字节跳动有多少台服务器?
字节跳动大型的数据中心出口带宽是多少?
最近看到一个有意思的提问:抖音服务器带宽有多大,为什么能够供那么多人同时刷?今天来给大家科普一下。
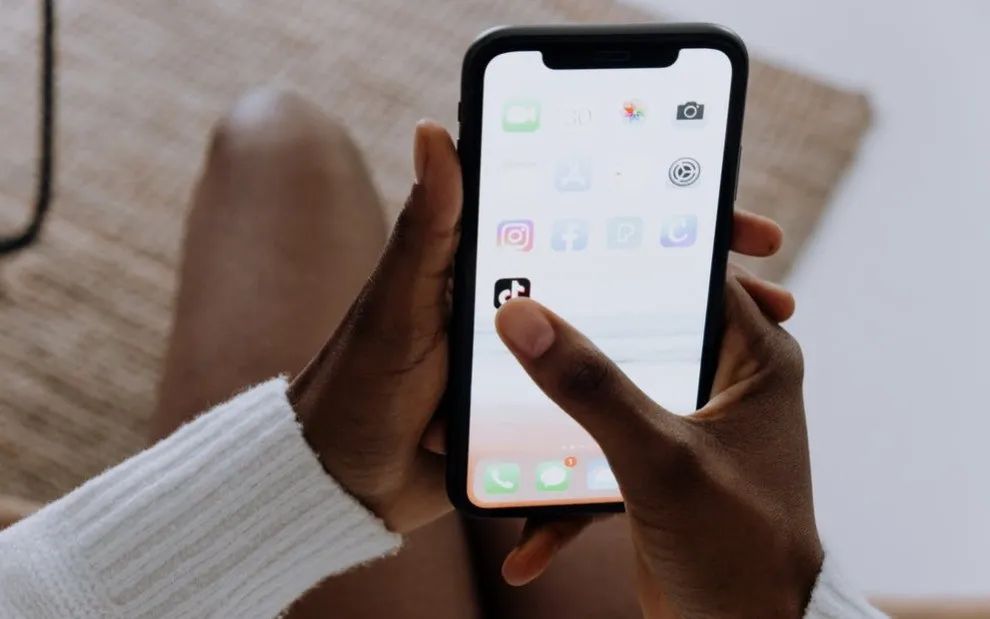
抖音,百度,阿里云,腾讯都是自建的数据中心,都是 T 级别出口带宽(总出口带宽),也就是达到 1T=1024G/s 的出口带宽,服务器总署基本都在 20 万台以上,甚至阿里云都超过了 100 万台。
字节跳动的数据中心总带宽,可能在 10TB 级别左右,预期突破 15TB 级别不远了。
一般情况下:总出口带宽 1TB,实际机房出口带宽可能只有 100G 上下,这是采用双(多)链路设计,双出口实现动态流量分担,总的出口带宽可以达到 T 级别。
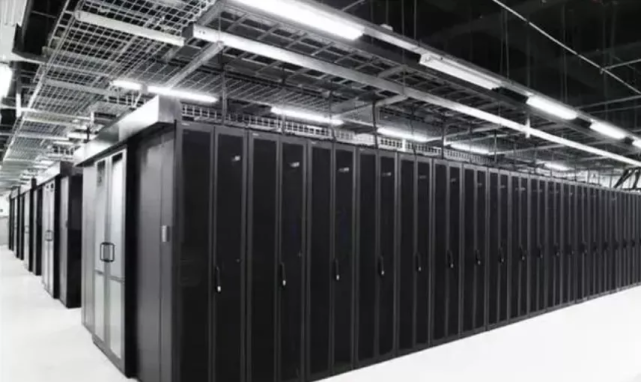
想要同一时间有数亿人在线,TB 级别带宽,CDN 加速和多节点,负载均衡等等技术缺一不可。(这个设计技术过于复杂,有相关专业朋友,可以评论简要概述)
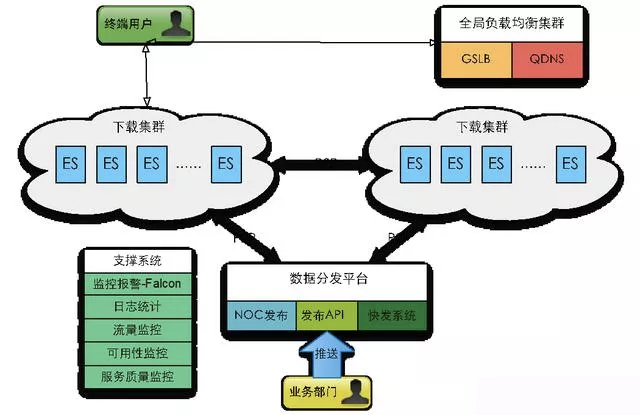
# 字节跳动有多少台服务器?
根据网络数据整理,2017 年 2-3 万台服务器,这个时候主要是租用服务器为主。
2018 年,字节跳动自己建设了数据中心,最大的数据中心在河北怀来官厅湖新媒体产业园,一期 5 万台服务器,二期 9 万台服务器。
2018 年,租用+自建的服务器数量达到 17 万台服务器。
2020 年,根据字节跳动招聘公告的数据,显示有 42 万台服务器。比 2018 年增长了 1.5 倍。(数据来自网络)
这部分服务器都是给中国区域使用,主要应用国内的抖音,西瓜视频,今日头条,飞书等产品。
在美国的 TIKTOK 是独立出来的运营,数据在美国当地存储和分发。2020 年 Tiktok 在美国也租用了近 10 万台服务器
据 Business Insider 公布数据,2020 年上半年,字节跳动在美国弗吉尼亚州北部租用了能耗达 53 兆瓦的数据中心。可以容纳数十万台服务器,占地面积可达数十万平方英尺。
Tiktok在印度,新加坡都在投资建设数据中心。
# 字节跳动大型的数据中心出口带宽是多少?
聊完了服务器数量,那么咱们来点硬核的东西:字节跳动大型的数据中心出口带宽是多少?
知识点:所谓的出口带宽,其实就是咱们普通人所说的下载带宽。就是服务器给每一个手机分发数据总速度。
一般情况下,小型的 IDC 公司自建机房,比如一些网站公司,租用联通,移动,电信的机房,可能总体出口带宽只有 5G。超过 30G 那都是具备一定规模的企业。网络公司营收少说也是几千万的企业。
所以,经常能够看到,一些规模还不错的企业,基本上都不再自建机房,都是使用云主机。例如阿里云的 ECS,腾讯云,百度云,AWS(亚马逊)。
一般一个企业网站(企业官网),20M 带宽,4G 内存,100G 硬盘,一年价格也就 4000-5000 块钱就足够了,赶上做活动价格可能更便宜。
这里面就是带宽最贵,当然增加带宽,达到一定等级,例如访问量增大,必须要增加内存和硬盘。
相比来说,带宽增加的话,费用更贵一些。这里就跟你说明一下:带宽比较昂贵,属于稀缺资源。
我们来看中国移动的一个机房,中国移动(河北石家庄)数据中心的数据:占地面积 174 亩,总建筑面积 13 万平方米,规划 10 栋单体建筑,全部建成后可提供约 3 万个机架的装机能力。
3 个 IDC 机房共可提供 3.1 万架机柜,15T 带宽资源。一个机柜,全 1U 设备部署数量一般不超过 16 台,全 2U 设备一般不超过 12 台,全 4U 设备一般 4 到 7 台。
我们取高性能的 2U 和 4U 服务器进行平均折中,各算一半(毕竟移动也算是有钱的大户,不能买低端的 1U 设备)。
那么 3.1 万架机柜就可以安装,最多 21-36 万台服务器。这里粗略取一个平均值:30 万台服务器。
享受 15T 的出口带宽资源。当然作为电信的干路网,移动拿带宽资源肯定是要比字节跳动更有优势的。
所以,我们粗略地估计字节跳动自建的 17 万台服务器的数据中心。总出口带宽可能在 7Tb-10TB 上下。
基本上肯定会采用双出口流量设计,再加上多链路的部署方式:可以做到实际出口带宽在 800G-1TG 就可以实现 10T 左右的总出口带宽。
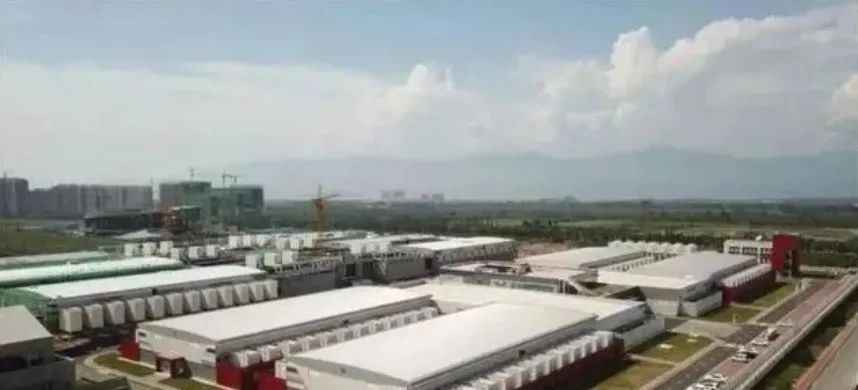
T 级别出口带宽是什么概念,如果我告诉你 2009 年,整个上海的出口带宽才 1140G,也就是刚刚达到 1TB。
在短短的 10 年后,一个企业的数据中心的出口带宽就超过 1TB,这个速度真的不可想象。
要知道 2009 年,虽然智能手机不发达,但是个人 PC 销量已经非常庞大了。
CDN 加速,让大众刷抖音,看视频都不再卡。
知识点:CDN(Content Delivery Network,内容分发网络)。
将服务端的内容发布到最接近用户的边缘节点,使用户可以就近取得所需的内容。
解决 Internet 网络拥塞状况,提高用户访问网站的响应速度。多种加速的方案集合。
用通俗的话解释 CDN 就是: 会把一些页面,专门压缩,有的压缩为静态页面,静态页面直接分发速度快。用户可以在 2s 内看到内容,体验感更好。【这是静态传输】
对于动态视频,首先通过智能路由,寻找最佳路径,然后协议优化将长连接,内容进行压缩,去除冗余。【这就是动态压缩】
给你们看一下 2015 年腾讯 5 亿日活,集合了音乐,即时通讯等等产品的 CDN 的级别,达到了 10TB 带宽。每天请求万亿次。
因此,我这里说字节跳动整体服务器有 10TB 应该只少不多。毕竟抖音日活有 6 亿,西瓜视频+今日头条我们粗略算是 2 亿,总计有 8 亿的日活。
就是这么大的带宽和技术实力,才能让我们看视频这么顺畅。
扫码关注
往
期
推
荐
1、Java网络编程之UDP和TCP套接字2、一天吃透计算机网络八股文3、IDE装上ChatGPT,彻底炸裂!4、求求你们了,别再重复造轮子了,一个 Spring 注解轻松搞定循环重试功能!5、全球最大ChatGPT开源平替来了!支持35种语言,写代码、讲笑话全拿捏6、一觉睡醒,ChatGPT 竟然被淘汰了?