1 Introduction
In this assignment, you will implement a (heavily) simplified version of the video game ”Into The Breach”. In this game players defend a set of civilian buildings from giant monsters. In order to achieve this goal, the player commands a set of equally giant mechanical heroes called ”Mechs”.
There are a variety of enemy and mech types, which each behave slightly differently. Gameplay is described in section 3 of this document.
Unlike assignment 1, in this assignment you will be using object-oriented programming and following the Apply Model-View-Controller design pattern shown in lectures. In addition to creating code for modelling the game, you will be implementing a graphical user interface (GUI). An example of a
final completed game is shown in Figure 1.
2 Getting Started
Download a2.zip from Blackboard — this archive contains the necessary files to start this assign ment. Once extracted, the a2.zip archive will provide the following files:
a2.py This is the only file you will submit and is where you write your code. Do not make changes to any other files.
a2 support.py Do not modify or submit this file , it contains pre-defined classes, functions, and con stants to assist you in some parts of your assignment. In addition to these, you are encouraged to create your own constants and helper functions in a2.py where possible.
levels/ This folder contains a small collection of files used to initialize games of Into The Breach . In addition to these, you are encouraged to create your own files to help test your implementation where possible.
3 Gameplay
This section describes an overview of gameplay for Assignment 2. Where interactions are not ex
plicitly mentioned in this section, please see Section 4.
3.1 Definitions
Gameplay takes place on a rectangular grid of tiles called a board , on which different types of entities can stand. There are three types of tile: Ground tiles, mountain tiles, and building tiles. Building
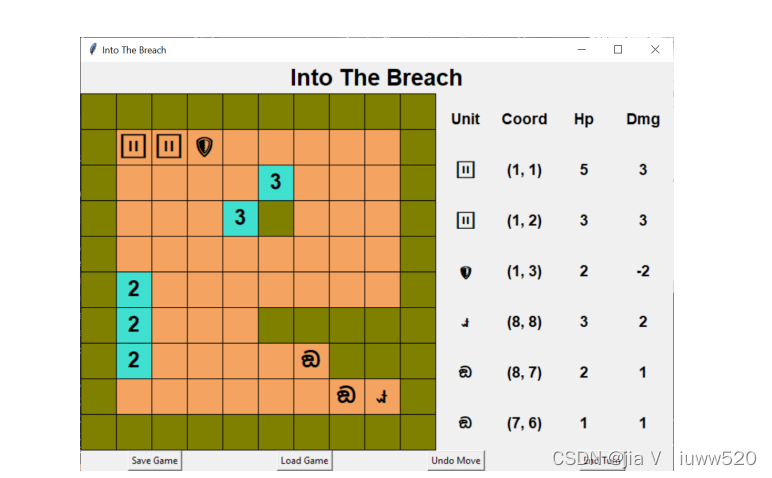
Figure 1: Example screenshot from a completed implementation. Note that your display may look slightly different depending on your operating system.
tiles each possess a given amount of health , which is the amount of damage they can suffer before they are destroyed . A building is destroyed if its health drops to 0. A tile may be blocking , in which case entities cannot stand on it. Tiles that are not blocking may have a maximum of one entity standing on them at any given time. Ground tiles are never blocking, mountain tiles are always blocking, and building tiles are blocking if and only if they are not destroyed.
Entities may either be Mechs , which are controlled by the player, or Enemies , which attack the player’s mechs and buildings. There are two types of mech; the Tank Mech and the Heal Mech . There are also two types of enemy; the Scorpion and the Firefly . Each entity possesses 4 characteristics:
1. position : the coordinate of the tile within the board on which the entity is currently standing.
2. health : the remaining amount of damage the entity can suffer before it is destroyed . An entity is destroyed the moment its health drops to 0, at which point it is immediately removed from the game.
3. speed : the number of tiles the entity can move during its movement phase (see below for details). Entities can only move horizontally and vertically; that is, moving one tile diagonally is considered two individual movements.
4. strength : how much damage the entity deals to buildings and other entities (i.e. the amount by which it reduces the health of attacked buildings or entities).
The game is turn based, with each turn consisting of a player movement phase, an attack phase, and an enemy movement phase. During the player movement phase, the player has the option to move each of the mechs under their control to a new tile on the grid. During the attacking phase, each
mech and enemy perform an attack: an action that can damage mechs, enemies, or even buildings.
Each enemy, mech, and building can only receive a certain amount of damage. If a mech or enemy is destroyed before they attack during a given attack phase, they do not attack during that attack phase. During the enemy movement phase, each enemy chooses a tile as their objective, and then
moves to a new tile on the grid such that they are closer to their objective. The order in which 2mechs and enemies move and attack is determined by a fixed priority that will be displayed to the user at all times.