使用GraphQL构建现代API
- GraphQL简介
- 安装GraphQL
- 使用npm安装GraphQL
- 使用Yarn安装GraphQL
- 创建GraphQL服务器
- 定义Schema
- 编写Resolver
- 查询数据
- 变更数据
- 使用Apollo Client
- GraphQL订阅
- 数据验证
- 错误处理
- 分页查询
- 拆分和组合Schema
- 总结
随着API的发展,传统的RESTful API逐渐显露出其局限性,特别是在需要灵活的数据查询和减少网络往返次数的应用场景中。GraphQL作为一种新兴的数据查询和操作语言,正好弥补了这一缺陷。本文将详细介绍如何使用GraphQL来构建现代API。
GraphQL是一种用于API的查询语言,它提供了一种更有效的方式去获取数据。 GraphQL通常通过GraphQL服务器和客户端库来使用。 如果项目使用Node.js,可以使用npm安装GraphQL。npm install graphql graphql-yoga
如果项目使用Yarn,可以使用Yarn安装GraphQL。
yarn add graphql graphql-yoga
使用graphql-yoga快速创建一个GraphQL服务器。
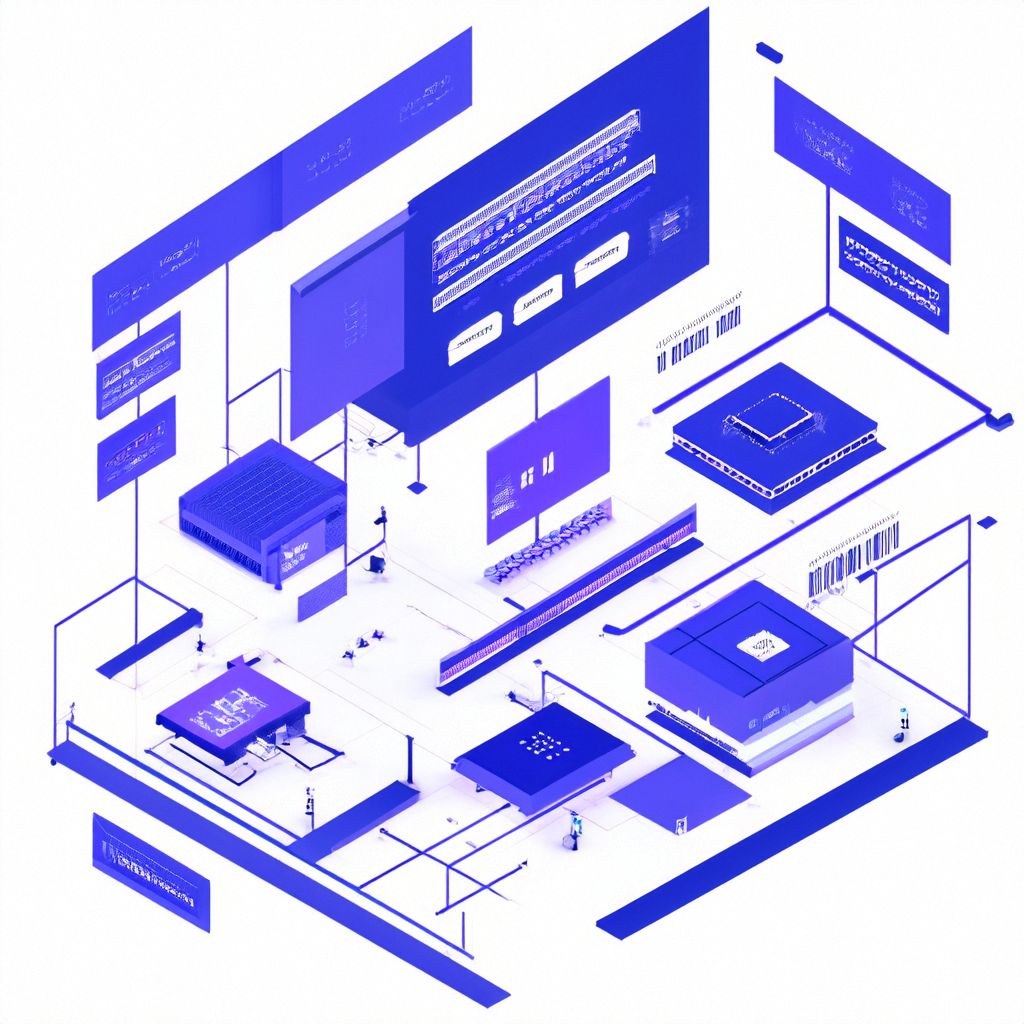
const { GraphQLServer } = require('graphql-yoga');const resolvers = {Query: {hello: () => 'Hello World!',},
};const server = new GraphQLServer({ typeDefs, resolvers });
server.start(() => console.log('Server running on http://localhost:4000'));
在GraphQL中,Schema定义了数据的结构和可用的操作。
type Query {hello: String
}
Resolver负责执行具体的操作并返回数据。
const resolvers = {Query: {hello: () => 'Hello World!',},
};
在GraphQL中,可以通过简单的查询语句来获取数据。
query {hello
}
除了查询之外,GraphQL还支持变更数据的操作。
schema {query: Querymutation: Mutation
}... existing schema ...type Mutation {incrementCount: Int!
}
const resolvers = {... existing resolvers ...Mutation: {incrementCount: (parent, args, context, info) => {context.count++;return context.count;}}
};
mutation {incrementCount
}
Apollo Client是一个完整的状态管理库,专门为GraphQL打造。
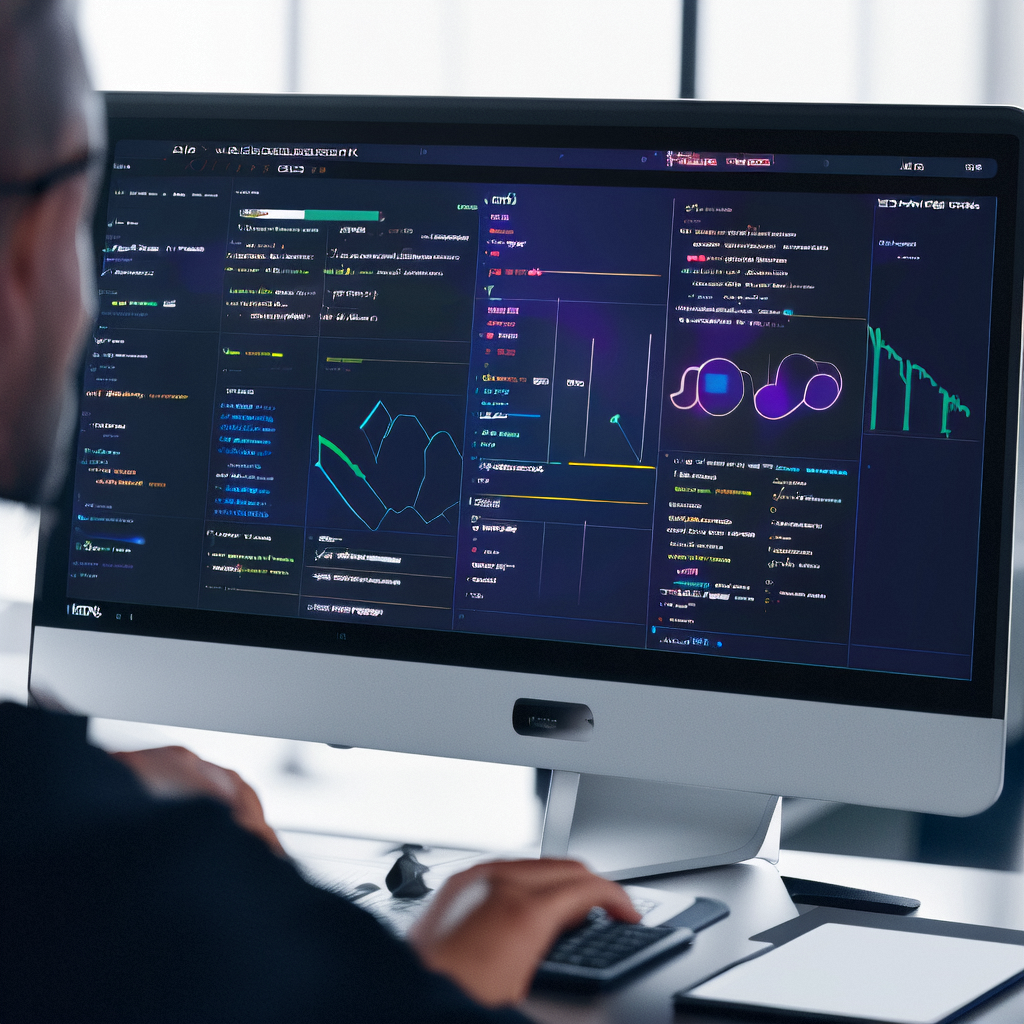
npm install @apollo/client
import { ApolloClient, InMemoryCache, gql } from '@apollo/client';const client = new ApolloClient({uri: 'http://localhost:4000/',cache: new InMemoryCache(),
});client.query({query: gql`{hello}`,
}).then(console.log);
GraphQL不仅支持查询和变更数据,还支持订阅数据的变化。
subscription {countUpdated @subscription
}
const resolvers = {Subscription: {countUpdated: {subscribe: (parent, args, { pubsub }) => {return pubsub.asyncIterator('COUNT_UPDATED');}}}
};
const pubsub = new PubSub();// Inside resolver that modifies the count
pubsub.publish('COUNT_UPDATED', { countUpdated: context.count });
GraphQL提供了强大的类型系统来帮助开发者定义和验证数据。
type User {id: ID!name: String!email: String!
}
const resolvers = {Query: {user: () => ({ id: '1', name: 'Alice', email: 'alice@example.com' }),},
};
GraphQL服务器应该能够处理错误并将其返回给客户端。
const resolvers = {Query: {user: () => {throw new Error('User not found');},},
};
query {user {idnameemail}
}
GraphQL可以轻松地实现分页查询。
type Query {users(first: Int, after: String): UsersConnection
}union UsersConnection = UsersPagetype UsersPage {edges: [UserEdge]!pageInfo: PageInfo!
}type UserEdge {node: User!cursor: String!
}type PageInfo {hasNextPage: Boolean!endCursor: String
}
const resolvers = {Query: {users: (parent, { first, after }) => {const users = [{ id: '1', name: 'Alice', email: 'alice@example.com' }, { id: '2', name: 'Bob', email: 'bob@example.com' }];const pageOfUsers = users.slice(0, first);const pageInfo = {hasNextPage: pageOfUsers.length < users.length,endCursor: pageOfUsers[pageOfUsers.length - 1].id,};return {edges: pageOfUsers.map(user => ({ node: user, cursor: user.id })),pageInfo,};},},
};
在大型应用中,可以将Schema拆分成多个文件,然后组合在一起。
import { makeExecutableSchema } from '@graphql-tools/schema';
import { loadFilesSync } from '@graphql-tools/load-files';
import path from 'path';const typeDefsArray = loadFilesSync(path.join(__dirname, './schema/**/*.graphql'));
const resolversArray = loadFilesSync(path.join(__dirname, './resolvers/**/*.js'));const schema = makeExecutableSchema({typeDefs: typeDefsArray,resolvers: resolversArray,
});
通过本文,你已经学习了如何使用GraphQL来构建现代API。我们介绍了GraphQL的基本概念、安装方法、创建GraphQL服务器、定义Schema、编写Resolver、查询数据、变更数据、使用Apollo Client、GraphQL订阅、数据验证、错误处理、分页查询、拆分和组合Schema等内容。掌握了这些知识,将有助于你在实际工作中更好地利用GraphQL来构建高效、灵活的API。
使用GraphQL可以让你构建出更加高效和灵活的API。