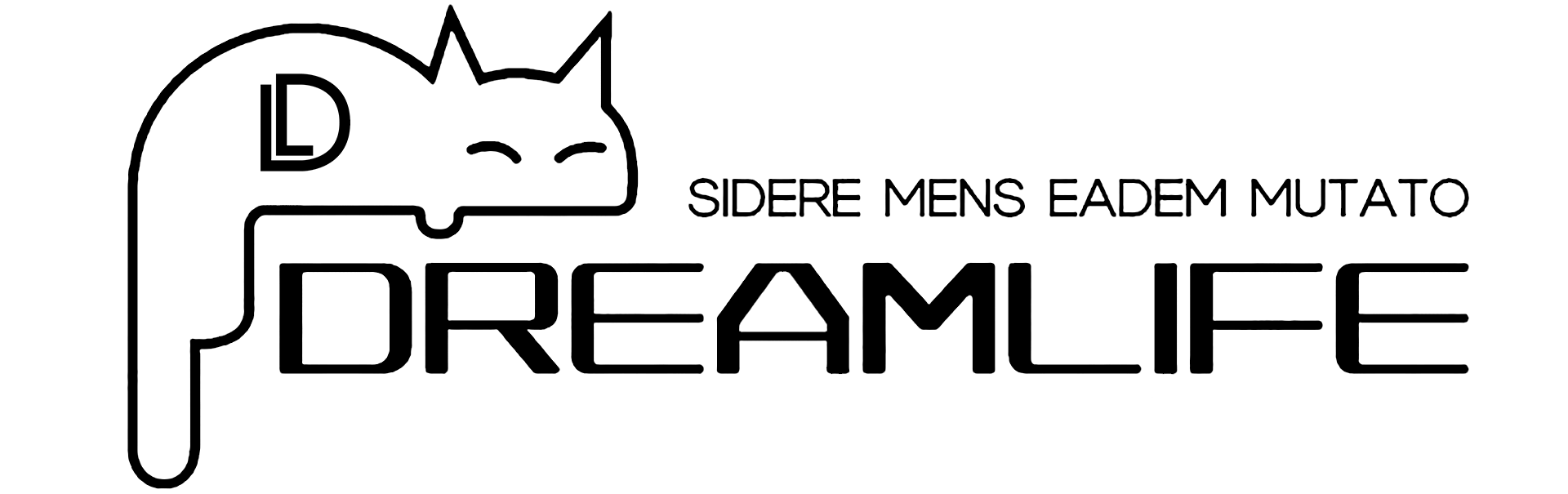
第三代软件开发-文件Model
文章目录
- 第三代软件开发-文件Model
- 项目介绍
- 文件Model
关键字:
Qt
、
Qml
、
关键字3
、
关键字4
、
关键字5
项目介绍
欢迎来到我们的 QML & C++ 项目!这个项目结合了 QML(Qt Meta-Object Language)和 C++ 的强大功能,旨在开发出色的用户界面和高性能的后端逻辑。
在项目中,我们利用 QML 的声明式语法和可视化设计能力创建出现代化的用户界面。通过直观的编码和可重用的组件,我们能够迅速开发出丰富多样的界面效果和动画效果。同时,我们利用 QML 强大的集成能力,轻松将 C++ 的底层逻辑和数据模型集成到前端界面中。
在后端方面,我们使用 C++ 编写高性能的算法、数据处理和计算逻辑。C++ 是一种强大的编程语言,能够提供卓越的性能和可扩展性。我们的团队致力于优化代码,减少资源消耗,以确保我们的项目在各种平台和设备上都能够高效运行。
无论您是对 QML 和 C++ 开发感兴趣,还是需要我们为您构建复杂的用户界面和后端逻辑,我们都随时准备为您提供支持。请随时联系我们,让我们一同打造现代化、高性能的 QML & C++ 项目!
重要说明☝
☀该专栏在第三代软开发更新完将涨价
文件Model
这个就是想做一个通用一点的model,因为我需要知道我的文件夹下的mp4 和pdf 文件,这两个除了类型不同,其他功能都相同,所以就有了这个,代码如下
头文件
#ifndef XXXX_FILEMODEL_H
#define XXXX_FILEMODEL_H#include <QAbstractListModel>class XXXX_FileModel : public QAbstractListModel
{Q_OBJECTenum FileType{Name,};Q_PROPERTY(QString path READ path WRITE setPath NOTIFY pathChanged FINAL)public:explicit XXXX_FileModel(QObject *parent = nullptr);// Header:QVariant headerData(int section, Qt::Orientation orientation, int role = Qt::DisplayRole) const override;bool setHeaderData(int section, Qt::Orientation orientation, const QVariant &value, int role = Qt::EditRole) override;// Basic functionality:int rowCount(const QModelIndex &parent = QModelIndex()) const override;// Fetch data dynamically:bool hasChildren(const QModelIndex &parent = QModelIndex()) const override;bool canFetchMore(const QModelIndex &parent) const override;void fetchMore(const QModelIndex &parent) override;QVariant data(const QModelIndex &index, int role = Qt::DisplayRole) const override;QHash<int,QByteArray> roleNames() const override;// Editable:bool setData(const QModelIndex &index, const QVariant &value,int role = Qt::EditRole) override;Qt::ItemFlags flags(const QModelIndex& index) const override;// Add data:bool insertRows(int row, int count, const QModelIndex &parent = QModelIndex()) override;// Remove data:bool removeRows(int row, int count, const QModelIndex &parent = QModelIndex()) override;QString path() const;void setPath(const QString &newPath);signals:void pathChanged();protected:void updateFile();private:QString m_path = "/home";QStringList m_fileList;
};#endif // XXXX_FILEMODEL_H
源文件
#include "XXXX_filemodel.h"
#include "qdebug.h"
#include "qdir.h"XXXX_FileModel::XXXX_FileModel(QObject *parent): QAbstractListModel(parent)
{updateFile();
}QVariant XXXX_FileModel::headerData(int section, Qt::Orientation orientation, int role) const
{// FIXME: Implement me!
}bool XXXX_FileModel::setHeaderData(int section, Qt::Orientation orientation, const QVariant &value, int role)
{if (value != headerData(section, orientation, role)) {// FIXME: Implement me!emit headerDataChanged(orientation, section, section);return true;}return false;
}int XXXX_FileModel::rowCount(const QModelIndex &parent) const
{// For list models only the root node (an invalid parent) should return the list's size. For all// other (valid) parents, rowCount() should return 0 so that it does not become a tree model.if (parent.isValid())return 0;// FIXME: Implement me!return m_fileList.count();
}bool XXXX_FileModel::hasChildren(const QModelIndex &parent) const
{// FIXME: Implement me!
}bool XXXX_FileModel::canFetchMore(const QModelIndex &parent) const
{// FIXME: Implement me!return false;
}void XXXX_FileModel::fetchMore(const QModelIndex &parent)
{// FIXME: Implement me!
}QVariant XXXX_FileModel::data(const QModelIndex &index, int role) const
{if (!index.isValid())return QVariant();// FIXME: Implement me!if(m_fileList.count() > 0)return m_fileList.at(index.row());return QVariant();
}QHash<int, QByteArray> XXXX_FileModel::roleNames() const
{QHash<int,QByteArray> roles;roles.insert(FileType::Name,"name");return roles;
}bool XXXX_FileModel::setData(const QModelIndex &index, const QVariant &value, int role)
{if (data(index, role) != value) {// FIXME: Implement me!emit dataChanged(index, index, {role});return true;}return false;
}Qt::ItemFlags XXXX_FileModel::flags(const QModelIndex &index) const
{if (!index.isValid())return Qt::NoItemFlags;return QAbstractItemModel::flags(index) | Qt::ItemIsEditable; // FIXME: Implement me!
}bool XXXX_FileModel::insertRows(int row, int count, const QModelIndex &parent)
{beginInsertRows(parent, row, row + count - 1);// FIXME: Implement me!endInsertRows();return true;
}bool XXXX_FileModel::removeRows(int row, int count, const QModelIndex &parent)
{beginRemoveRows(parent, row, row + count - 1);// FIXME: Implement me!endRemoveRows();return true;
}QString XXXX_FileModel::path() const
{return m_path;
}void XXXX_FileModel::setPath(const QString &newPath)
{if (m_path == newPath)return;m_path = newPath;emit pathChanged();updateFile();
}void XXXX_FileModel::updateFile()
{QDir folder(m_path);m_fileList.clear();m_fileList = folder.entryList(QDir::Files);foreach(QString file, m_fileList){qDebug() << file;}
}
