大家好,我是小锋我们继续来学习链表。
我们在上一节已经把链表中的单链表讲解完了,大家感觉怎么样我们今天来带大家做一些练习帮助大家巩固所学内容。
1. 删除链表中等于给定值 val 的所有结点
. - 力扣(LeetCode)
我们大家来分析一下这个题,我们能想到的思路有两种,1,删除 2,插入
第一种,我们直接一个一个找当找到val我们就删除,然后继续重复下去。
第二种,我们创建一个新的头节点并沿着原节点一个一个走下去如果不是val我们就在新的头节点尾插。
第一种
#include<stdlib.h>struct ListNode* removeElements(struct ListNode* head, int val) {struct ListNode* ps = head;while (ps){if (ps->val == val){head = head->next;ps->next = NULL;ps = head;}else{struct ListNode* pt = ps->next;if (pt) {if (pt->val == val){ps->next = pt->next;pt->next = NULL;}else{ps = ps->next;}}else {ps = ps->next;}}}return head;}
第二种
#include<stdlib.h>struct ListNode* removeElements(struct ListNode* head, int val) {struct ListNode* pt=NULL;struct ListNode* ps=NULL;struct ListNode* cur=head;while(cur){if(cur->val==val){cur=cur->next;}else{if(ps){pt->next=cur;cur=cur->next;pt=pt->next;pt->next=NULL;}else{ps=cur;cur=cur->next;ps->next=NULL;pt=ps;}}}return ps;}
反转一个单链表
. - 力扣(LeetCode)
我们大家来分析这道题,有很多种思路这里我向大家推荐两种
1,我们将链表的朝向改变让最后一个节点变成表头,然后依次改变每个节点的指向就完成了反转
2,我们找到最后一个节点然后依次将每个节点尾插。
第一种
# include<stdio.h>
struct ListNode* reverseList(struct ListNode* head) {struct ListNode*ps=head;if(ps){struct ListNode*pt=ps->next;while(pt){struct ListNode*cur=pt->next;if(ps==head){pt->next=ps;ps->next=NULL;ps=pt;pt=cur;}else{pt->next=ps;ps=pt;pt=cur;}}}else{return NULL;}return ps;
}
第二种
# include<stdio.h>
struct ListNode* reverseList(struct ListNode* head) {struct ListNode*ps=NULL;struct ListNode*cur=head;struct ListNode*pt=NULL;struct ListNode*tall=NULL;if(cur){while(cur){if(cur->next){while(cur->next->next){cur=cur->next;}tall=cur;cur=cur->next;tall->next=NULL;}else{head=NULL;}if(ps){pt->next=cur;pt=pt->next;}else{ps=cur;pt=ps;}cur=head;}}else{return NULL;}return ps;
}
返回链表的中间结点
. - 力扣(LeetCode)
这一道题的思路也有很多,我们这里主要用快慢指针的思路来解决
我们创建两个指针,都指向头节点,然后一个指针一次走两步,一个指针一次走一步当快的指针走到最后一个节点是慢的指针刚好走到中间节点。
struct ListNode* middleNode(struct ListNode* head) {struct ListNode*quick=head;struct ListNode*slow=head;if(head){while(quick&&quick->next){quick=quick->next->next;slow=slow->next; }}else{return NULL;}return slow;
}
输入一个链表,输出该链表中倒数第k个结点
这道题的思路在头节点定义两个指针先让一个指针先走k个然后再一起走当其中一个指针走到链表末尾时另一个指针就在倒数第k个。
typedef struct ListNode ListNode;struct ListNode {int val;struct ListNode *next;};struct ListNode* middleNode(int k, struct ListNode* head) {struct ListNode* ps = head;struct ListNode* pt = head;if (head) {while (k) {if (ps) {ps = ps->next;}else {break;}k--;}while (ps) {ps = ps->next;pt = pt->next;}}else {return NULL;}if (k<=0) {return pt;}else {return NULL;}}
下面这个是测试函数
int main() {ListNode* n1 = (ListNode*)malloc(sizeof(ListNode));assert(n1);ListNode* n2 = (ListNode*)malloc(sizeof(ListNode));assert(n2);ListNode* n3 = (ListNode*)malloc(sizeof(ListNode));assert(n3);ListNode* n4 = (ListNode*)malloc(sizeof(ListNode));assert(n4);ListNode* n5 = (ListNode*)malloc(sizeof(ListNode));assert(n5);n1->val = 1;n2->val = 2;n3->val = 3;n4->val = 4;n5->val = 5;n1->next = n2;n2->next = n3;n3->next = n4;n4->next = n5;n5->next = NULL;ListNode* add= middleNode(6,NULL);if (add)printf("%d", add->val);elseprintf("NULL");return 0;
}
有序链表合并
. - 力扣(LeetCode)
这道题的解题思路
创建一个新的头节点然后建立两个指针分别指向两个升序链表对比两个节点的val,选小的尾插选中的那个节点的指针指向下一个节点,最后还没有走完的指针将全部节点都尾插。
struct ListNode* mergeTwoLists(struct ListNode* list1, struct ListNode* list2) {struct ListNode*ps=NULL;struct ListNode*cur=NULL;if(list1==NULL)return list2;if(list2==NULL)return list1;while(list1&&list2){if(list1->val>list2->val){if(ps){cur->next=list2;cur=cur->next;list2=list2->next;}else{ps=list2;cur=ps;list2=list2->next;}}else{if(ps){cur->next=list1;cur=cur->next;list1=list1->next;}else{ps=list1;cur=ps;list1=list1->next;}}}if(list1){cur->next=list1;}else{cur->next=list2;}return ps;
}
链表分割
这一道题我想的思路是创建一个头节点,把小于x的节点在原链表中删除后尾插该节点,然后在把该链表与原链表连接。
但是还有一种更好的方法,我们直接创建两个头节点分别尾插大于x和小于x的节点最后再连接。
无疑这一种方法更为简单。
我们来试试
/*
struct ListNode {int val;struct ListNode *next;ListNode(int x) : val(x), next(NULL) {}
};*/
class Partition {
public:ListNode* partition(ListNode* pHead, int x) {ListNode*ps,*pt,*psd,*ptd;psd=ps=(ListNode*)malloc(sizeof(int)+sizeof(ListNode*));ptd=pt=(ListNode*)malloc(sizeof(int)+sizeof(ListNode*));ListNode*cur=pHead;while(cur){if(cur->val<x){psd->next=cur;psd=cur;}else{ptd->next=cur;ptd=cur;}cur=cur->next;}psd->next=pt->next;ptd->next=NULL;pHead=ps->next;free(ps);free(pt);return pHead;}
};
链表的回文结构
这一道题我们可以找到链表的中间节点把中间节点后面的反转然后从头与尾向中间比较如果都一样那么就是回文结构了。
/*
struct ListNode {int val;struct ListNode *next;ListNode(int x) : val(x), next(NULL) {}
};*/
class PalindromeList {
public:bool chkPalindrome(ListNode* A) {ListNode*ps=A;ListNode*pt=A;while(ps&&ps->next){ps=ps->next->next;pt=pt->next;}ListNode*cur=pt;ListNode*list=cur->next;ListNode*add=list->next;while(list){list->next=cur;cur=list;list=add;if(add)add=add->next;}pt->next=NULL;ListNode*are=A;while(cur){if(are->val!=cur->val){return false;}else{are=are->next;cur=cur->next;}}return true;}
};
相交链表
这道题我们的思路是分别找出长的链表与短的链表节点个数然后用长的节点数减去短的节点数得到的数就是长的链表比短的链表多出的节点个数然后创建两个指针long,short,long先走多出的个数然后再一起走当long与short指向的next相等时就找到了相交节点。
struct ListNode *getIntersectionNode(struct ListNode *headA, struct ListNode *headB) {int a=0;int b=0;int n=0;struct ListNode *cut=headA;while(cut->next){a++;cut=cut->next;}cut=headB;while(cut->next){b++;cut=cut->next;}struct ListNode *longg=headA;struct ListNode *shortt=headB;if(a<b){n=b-a;while(n--){shortt=shortt->next;}}else{n=a-b;while(n--){longg=longg->next;}}while(longg&&shortt){if(longg==shortt){return longg;}else{longg=longg->next;shortt=shortt->next;}}return NULL;
}
判断环形链表
让我们来看看这道题,我的思路是快慢指针,类似追击问题,我们创建两个指针一个指针以一次两个节点的速度走下去,一个指针一次一个节点走下去,如果是有环的链表那么指针一定会相交,如果不是环形链表那么快的会遇到NULL。
bool hasCycle(struct ListNode *head) {struct ListNode *quick=head;struct ListNode *siow=head;while(quick&&quick->next){quick=quick->next->next;siow=siow->next;if(quick==siow){return true;}}return false;
}
找环形链表入口
先说一个结论:
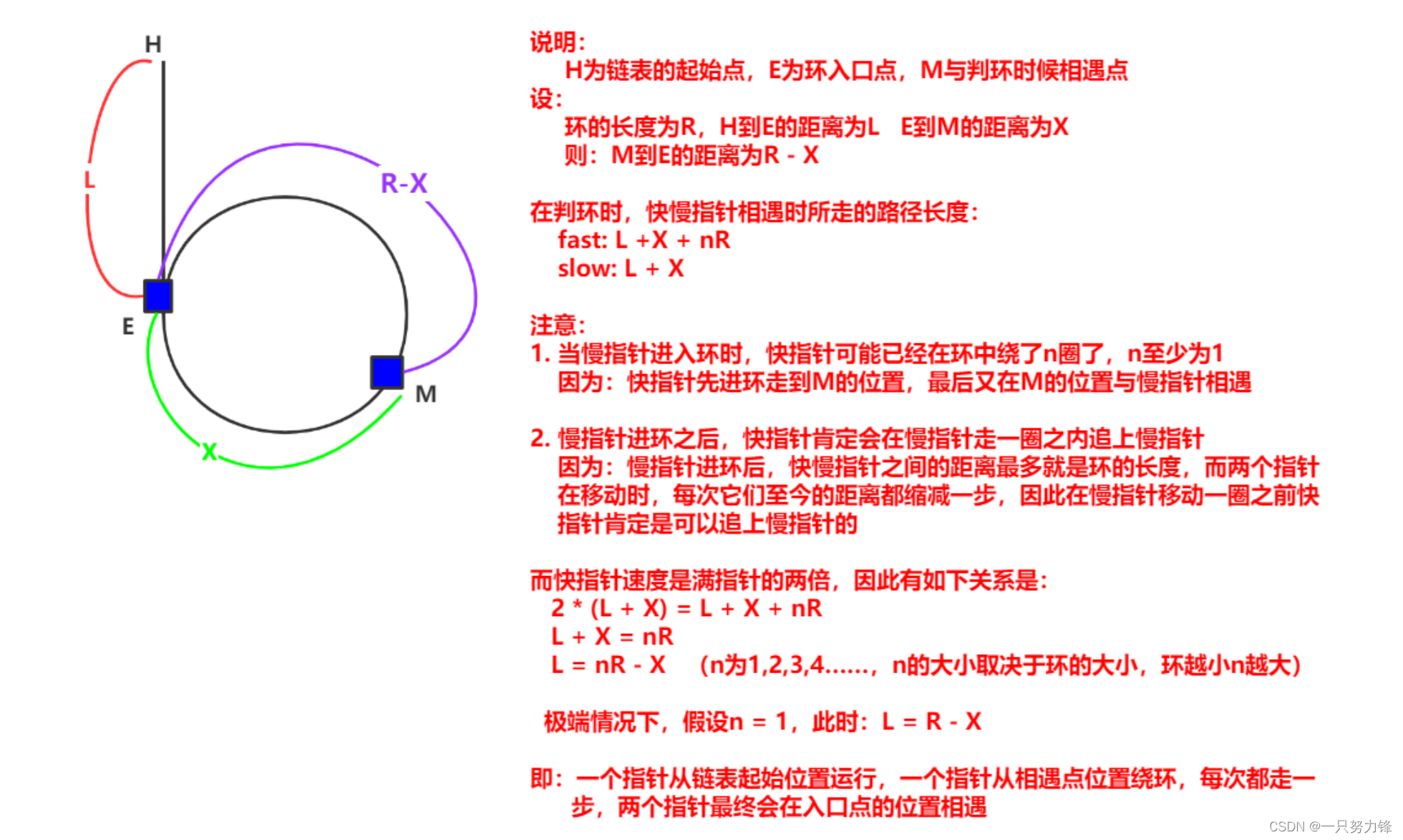
struct ListNode *detectCycle(struct ListNode *head) {struct ListNode *quick=head;struct ListNode *siow=head;while(quick&&quick->next){quick=quick->next->next;siow=siow->next;if(quick==siow){struct ListNode *ps=head;while(quick!=ps){quick=quick->next;ps=ps->next;}return ps;}}return NULL;
}
接下来大家思考几个问题
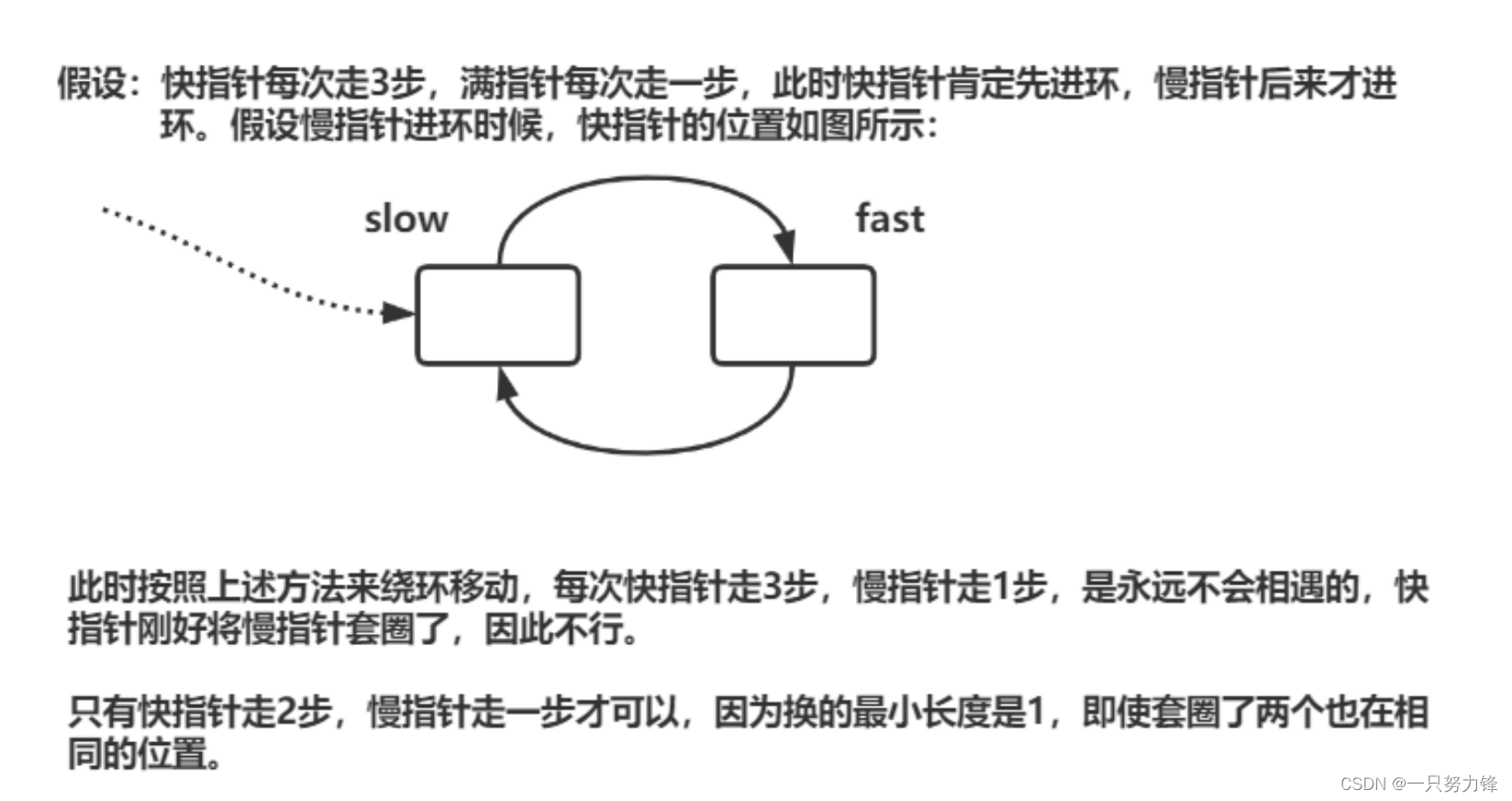
以上就是全部内容了,如果有错误或者不足的地方欢迎大家给予建议。