在本教程中,我们利用 OpenAI 的语言模型 ChatGPT 的强大功能为鼠标移动机器人生成 Python 代码。
代码应每 2 秒将鼠标指针移动到屏幕上的随机位置。如何在指定时间自动打开计算机凭借基于自然语言输入生成代码的能力,ChatGPT 为希望快速实现某些功能而无需从头编写代码的开发人员提供了一种方便高效的解决方案。
让我们通过几个简单的步骤看看它是如何工作的:
通过 https://chat.openai.com/chat 登录 ChatGPT 并输入以下内容:
“生成 Python 代码以每 2 秒将鼠标移动到屏幕上的随机位置”
在下面的屏幕截图中,您可以看到 ChatGPT 正在为您提供能够满足要求的完整 Python 脚本作为答案:
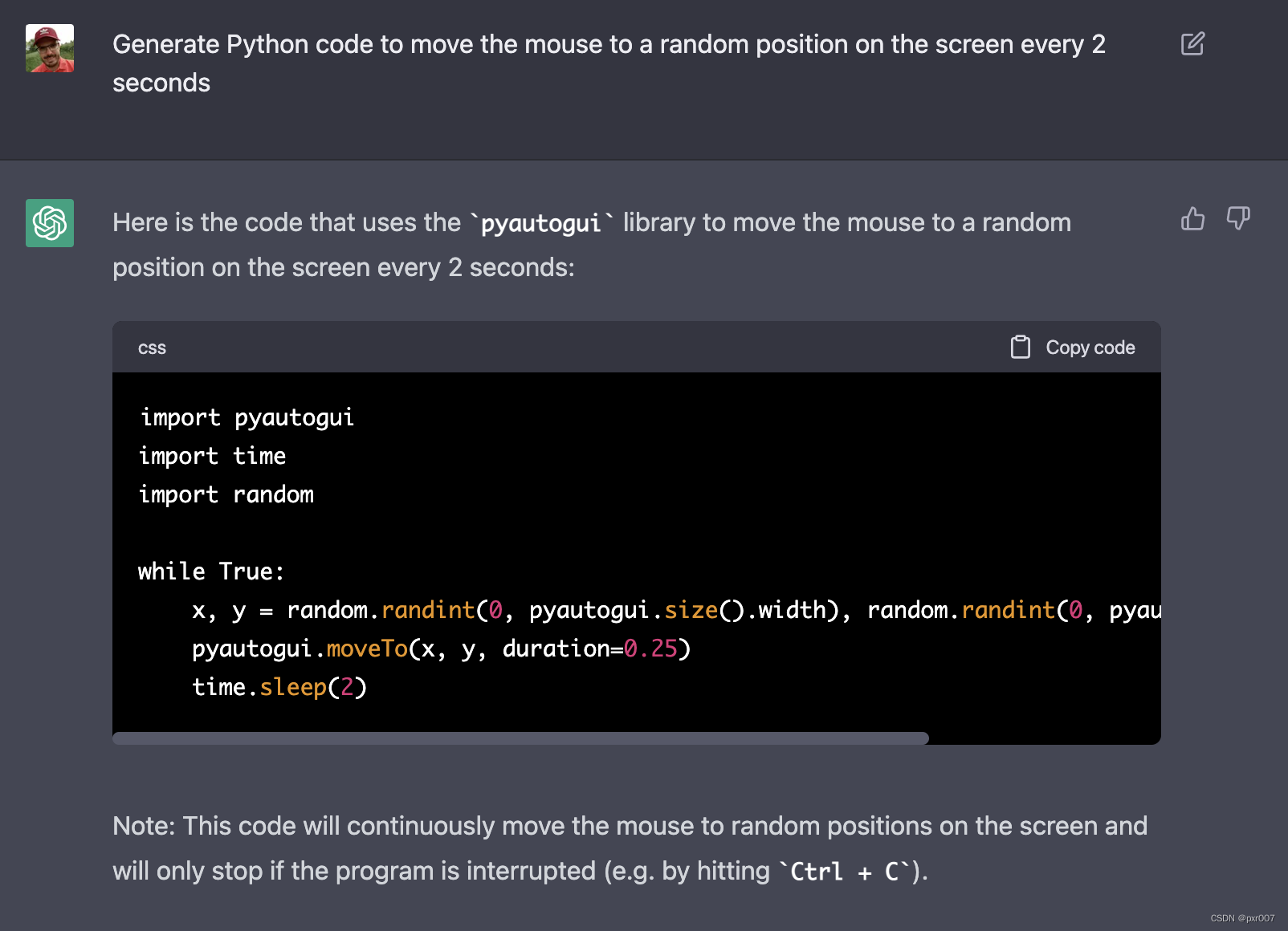
同样在以下清单中,您可以看到生成的 Python 代码:
import pyautogui
import time
import randomwhile True:x = random.randint(0, pyautogui.size().width)y = random.randint(0, pyautogui.size().height)pyautogui.moveTo(x, y, duration=0.25)time.sleep(2)
该代码使用 pyautogui 库自动执行鼠标移动,使用时间库将程序暂停特定时间,使用随机库生成随机数。
代码以一个无限循环开始,该循环生成代表鼠标指针的水平和垂直位置的随机 x 和 y 坐标。pyautogui.size().width和pyautogui.size().height函数用于获取屏幕的宽高,random.randint()函数用于生成屏幕边界内的随机x和y值.
然后使用生成的 x 和 y 坐标调用 pyautogui.moveTo() 函数,将鼠标指针移动到该位置。duration 参数设置为 0.25,这意味着鼠标移动到新位置需要 0.25 秒。
移动鼠标后,调用time.sleep()函数,值为2秒,让程序暂停2秒,然后重复循环。
这段代码会不断地将鼠标移动到屏幕上的随机位置,并且只有在程序被中断时才会停止(例如,通过按 Ctrl + C)。
为了执行代码,首先创建一个新的项目目录,并在该目录中创建一个新的空 Python 文件 mousemouse.py:
$ mkdir py-mouse
$ cd py-mouse
$ touch mousemove.py
将生成的代码复制并粘贴到该文件中,然后返回到命令行,并确保通过以下方式使用 pip 命令安装了 pyautogui 包:
$ pip install pyautogui
最后启动脚本:
$ python mousemove.py
您应该会看到鼠标指针每两秒移动到屏幕上的随机位置。
请记住: 此代码会连续将鼠标移动到屏幕上的随机位置,并且只有在程序被中断时才会停止(例如,通过按 Ctrl + C)。
结论
让 ChatGPT 为鼠标移动机器人生成 Python 代码非常容易。您需要做的就是让 ChatGPT 为您想要的任务编写代码,它会为您提供简洁且编写良好的代码片段。在这种情况下,它生成了一个完整的 Python 代码,该代码实现了一个鼠标移动机器人,每 2 秒将鼠标指针移动到屏幕上的随机位置。
ChatGPT 基于自然语言输入生成代码的能力使其成为希望快速实现某些功能而无需从头编写代码的开发人员的极其有用的工具。这可以节省大量时间和精力,尤其是对于像这样的简单任务。