硬件设计:
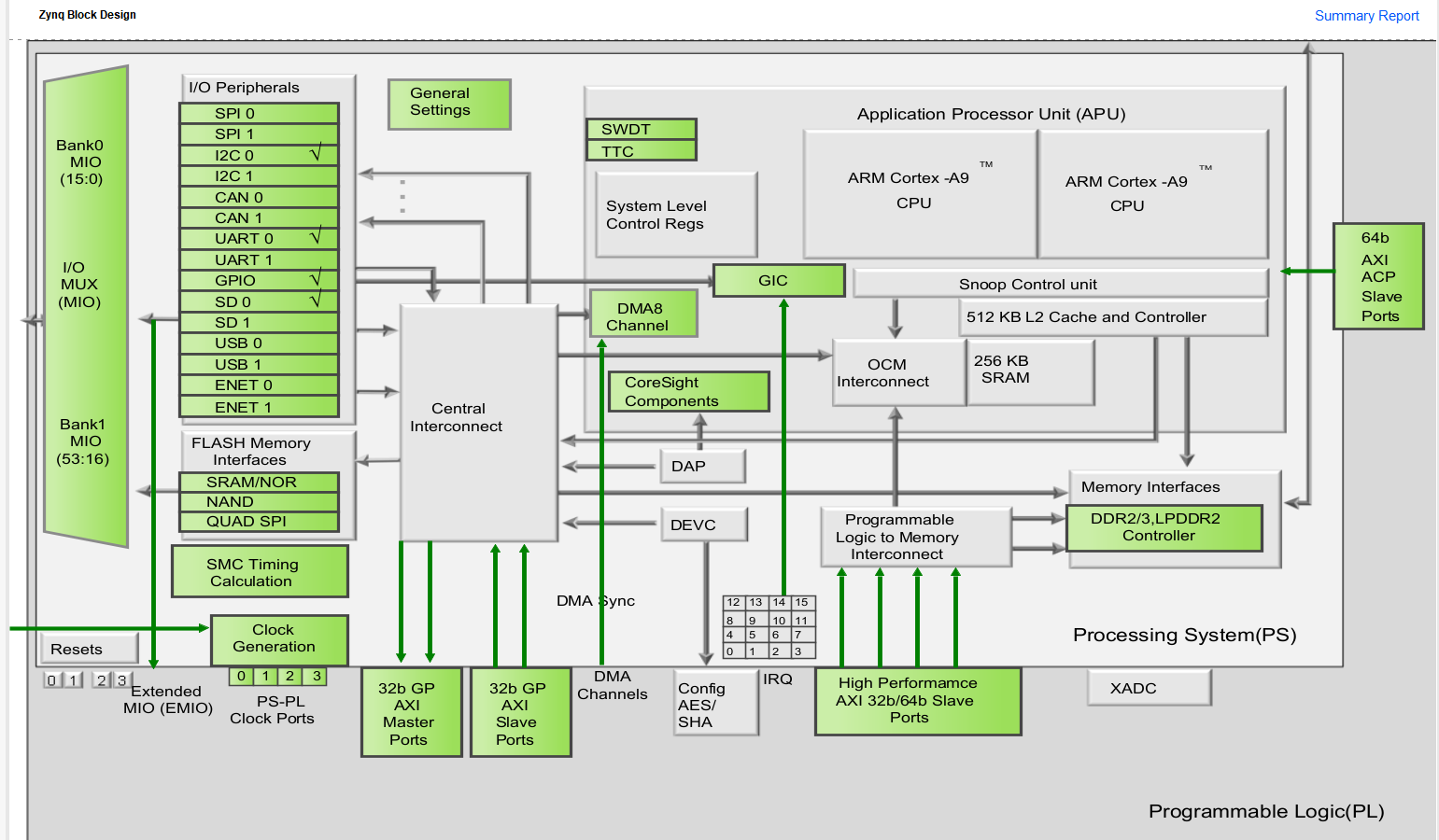
软件设计:
/******************************************************************************
*
* Copyright (C) 2009 - 2014 Xilinx, Inc. All rights reserved.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* Use of the Software is limited solely to applications:
* (a) running on a Xilinx device, or
* (b) that interact with a Xilinx device through a bus or interconnect.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
* XILINX BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
* WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF
* OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*
* Except as contained in this notice, the name of the Xilinx shall not be used
* in advertising or otherwise to promote the sale, use or other dealings in
* this Software without prior written authorization from Xilinx.
*
******************************************************************************//** helloworld.c: simple test application** This application configures UART 16550 to baud rate 9600.* PS7 UART (Zynq) is not initialized by this application, since* bootrom/bsp configures it to baud rate 115200** ------------------------------------------------* | UART TYPE BAUD RATE |* ------------------------------------------------* uartns550 9600* uartlite Configurable only in HW design* ps7_uart 115200 (configured by bootrom/bsp)*/#include <stdio.h>
#include "platform.h"
#include "xil_printf.h"
#include "xparameters.h"
#include "xgpiops.h"
#include "xscugic.h"
#include "xiicps.h"//IIC
#define IIC_DEVICE_ID XPAR_PS7_I2C_0_DEVICE_ID
#define IIC_RATE 100000
#define IIC_SLV_ADDR 0x40
//GPIO
#define GPIO_DEVICE_ID XPAR_XGPIOPS_0_DEVICE_ID
#define INTC_DEVICE_ID XPAR_SCUGIC_0_DEVICE_ID
#define GPIO_BANK_2 XGPIOPS_BANK2
#define Interrupt_ID_Gpio 52
#define XIL_EXCEPTION_ID_INT XIL_EXCEPTION_ID_IRQ_INT#define LED0 54
#define LED1 55
#define Bottom 56
//T&H
char CMD_GET_Humid = 0xE5;
char CMD_GET_Temp =0xE3;
char Hdata[2];
char Tdata[2];
double Tout,Hout;
double avgdata[4];
//Iic
static XIicPs IIC_point_instance;
static XIicPs_Config * IIC_ConFig;
///Gpio
static XGpioPs GPIO_Decive;
static XGpioPs_Config *GPIO_Device_ConFig;
//Gpio gic
static XScuGic Interrupt_Gpio_instance;
static XScuGic_Config *Interrupt_Gpio_ConFig;
//LED
static int LED_value = 0;//initial iic
int initIicPs();
double avgfilter(double * srcDataPtr,double inData,int count);int GPIO_init_TEST(void);
void GPIO_GIC_INIT();
static void IntrHandler(void *CallBackRef, u32 Bank, u32 Status);int main()
{int status;init_platform();status = initIicPs();avgdata[0]=0;avgdata[1]=0;avgdata[2]=0;avgdata[3]=0;if(status != XST_SUCCESS){return XST_FAILURE;}GPIO_init_TEST();print("init done");GPIO_GIC_INIT();while (1){}cleanup_platform();return 0;
}int GPIO_init_TEST(void)
{int Status;GPIO_Device_ConFig = XGpioPs_LookupConfig(GPIO_DEVICE_ID);if (GPIO_Device_ConFig == NULL) {return XST_FAILURE;}Status = XGpioPs_CfgInitialize(&GPIO_Decive,GPIO_Device_ConFig,GPIO_Device_ConFig->BaseAddr);if (Status != XST_SUCCESS) {return XST_FAILURE;}//LED0XGpioPs_SetDirectionPin(&GPIO_Decive,LED0,0x01);XGpioPs_SetOutputEnablePin(&GPIO_Decive,LED0,0x01);//LED1XGpioPs_SetDirectionPin(&GPIO_Decive,LED1,0x01);XGpioPs_SetOutputEnablePin(&GPIO_Decive,LED1,0x01);//BottomXGpioPs_SetDirectionPin(&GPIO_Decive,Bottom,0x0);XGpioPs_SetOutputEnablePin(&GPIO_Decive,Bottom,0x01);//initXGpioPs_WritePin(&GPIO_Decive, LED0, 0x00);XGpioPs_WritePin(&GPIO_Decive, LED1, 0x00);
}void GPIO_GIC_INIT(){int Status;Xil_ExceptionInit();Interrupt_Gpio_ConFig = XScuGic_LookupConfig(GPIO_DEVICE_ID);if (NULL == Interrupt_Gpio_ConFig) {return XST_FAILURE;}Status = XScuGic_CfgInitialize(&Interrupt_Gpio_instance,Interrupt_Gpio_ConFig,Interrupt_Gpio_ConFig->CpuBaseAddress);if (Status != XST_SUCCESS) {return XST_FAILURE;}Xil_ExceptionRegisterHandler(XIL_EXCEPTION_ID_INT,(Xil_ExceptionHandler) XScuGic_InterruptHandler,&Interrupt_Gpio_instance);Status = XScuGic_Connect(&Interrupt_Gpio_instance,Interrupt_ID_Gpio,(Xil_InterruptHandler )XGpioPs_IntrHandler,(void *)&GPIO_Decive);if (Status != XST_SUCCESS) {return XST_FAILURE;}XGpioPs_SetIntrType(&GPIO_Decive,GPIO_BANK_2,0xffffffff,0x00,0x00) ;XGpioPs_SetCallbackHandler(&GPIO_Decive,(void *) &GPIO_Decive,(XGpioPs_Handler )IntrHandler);XGpioPs_IntrEnable(&GPIO_Decive,GPIO_BANK_2,(1<<(Bottom-54)));XScuGic_Enable(&Interrupt_Gpio_instance,Interrupt_ID_Gpio);Xil_ExceptionEnableMask(XIL_EXCEPTION_IRQ);return XST_SUCCESS;
}static void IntrHandler(void *CallBackRef, u32 Bank, u32 Status)
{XGpioPs *Gpio = (XGpioPs *)CallBackRef;u32 DataRead;int status;unsigned short tmp =0;/* Push the switch button */DataRead = XGpioPs_ReadPin(Gpio, Bottom);if (DataRead == 0) {status = XIicPs_MasterSendPolled(&IIC_point_instance,&CMD_GET_Temp,0x01,IIC_SLV_ADDR);if(status != XST_SUCCESS){return XST_FAILURE;}status = XIicPs_MasterRecvPolled(&IIC_point_instance,Tdata,0x02,IIC_SLV_ADDR);//read hum valuestatus = XIicPs_MasterSendPolled(&IIC_point_instance,&CMD_GET_Humid,0x01,IIC_SLV_ADDR);if(status != XST_SUCCESS){return XST_FAILURE;}status = XIicPs_MasterRecvPolled(&IIC_point_instance,Hdata,0x02,IIC_SLV_ADDR);tmp = (Tdata[0]<<8) | (Tdata[1]&0xfc);Tout = (175.72*tmp/65536)-46.85;tmp =(Hdata[0]<<8) | (Hdata[1]&0xfc);Hout = ((125*tmp)/65536) - 6;Tout = avgfilter(avgdata,Tout,0x04);printf("Humidity = %.1f; temperature = %.1f \n",Hout,Tout);}
}int initIicPs(){int status;IIC_ConFig = XIicPs_LookupConfig(IIC_DEVICE_ID);status = XIicPs_CfgInitialize(&IIC_point_instance,IIC_ConFig,IIC_ConFig->BaseAddress);if(status != XST_SUCCESS){return XST_FAILURE;}//set iic ratestatus = XIicPs_SetSClk(&IIC_point_instance,IIC_RATE);if(status != XST_SUCCESS){return XST_FAILURE;}return XST_SUCCESS;
}
double avgfilter(double * srcDataPtr,double inData,int count){int i;double outdata;for(i=0;i<count;i++){if(i <(count-1)){srcDataPtr[i+1]=srcDataPtr[i];}}srcDataPtr[0] =inData;outdata = (srcDataPtr[0] + srcDataPtr[1] + srcDataPtr[2] +srcDataPtr[3])/4;return outdata;
}