题目: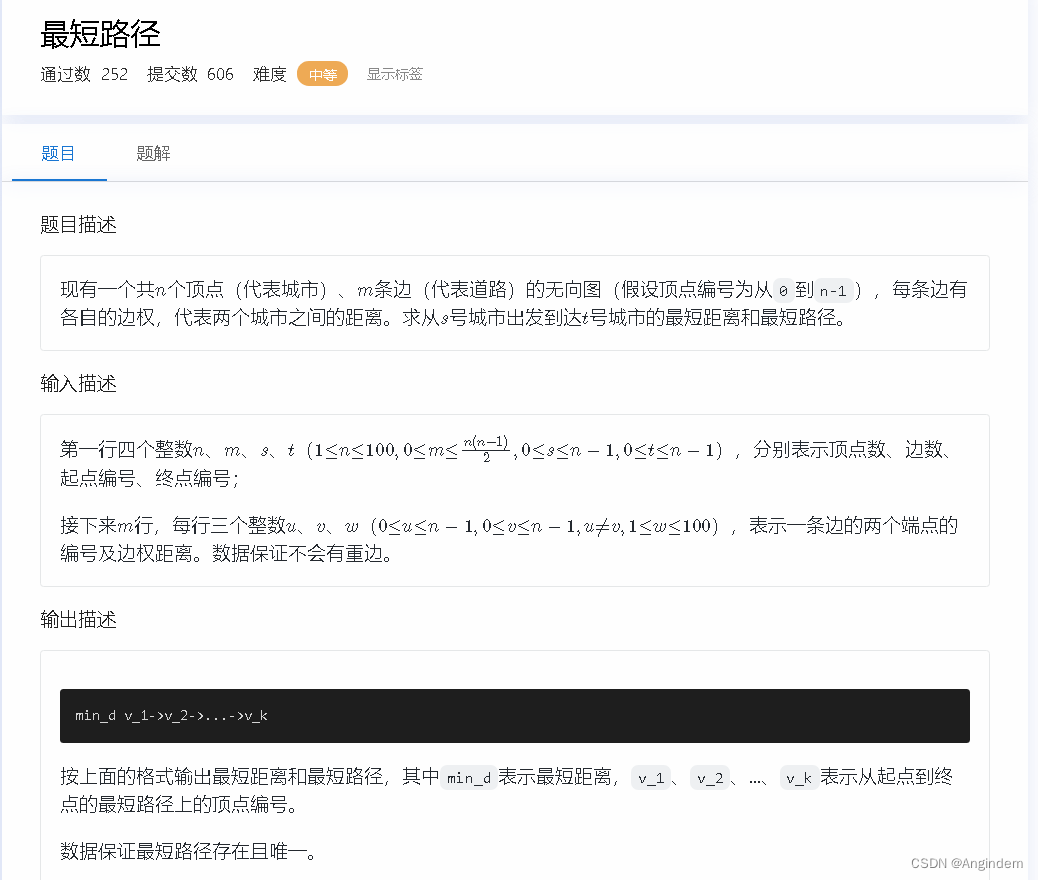
样例:
|
3 0->3->2 |
思路:
根据题目意思,求最短路,这个根据平时的Dijkstra(堆优化)即可,关键在于求路径的方法,求路径的方法有很多种,其中最经典的就是通过 DFS递归求路径,其中我之前做的笔记 BFS求路径 便是用到了该方法。
1、初始化记录数组为某一个特定值,比如初始化为 -1,方便递归求路径边界; 2、记录当前结点是由哪上一个结点走动的; 3、DFS递归求路径函数,由于是当前结点记录上一个结点,所以递归由终点开始,到起点结束。 |
代码详解如下:
#include <iostream>
#include <cstring>
#include <algorithm>
#include <queue>
#include <unordered_map>
#define endl '\n'
#define x first
#define y second
#define mk make_pair
#define int long long
#define NO puts("NO")
#define YES puts("YES")
#define umap unordered_map
#define INF 0x3f3f3f3f3f3f3f3f
#define All(x) (x).begin(),(x).end()
#pragma GCC optimize(3,"Ofast","inline")
#define ___G std::ios::sync_with_stdio(false),cin.tie(0), cout.tie(0)
using namespace std;
const int N = 2e6 + 10;
using PII = pair<int,int>;int n,m,start,last;int dist[N]; // 记录最短路距离
int tree[N]; // 记录路径
bool st[N]; // 标记走动结点vector<int>path; // 存储路径结点// 建立链表
int h[N],e[N],w[N],ne[N],idx;
inline void Add(int a,int b,int c)
{e[idx] = b,w[idx] = c,ne[idx] = h[a],h[a] = idx++;
}inline void Dijkstra()
{// 初始化记录路径数组memset(tree,-1,sizeof tree);// 初始化最短距离memset(dist,INF,sizeof dist);dist[start] = 0;// 建立堆priority_queue<PII,vector<PII>,greater<PII>>q;// 存储起点和最短距离的对组q.push(mk(0,start));// 开始堆排序的求值while(q.size()){// 获取当前结点的对组PII now = q.top();q.pop();int a = now.y; // 获取当前结点int dis = now.x;// 获取当前结点相关的最短距离// 如果当前结点走动过,进入下一个结点的最短距离更新if(st[a]) continue; st[a] = true; // 标记当前结点for(int i = h[a];i != -1;i = ne[i]){// 获取相关结点int j = e[i];// 更新最短距离if(dist[j] > dis + w[i]){dist[j] = dis + w[i];tree[j] = a; // 记录当前结点 j 由 a 走动得来的}// 存储当前结点 j 和相关最短距离 的对组q.push(mk(dist[j],j));}}return ;
}void getPath(int now)
{// 如果当前状态到达了边界起点// 那么开始回溯递归取路径if(now == start){path.emplace_back(now);return ;}// 递归上一个结点getPath(tree[now]);// 回溯回来,取当前结点path.emplace_back(now);
}inline void solve()
{// 初始化链表memset(h,-1,sizeof h);cin >> n >> m >> start >> last;while(m--){int a,b,c;cin >> a >> b >> c;// 由于是无向图,添加相连两个结点的链表Add(a,b,c);Add(b,a,c);}// Dijkstra 求值Dijkstra();// 根据终点递归获取路径getPath(last);// 输出最短距离cout << dist[last] << ' ';// 输出最短路径bool rem = false; // 控制输出格式for(int i : path){if(rem) cout << "->";cout << i;rem = true;}return ;
}signed main()
{
// freopen("a.txt", "r", stdin);___G;int _t = 1;
// cin >> _t;while (_t--){solve();}return 0;
}