👀日报&周刊合集 | 🎡生产力工具与行业应用大全 | 🧡 点赞关注评论拜托啦!
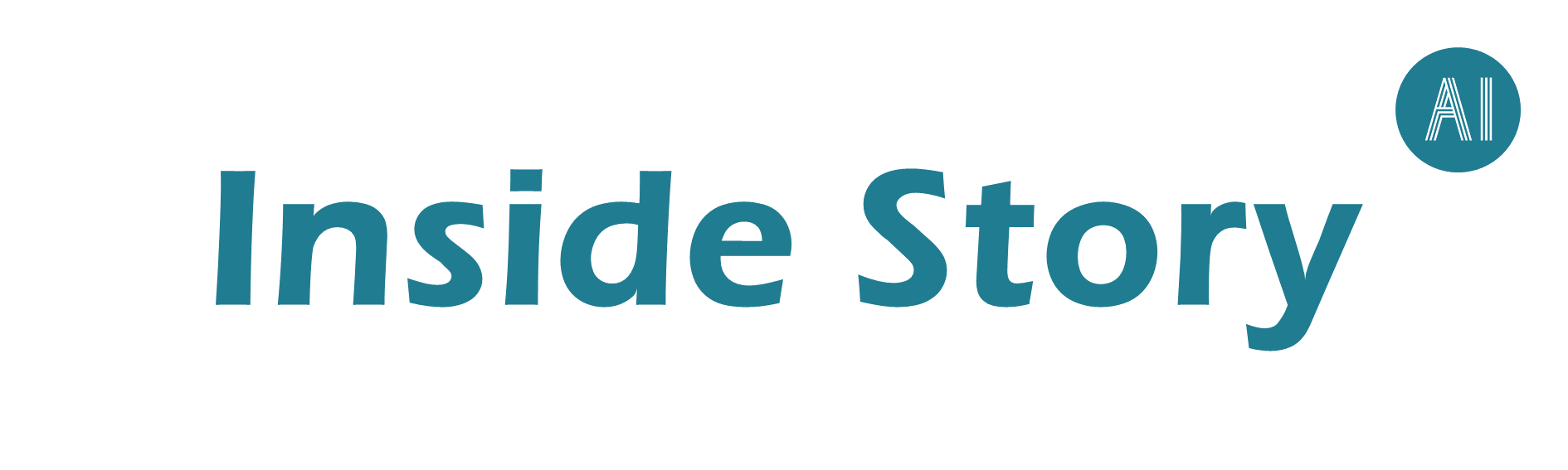
🤖 面向虚拟世界的生成式AI市场全景图
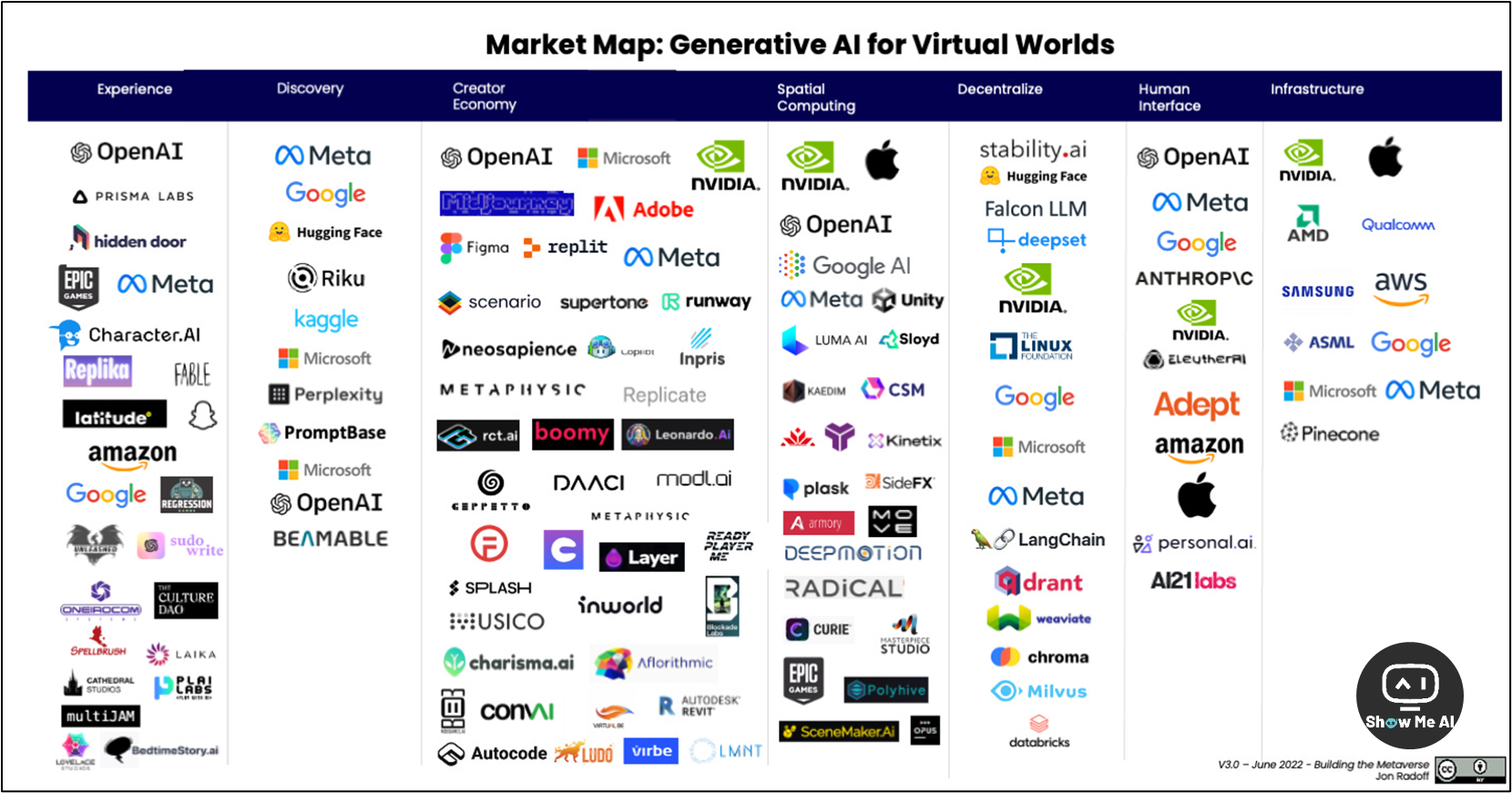
作者在这篇文章中探讨了生成式AI在虚拟世界的应用,并绘制了 Market Map V3.0 (市场全景图),来展示Experiences、Discovery、Creator Economy、Spatial Computing、Decentralization、Human Interface、Infrastructure 各部分的含义与价值链条。
虚拟世界的复杂性在于它们的内部结构、网络效应和不断变化的组件。生成式AI在解决关键技术问题后,有潜力协助解决这些复杂的问题 ⋙ 来源
🤖 WPS AI 最全申请与使用手册:推荐国内版,真香!
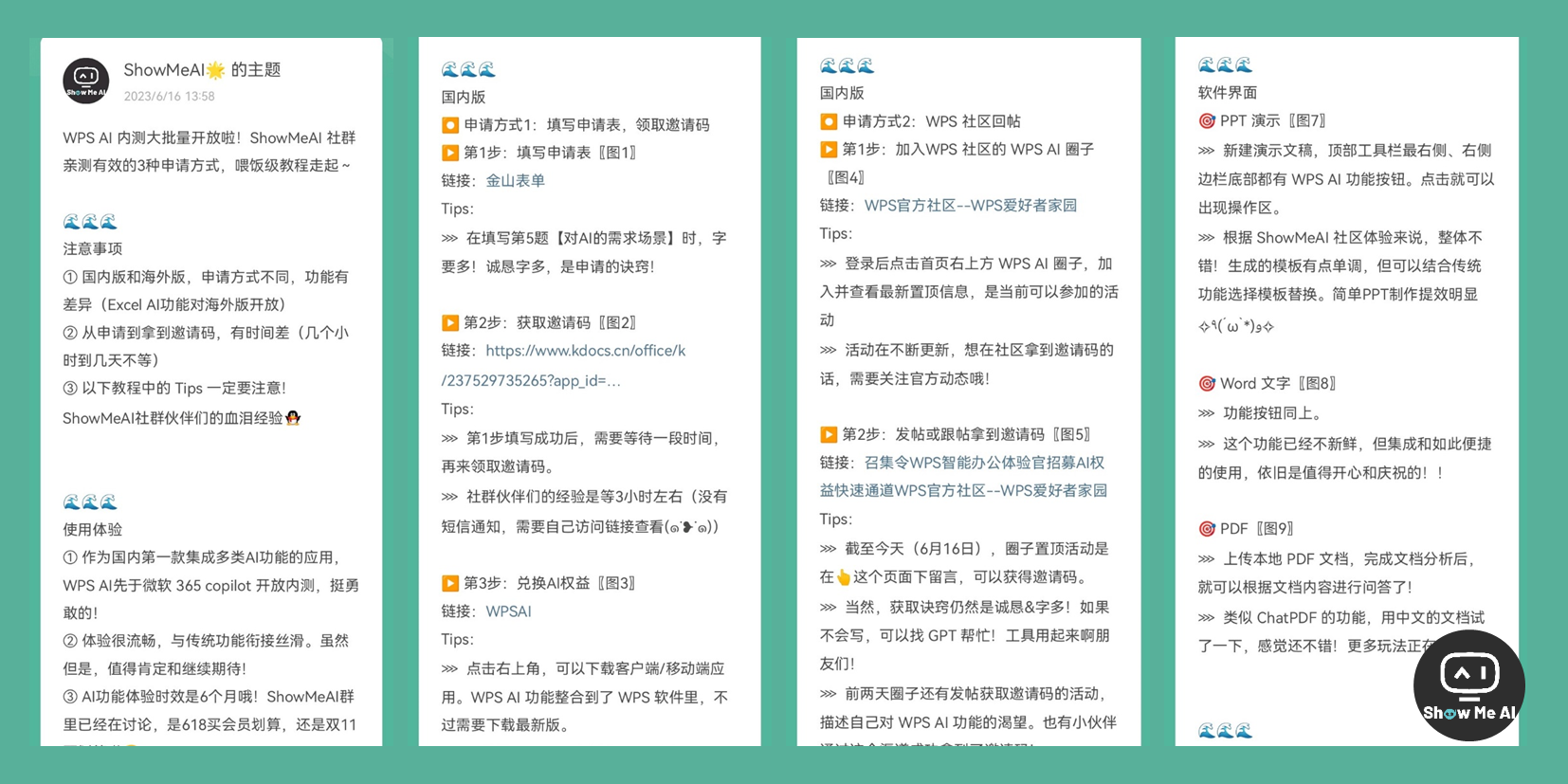
WPS AI 今天放出了大量国内版和海外版的内测名额,社群小伙伴们尝试得不亦乐乎!!我也第一时间整理了一份「WPS AI 最全申请与使用手册」,非常细致地讲解了3种申请渠道、申请注意事项和试用方法。
综合社区体验和通过率,建议小伙伴们使用教程中「申请方式1」,一般几个小时后都能成功拿到邀请码。国内版申请成功后,可以免费体验6个月 (海外版本只有7天),而且 WPS 社区还一直掉福利,简直不能更开心好嘛!! ⋙ 点击查看完整教程
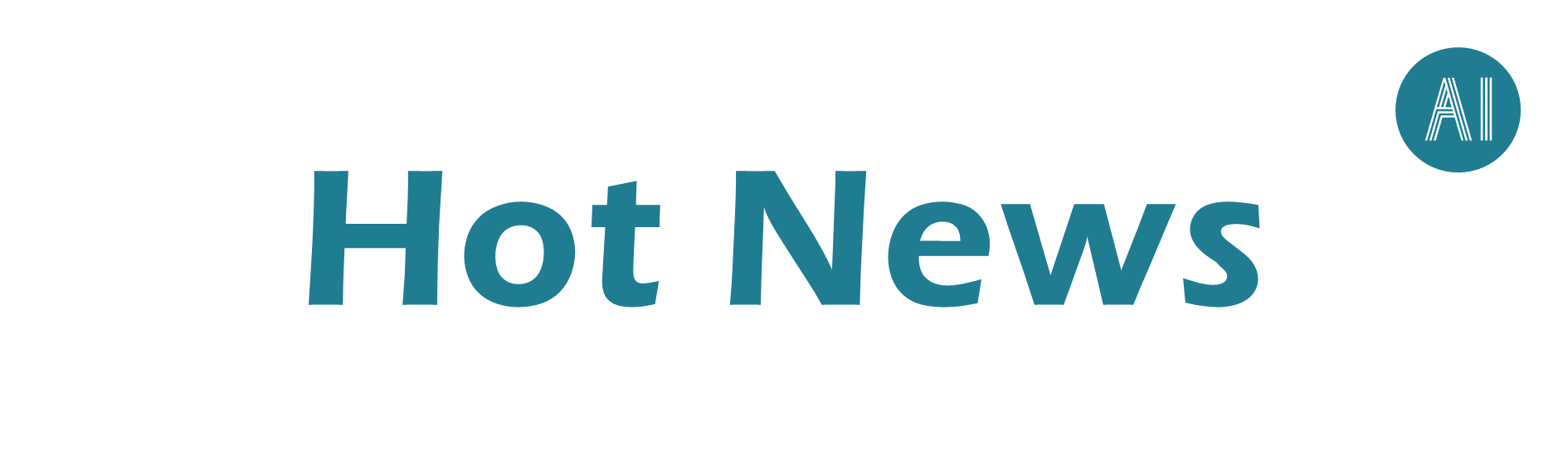
🤖 Perplexity 推出 Pro 服务和个性化功能
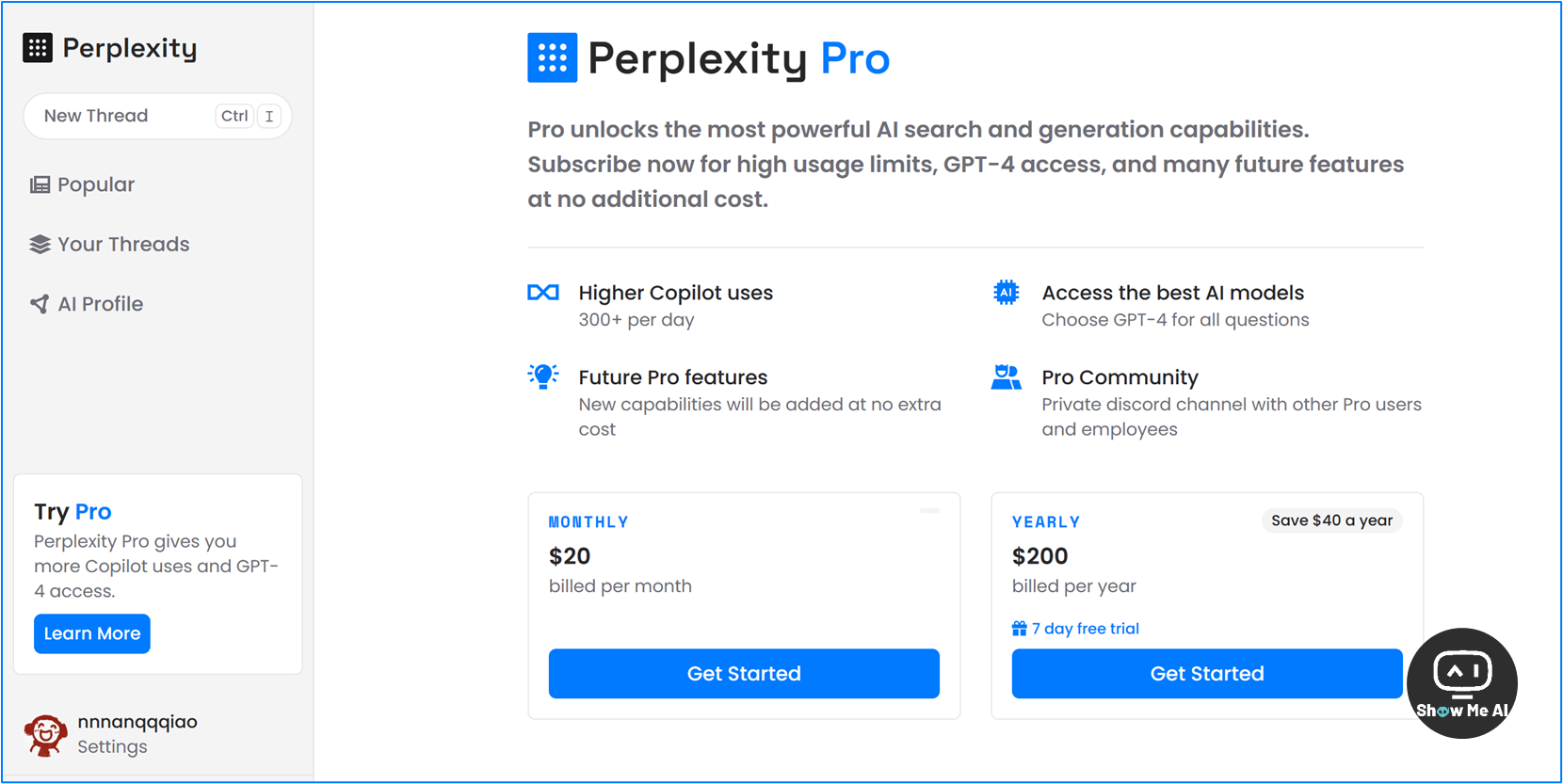
https://www.perplexity.ai/pro
Perplexity AI 是基于OpenAI API的搜索引擎,基于大型语言模型为问题提供准确全面的答案,主打精准度高。
今天,Perplexity AI 再次发布重大升级,推出了 Pro 付费版服务:更快的响应时间、更多的API调用次数、更高级的模型 ,以及未来有限使用Pro 特性等。
费用方面,按月订阅的价格是20美元,那么全年需要240美元;按年订阅则可以节省40美元,只需支付200美元。
🤖 Stack Overflow:70% 程序员已使用各种AI编程工具
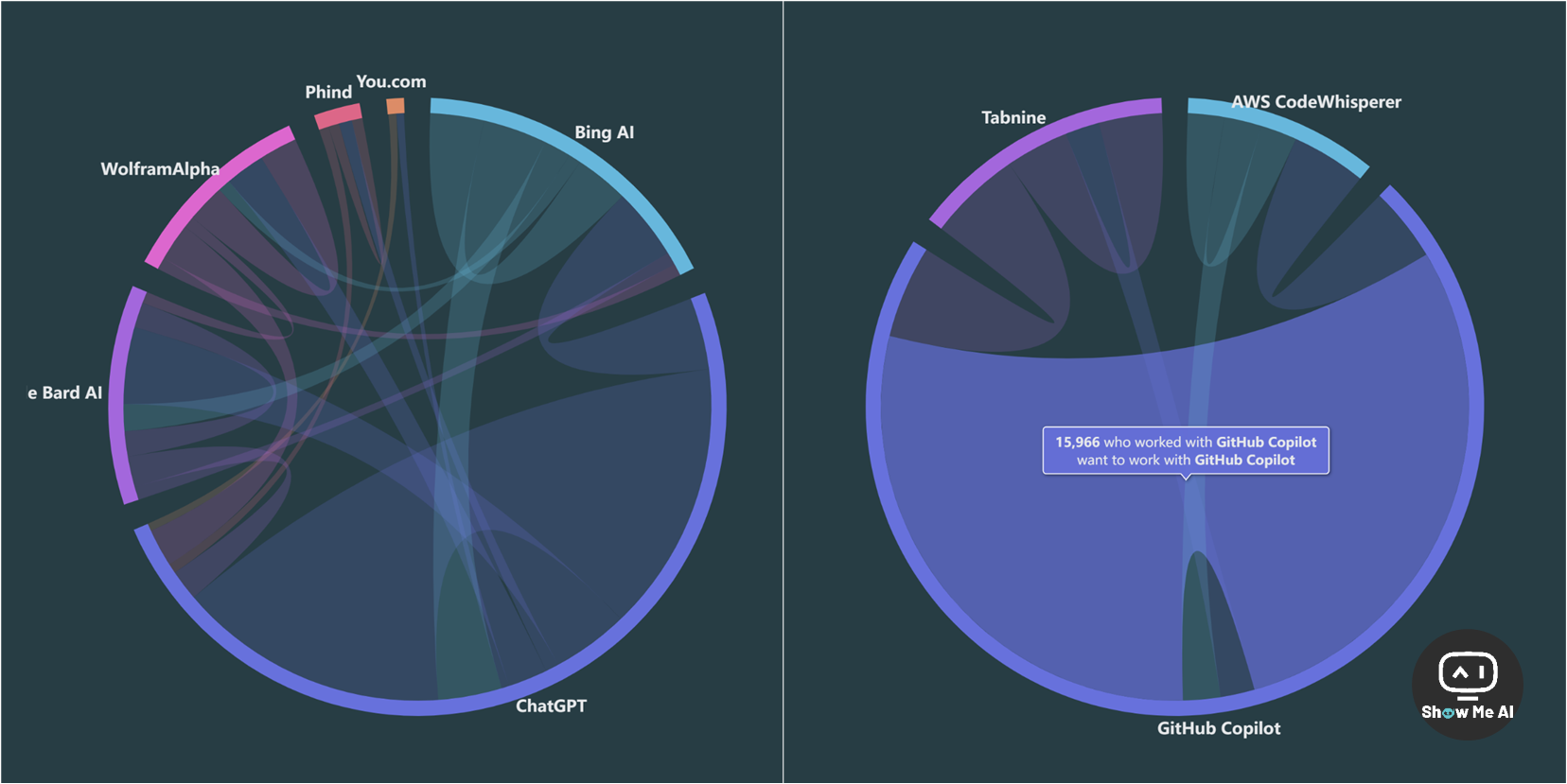
一年一度的 Stack Overflow 开发者大调查又来了!今年共约9万人参与问卷,最大的变化是加入了 ChatGPT 相关的内容。程序员是否比大家想象地更能接受AI工具呢?结果显示:
▢ 有83% 的受访者都表示用过 ChatGPT 来进行搜索,相比之下,第二名的 Bing AI 只占20%
▢ 不过有42%的 ChatGPT 用户希望明年尝试谷歌 Bard 或 Bing AI
▢ AI编程上,有55% 的受访者使用过 GitHub Copilot,相比之下,AWS的CodeWhisperer则只有5% ⋙ 2023 Developer Survey
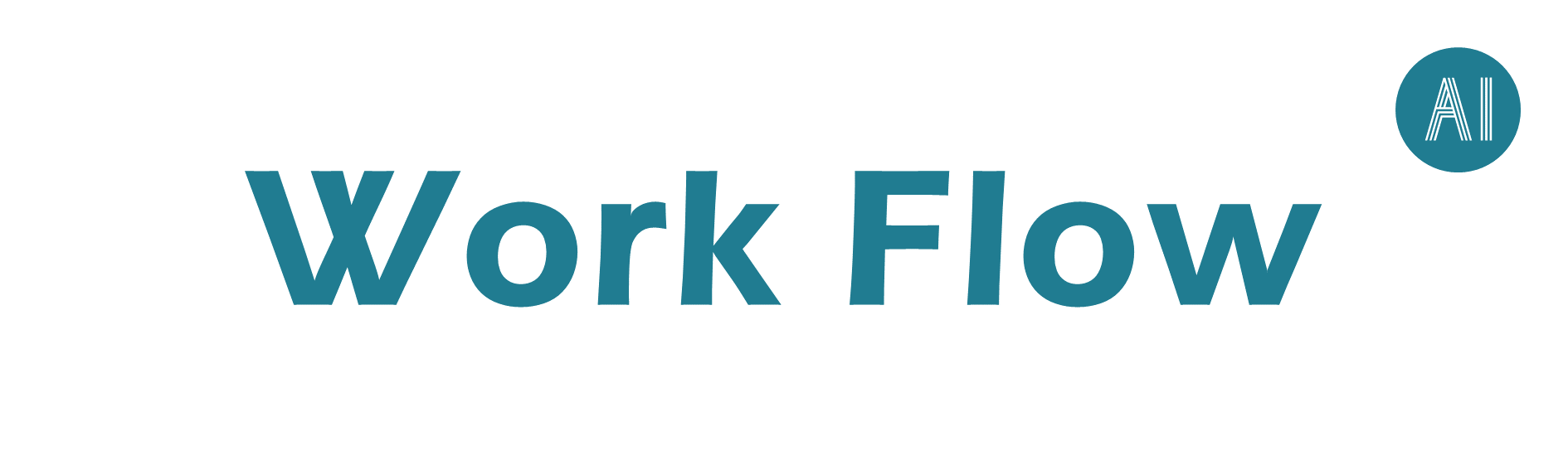
🤖 不再为游戏配乐发愁,AIGC制作音乐的时代已到来!
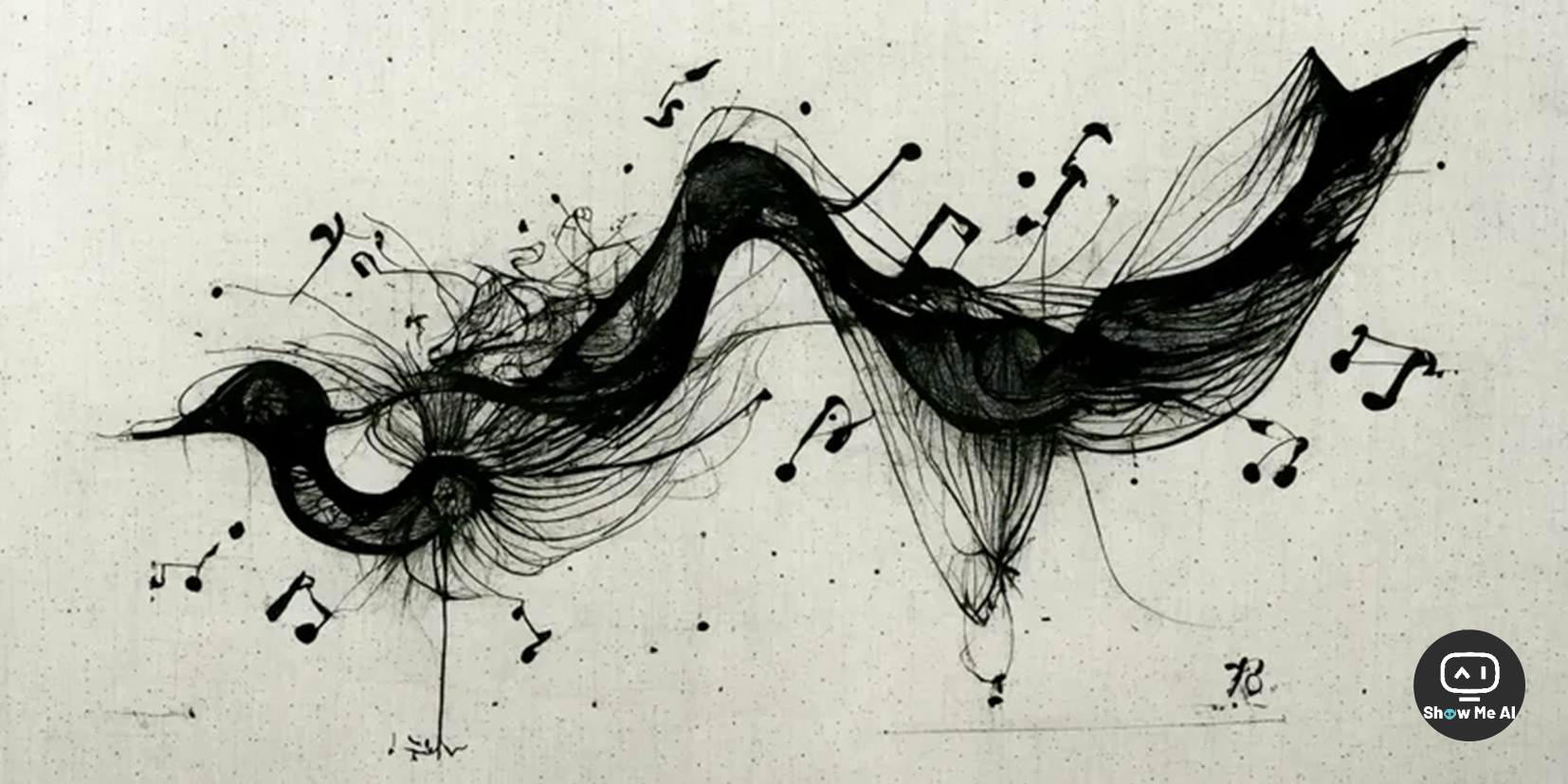
在文生图、文生文赛道的成功产品的万丈光芒之下,人们似乎忽视了另一条探索起步更早、重要性也并不逊色的AIGC赛道:音乐和音效。事实上,作为结合了声光效的综合型娱乐媒介,游戏公司们都在快速上马 AIGC 开发流程。
作者回顾了大模型时代到来前后,市面上的多款基于AI的商业化音乐产品,如 Amper Music、AIVA、OpenAI Jukebox、Google MusicLM等,并分享了 AIGC 在实际游戏开发流程中所能满足的声音需求:
▢ 角色配音:在眼下的角色配音领域,AIGC早已出现了不少的现成的用例,表现出了极高的应用潜力;这很大程度上得益于TTS (语音合成) 技术的飞速发展
▢ 音效:某二次元头部大厂的相关负责人表示,正在实验通过AIGC生成多种不同风格Demo的形式,为音效师提供创作灵感和原型,进而加速创作过程
▢ 配乐:暂时无法满足精品游戏的高端需求,但为低成本的小游戏、超休闲游戏等提供了无限的游戏配乐创作空间
作者是游戏行业资深从业者,将行业发展历程娓娓道来,并清晰具体地阐明了行业发展现状,以及最常使用的技术和工具 ⋙ 感兴趣可以阅读原文
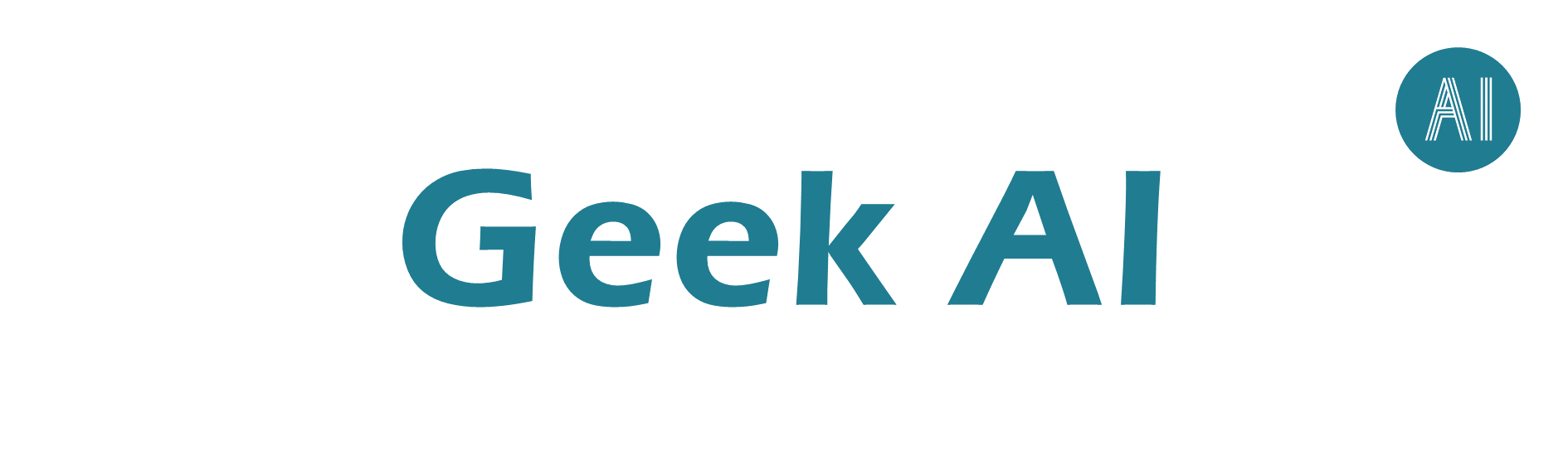
🤖 简单又便宜!所有人都能用上完整版 Stable Diffusion!
价格 | 简单 | 版本 | 便携 | 整体评价 | |
---|---|---|---|---|---|
Google colab | ⭐⭐⭐⭐⭐ | ⭐⭐ | ⭐⭐⭐⭐ | ⭐⭐⭐⭐⭐ | 价格相对较低,操作简单,但稳定性较差 |
大厂云服务 | ⭐⭐ | ⭐⭐ | ⭐⭐ | ⭐⭐⭐⭐⭐ | 价格较高,操作流程复杂,可能存在bug |
AI工具整合包 | ⭐⭐⭐ | ⭐⭐⭐⭐ | ⭐ | ⭐⭐⭐⭐⭐ | 价格适中,操作简单,但版本更新不及时 |
MAC 电脑本地安装 | ⭐⭐ | ⭐ | ⭐ | ⭐⭐⭐ | 成本高,操作复杂,效果不佳 |
PC 台式机本地安装 | ⭐⭐⭐ | ⭐⭐⭐ | ⭐⭐⭐⭐ | ⭐ | 成本高,操作复杂,便携性差 |
苹果设备、电脑性能受限或者预算有限,可以使用完整版 Stable Diffusion 么?完全可以!作者 @逗砂 分享了自己尝试且放弃过的多个方案,并最终找到了最满意的工具 —— 揽睿星舟!
0. 网址 :https://www.lanrui-ai.com/
1. 价格:一块 3090的每小时算力价格只要1.9元,这是目前看到的国内最低价格
2. 简单:官方针对 Stable Diffusion 做了快捷版应用,直接点击安装就可以使用,更新也比较及时
3. 版本:目前看到的唯一一个能够用非本地安装的方式看到最新版本的controlnet的工具,虽然目前只在基于工作空间的方式可以使用 ⋙ 使用教程
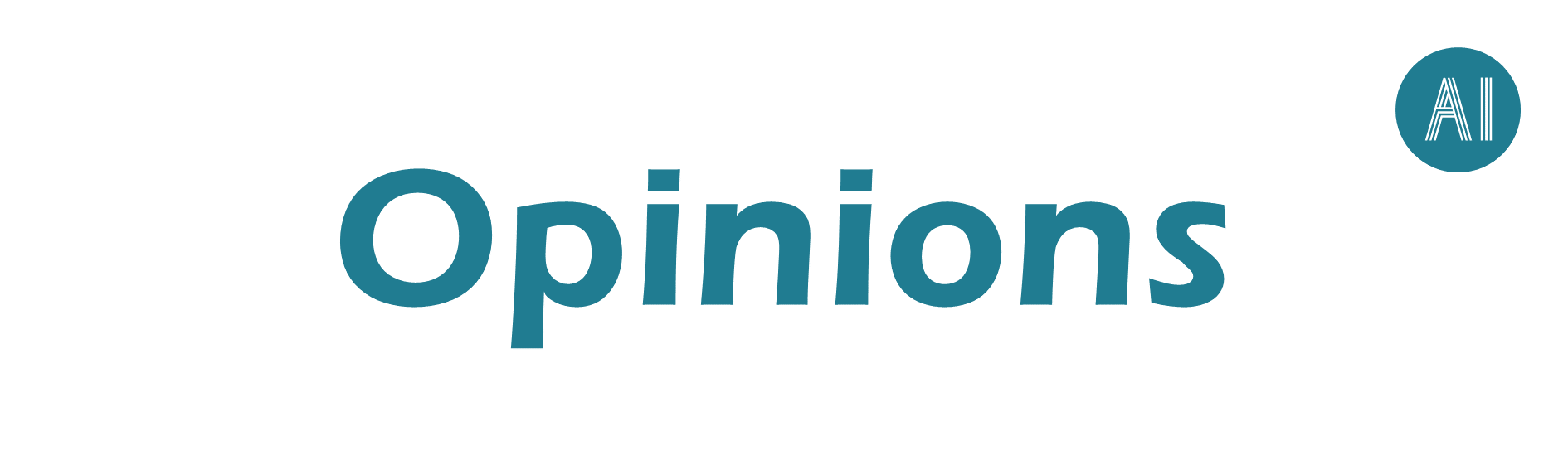
🤖 红杉美国最新观察:新的语言模型技术栈,企业如何将AI应用落地
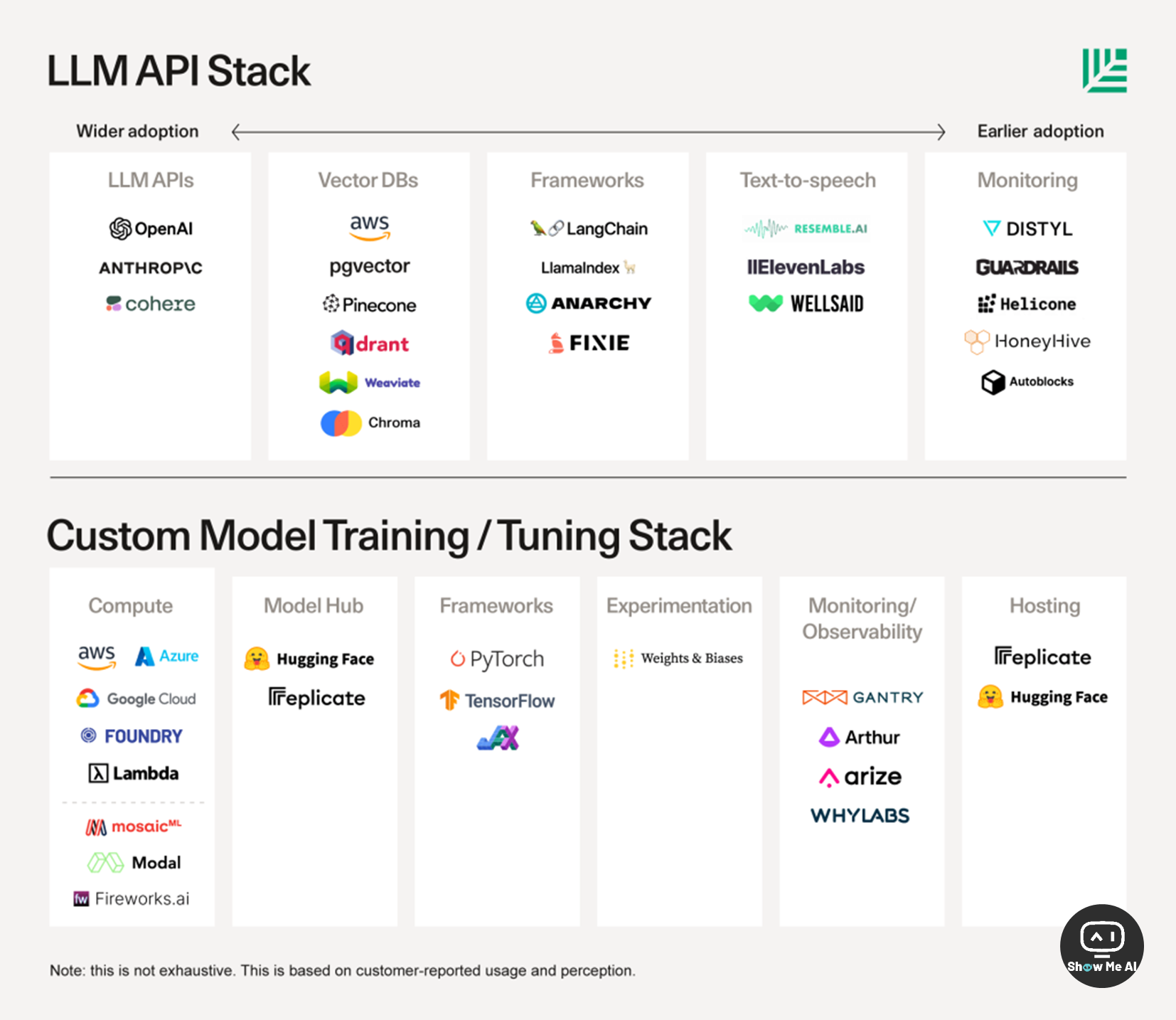
6月14日,红杉美国在官网发布了一篇文章「The New Language Model Stack (全新的语言模型技术栈) 」,是投资组合中33家公司的访谈总结,共8个重要结论,总结最新的发展现状并对未来进行了预测。
需要说明的是,这33家公司覆盖了从种子轮到上市各个阶段,因此本文对AI创业者及从业者都会非常有帮助!
1. 几乎所有在红杉网络中的公司,都在将语言模型融入到他们的产品中
2. 这些应用程序的新技术栈主要集中在语言模型API、检索和编排上,但开源使用也在增长
3. 公司希望根据自身独特的背景定制语言模型
4. 今天,LLM API的技术栈与自定义模型训练的技术栈可能感觉是分开的,但随着时间的推移,它们会逐渐融合在一起
5. 技术栈对开发人员越来越友好
6. 语言模型需要变得更加可靠(输出质量、数据隐私、安全性),以便得到全面采用
7. 语言模型应用将会越来越广泛
8. 时间还早 ⋙ 原文(英语) | 中文翻译版
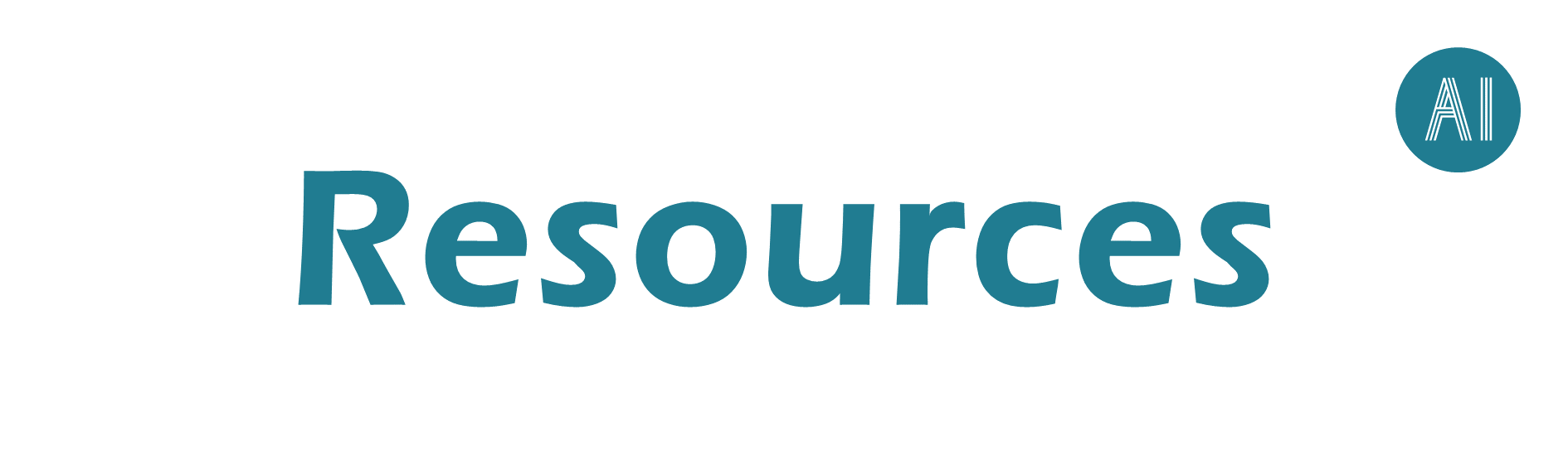
🤖 人人都能看懂的 ChatGPT 原理课
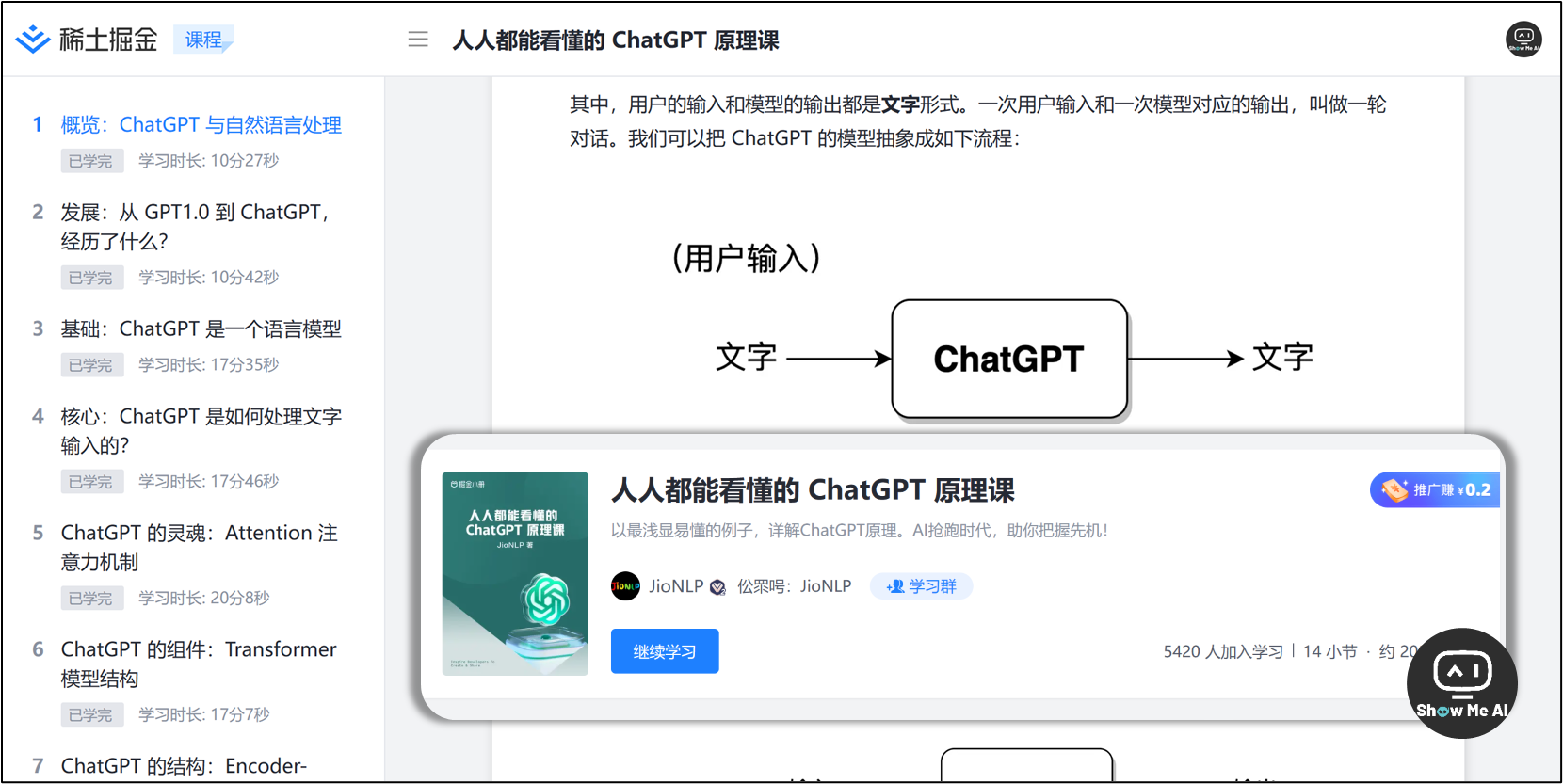
课程优惠价 0.6 元,使用课程简介中的优惠码可以0元购买。掘金疯了 😂
这是掘金社区推出的一门「免费」图文课程,以 最直观、最浅白 的方式,深入浅讲解 ChatGPT 的技术原理,揭开这个世界上目前为止最先进大模型技术的神秘面纱。
文章将重点内容用举例、类比等方式进行解释,帮助理解 ChatGPT 模型原理细节。非 AI 领域的读者可以从零开始了解掌握ChatGPT 模型原理,AI 领域从业人员可以快速温习、熟悉 ChatGPT 的原理与训练方式,深刻把握当前 AI 领域模型训练范式的变化。
1. 概览:ChatGPT 与自然语言处理
2. 发展:从 GPT1. 0 到 ChatGPT,经历了什么?
3. 基础:ChatGPT 是一个语言模型
4. 核心:ChatGPT 是如何处理文字输入的?
5. ChatGPT 的灵魂:Attention 注意力机制
6. ChatGPT 的组件:Transformer 模型结构
7. ChatGPT 的结构:Encoder-Decoder
8. 模型训练基础:监督学习与 ChatGPT 预训练
9. 模型训练基础:GPT 中的 few-shot 小样本学习
10. 模型训练基础:什么是强化学习?
11. 模型训练核心:ChatGPT 中的 RLHF 人工反馈强化学习模式
12. 模型训练核心:GPT 系列模型所依赖的数据
13. ChatGPT 的优缺点及影响
14. ChatGPT 吹响了第四次产业革命 (AI 革命) 的号角 ⋙ 点击学习完整内容
感谢贡献一手资讯、资料与使用体验的 ShowMeAI 社区同学们!
◉ 点击 👀日报&周刊合集,订阅话题 #ShowMeAI日报,一览AI领域发展前沿,抓住最新发展机会!
◉ 点击 🎡生产力工具与行业应用大全,一起在信息浪潮里扑腾起来吧!