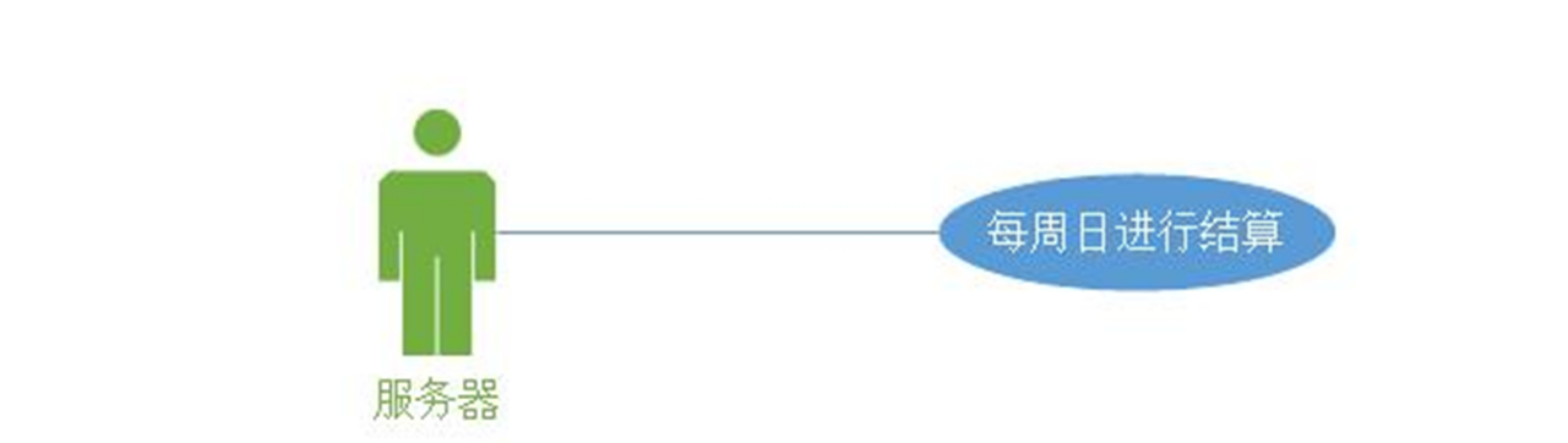
2.2编程环境以及相关工具
MySQL:
-
优化的 SQL 查询算法,有效地提高查询速度。(效率高)
-
提供 TCP/IP、ODBC 和 JDBC 等多种数据库连接途径。(支持C++连接)提供多语言支持,常见的编码如中文的 GB 2312、BIG 5,日文的 Shift_JIS 等都可以用作数据表名和数据列名。(中文不会出错,不会乱码)
-
既能够作为一个单独的应用程序应用在客户端服务器网络环境中,也能够作为一个库而嵌入其他的软件中。(方便在程序中嵌入,同时可以在脚本中直接调用嵌入式库)
Navicat:
-
使用Navicat浏览和修改数据,插入、编辑、删除数据或复制和粘贴记录到数据表形式的数据编辑器,Navicat将运行相应的命令(例如INSERT 或UPDATE),免除写复杂的SQL。(便于操作)
-
可以保证快捷地输入无错误的代码。(高亮标识表明提示代码正确性)
-
运用精密的数据库设计和模型工具,可以用图形表达数据库。使用实体关系图表来显现数据库结构及关系,这样你就可以更容易塑造,建立和理解复杂的数据库。(可以快捷地建立出数据库地模型)
-
用户管理功能提升和管理每个用户的管理权限,不需输入任何命令,在数分钟内就能创建和编辑用户角色,借助这个精确控制的层面,可以在不影响数据库的安全性下,创建规则并让用户访问数据库。(连接方便,安全性高)
Qt:
-
需要的库,在其API中都可以找到,并且有很高的集成度,不仅速度快并且简单易用。(用户友好)
-
Qt支持2D/3D图形渲染,并且支持OpenGL。(强大的图形库,便于ui设计)
-
模块化程度高,重用性好;使用相对安全的信号与槽机制来代替回调函数,各个函数之间的协调更加灵活。(便于程序设计,且安全性高)
-
通过更改编译步骤,是可以在其他嵌入式或者linux界面上被支持的(扩展性好)
Clion:(MAC)
-
非常好的智能感知功能,自动折叠、高亮、自动补全、类型推断都很好。Autofix工作的很好。(用户友好)
-
重构很方便,像inline函数、extract成员函数、常数,pull up/pull down、修改签名这些功能都有。调试功能很方便,可以自动解析STL容器。
-
继承了jetbrains系ide的很多优点,像方便的vim插件和keymap调整,滚动条预览,与VCS的紧密集成等等。
-
跨平台,支持CMake/gcc/clang/mingw/cygwin/gdb。虽然不多,但是其实基本上也够用了。(可以保证和其他成员不起冲突)
Gitee:
- 访问速度快,对国内用户比较友好
SourceTree
-
实用的git gui程序,各种分支迭代更新一目了然(可视化良好,可读性好)
-
操作简单快捷,不用刻意去记各种繁琐的命令(对用户友好)
-
可以在选项里直接配置diff和merge的工具,对于unity yaml merge来说极其好用。(功能强大)
Visio
-
模板库强大,基本覆盖所有学科的相关图的绘制(方便直接调用)
-
绘图专业,有利于提升工作效率(开发效率高)
-
生成格式多样,Visio能生成大约20多种不同格式的文件,与其他各类专业软件交互良好(便于与其他软件交互,接口广)
Drawio
-
开源免费(对学生党友好)
-
界面简介,美观,使用方便(美观)
-
占用空间小,轻便,不用担心存储空间的问题(小巧玲珑)
-
导出格式丰富,与其他软件交互良好(交互性不错)