科研论文期刊封面图、摘要图、图文摘要(Graphical Abstract)、TOC图(Table of Contents)、插图、配图、原理图、示意图、机制图、数据图等的设计和绘制,将科研学者的idea、概念、原理等以图表的形式展现出来,将艺术审美与严谨的科研相结合。
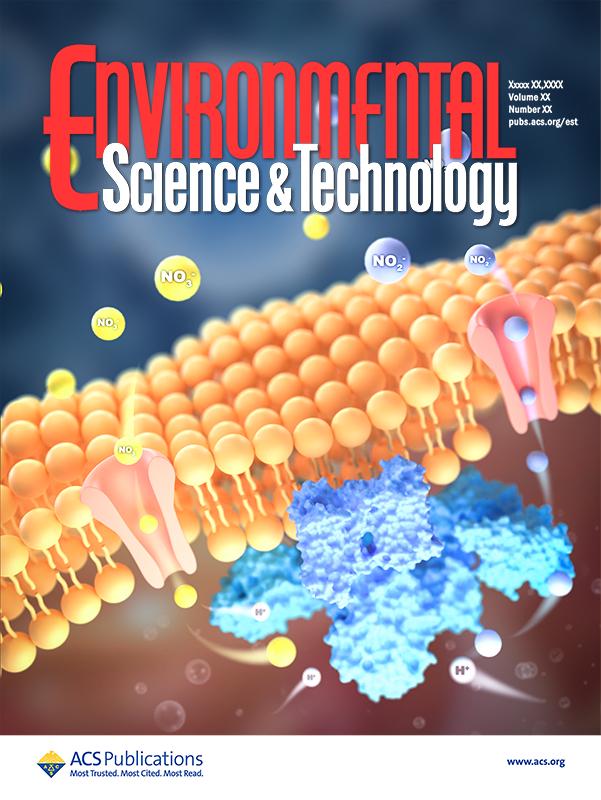
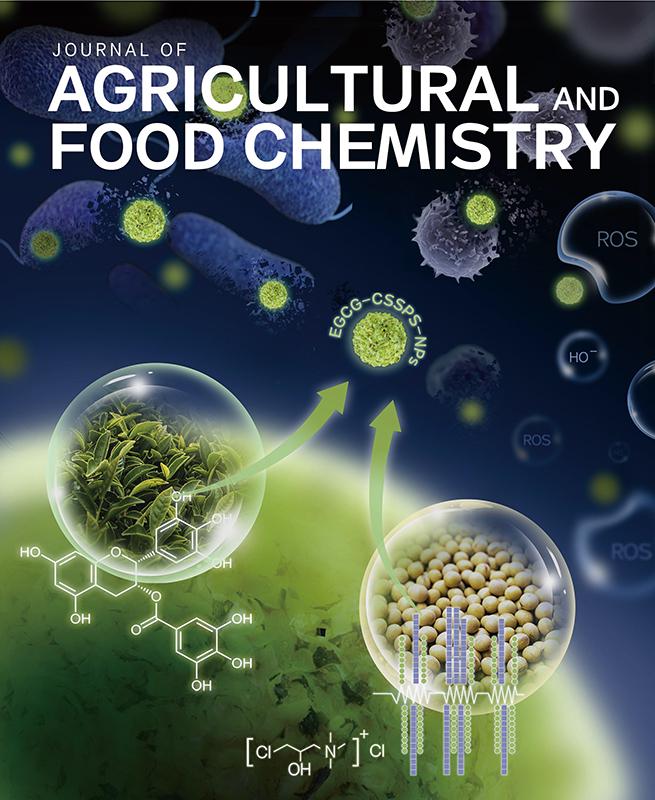
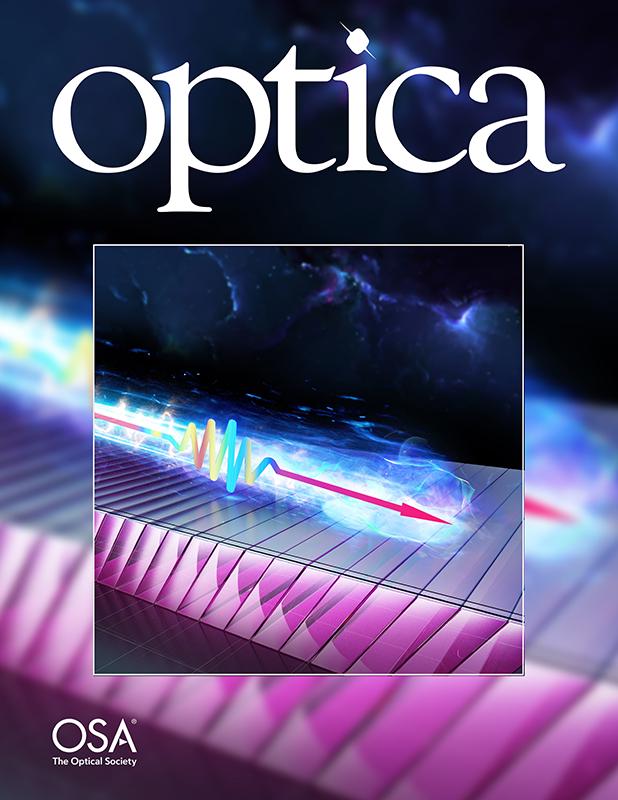
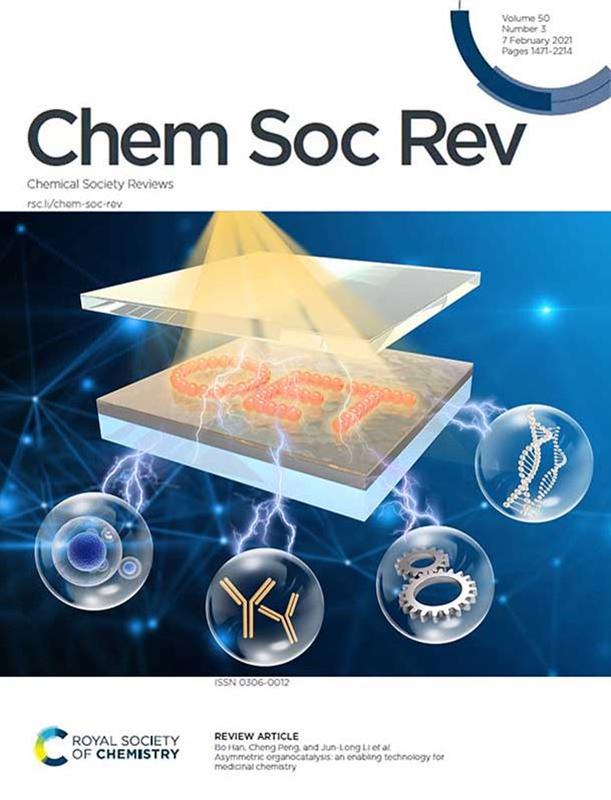
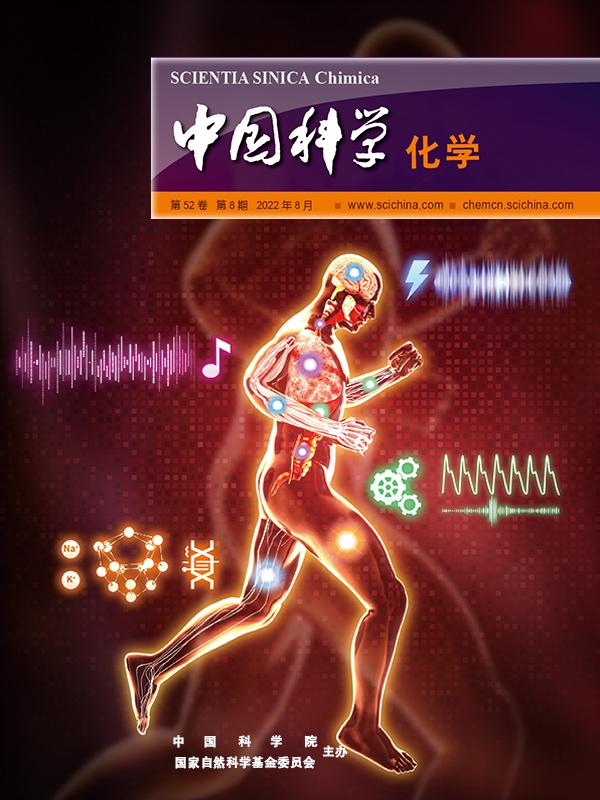
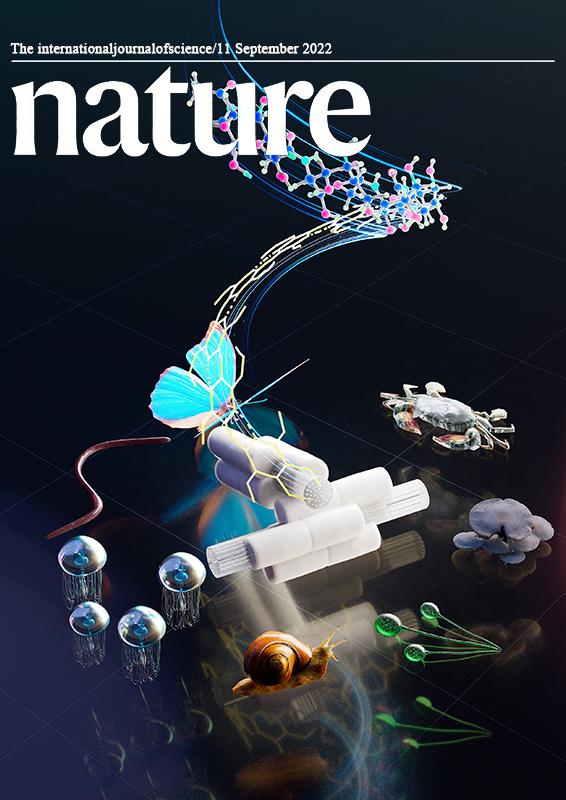
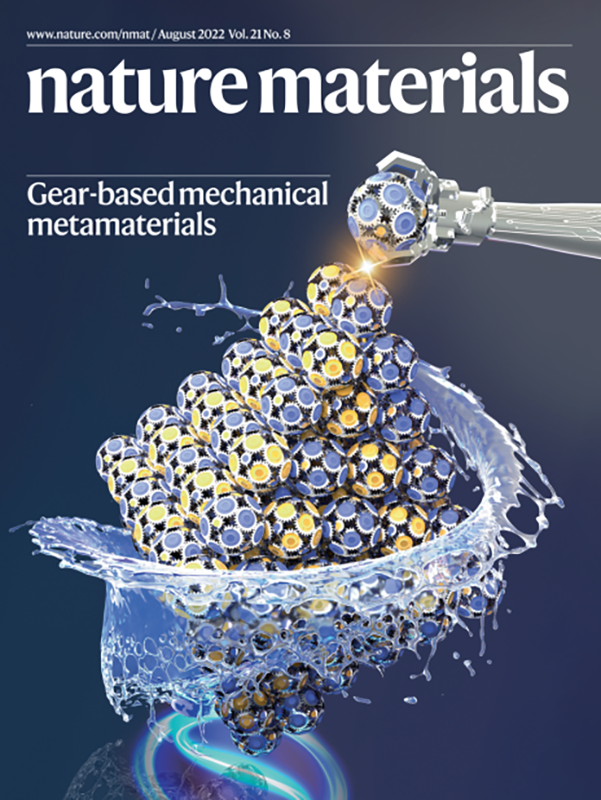
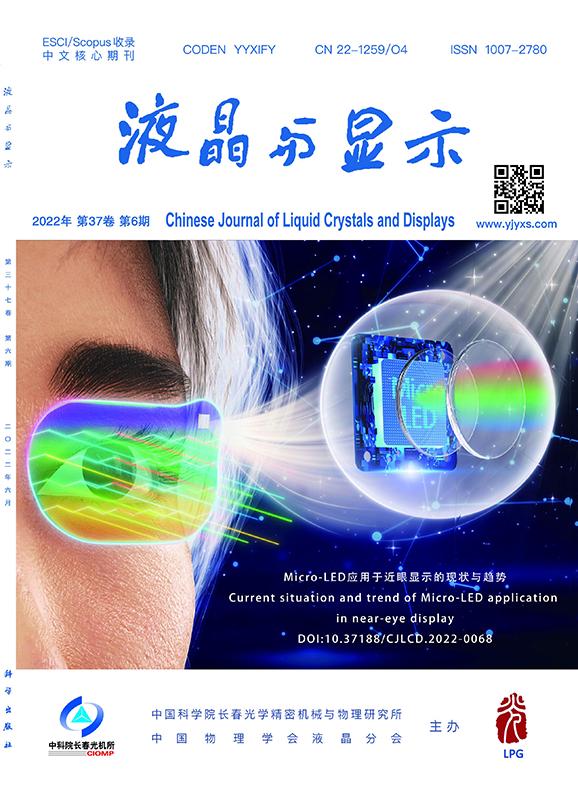
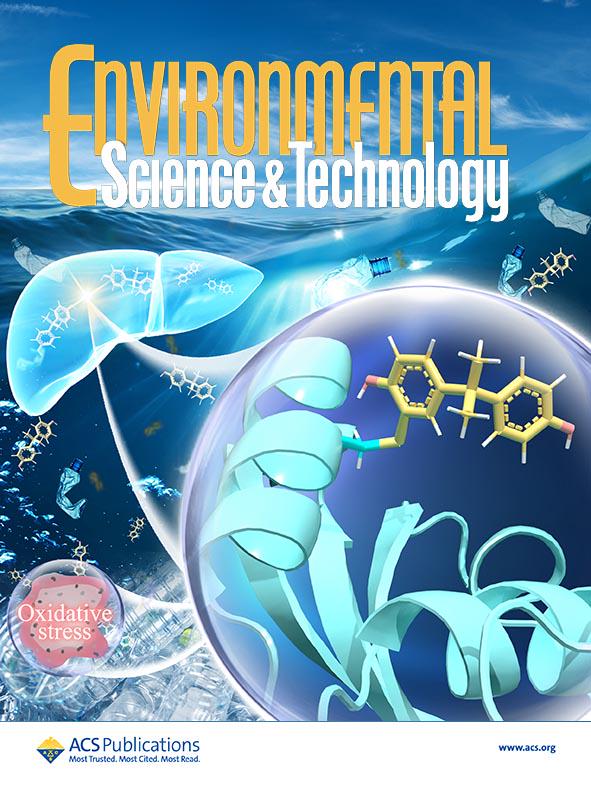
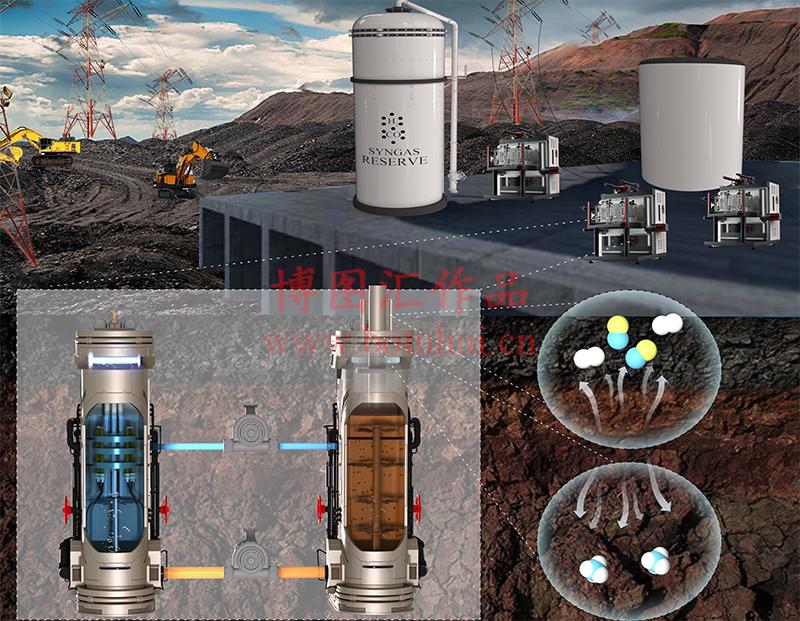
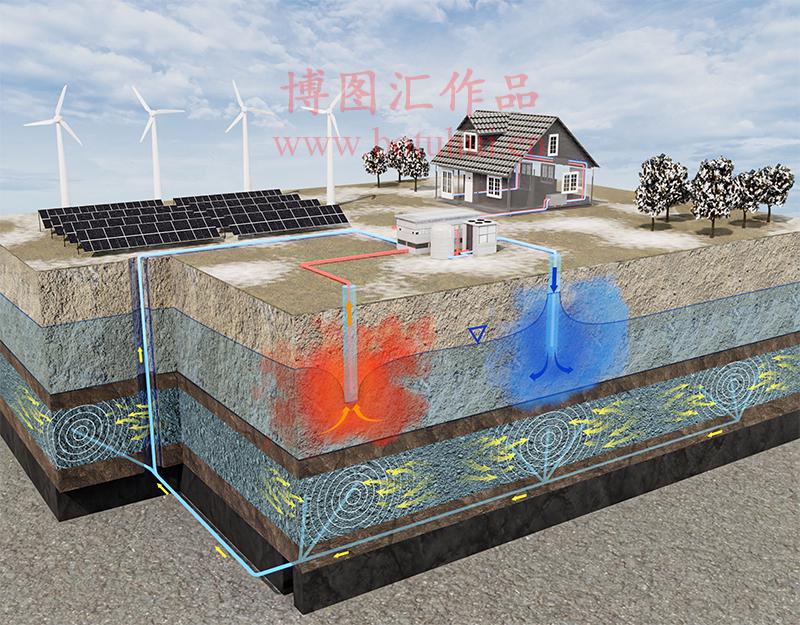
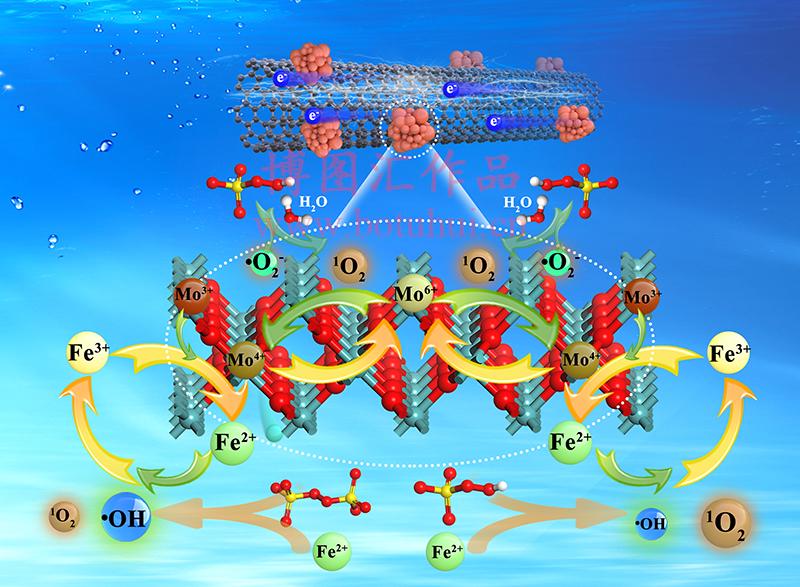
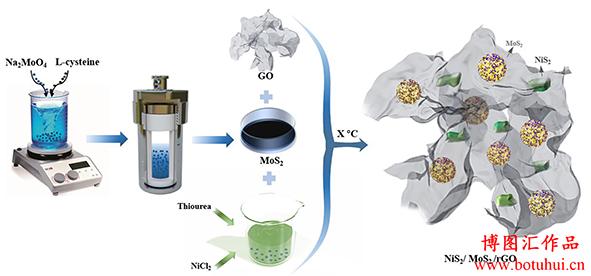
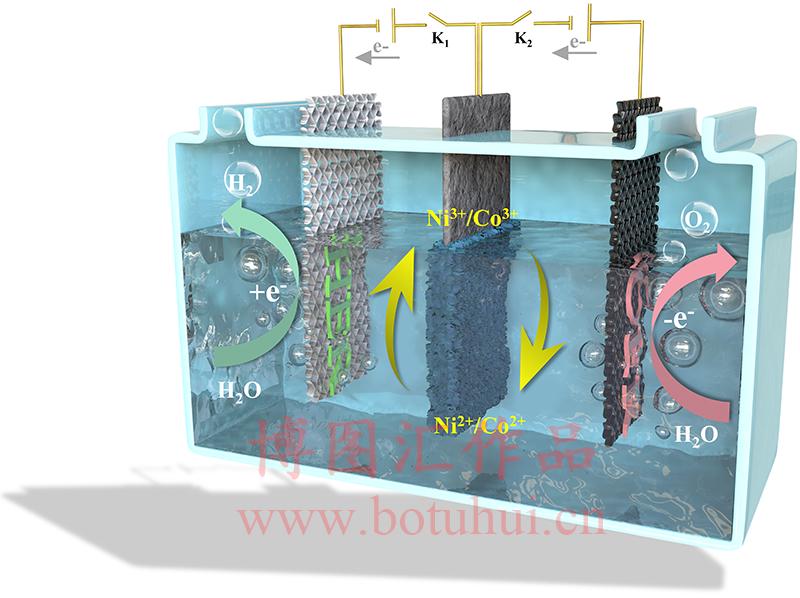
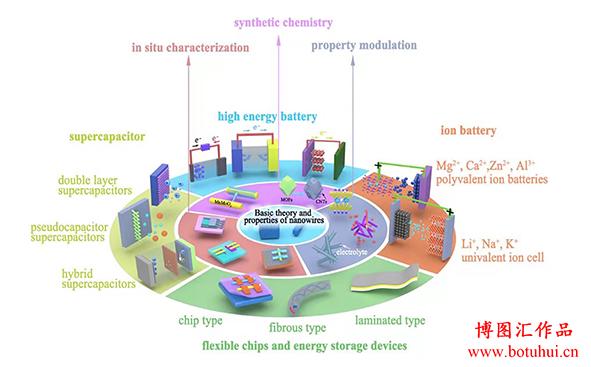
科研论文期刊封面图、摘要图、图文摘要(Graphical Abstract)、TOC图(Table of Contents)、插图、配图、原理图、示意图、机制图、数据图等的设计和绘制,将科研学者的idea、概念、原理等以图表的形式展现出来,将艺术审美与严谨的科研相结合。
本文来自互联网用户投稿,该文观点仅代表作者本人,不代表本站立场。本站仅提供信息存储空间服务,不拥有所有权,不承担相关法律责任。如若转载,请注明出处:http://www.rhkb.cn/news/32633.html
如若内容造成侵权/违法违规/事实不符,请联系长河编程网进行投诉反馈email:809451989@qq.com,一经查实,立即删除!